Introduction
In this article I am going to make a XML Web
service that inserts data into a Default table of the application. For the
basics of XML Web Services read my last article. Click Here
First go through SQL Server and make a table;
The following snapshot shows the process.
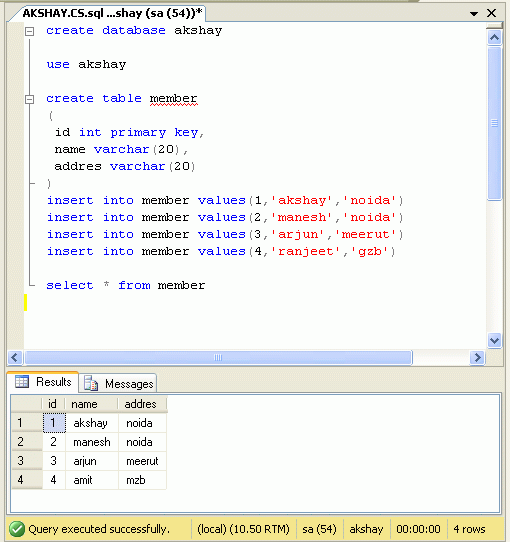
Database- akshay
Table Name-member
Creating XML Web Service in .Net
Here is sample code which I use to create and
consume ASP.NET
Web Service:
Step 1 : Create the ASP.NET Web Service Source
File
Open Visual Studio 2010 and create a new web
site.->Select .Net Framework 3.5. ->Select ASP.NET Web Service page -> Then, you
have to give the name of your service. In this example I am giving it the name "mywebservice1".
Then Click the ok Button. A screen-shot of this activity is shown below:
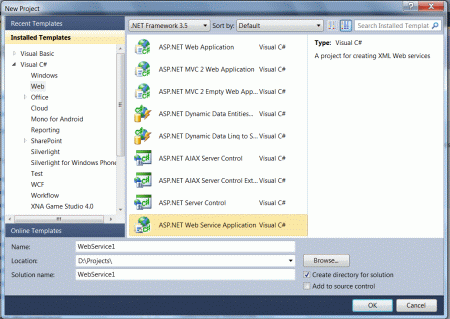
Step 2 : Click on the "OK" Button. You
will see the following window:
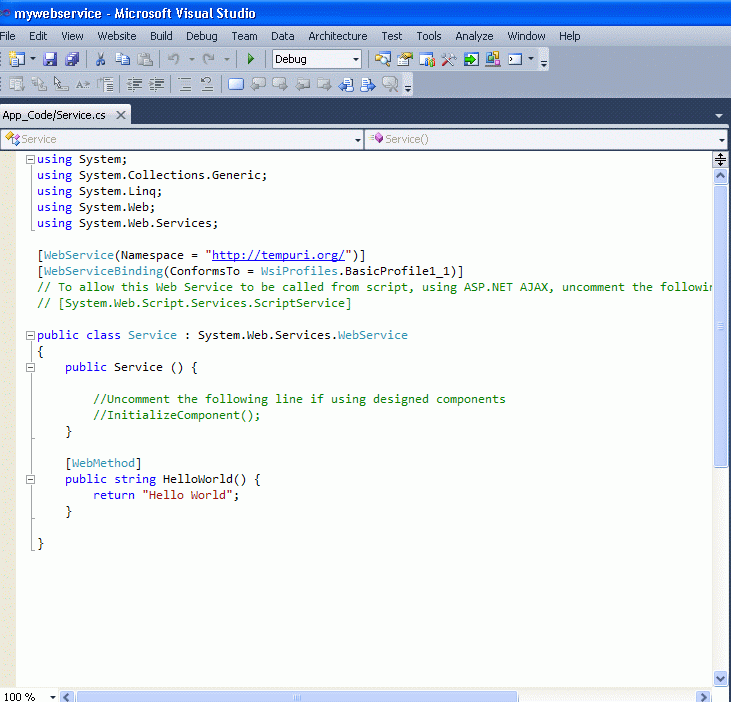
Here (in the above figure), you will note that
there is a predefined method "HelloWorld" which returns the string "Hello
World". You can use your own method and can perform other operations. Here I
made the simple method "SaveData" which returns a integer value.
Service.cs
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.Web.Services;
using
System.Data;
using
System.Data.SqlClient;
namespace
MyWebService1
{
///
<summary>
/// Summary
description for Service1
///
</summary>
[WebService(Namespace =
"http://tempuri.org/")]
[WebServiceBinding(ConformsTo =
WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from
script, using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class
Service1 : System.Web.Services.WebService
{
SqlConnection con;
SqlCommand cmd;
[WebMethod]
public int
SaveData(int pid,
string pna, string ad)
{
con = new
SqlConnection(@"Data
Source=.;Initial Catalog=akshay;Persist Security Info=True;User
ID=sa;pwd=wintellect");
con.Open();
cmd = new
SqlCommand("insert
into member values(" + pid + ",'" +
pna + "','" + ad +
"')", con);
int temp = cmd.ExecuteNonQuery();
return temp;
}
}
}
Step 3 : Build Web Service and Run the Web Service for
testing by pressing F5 function key.
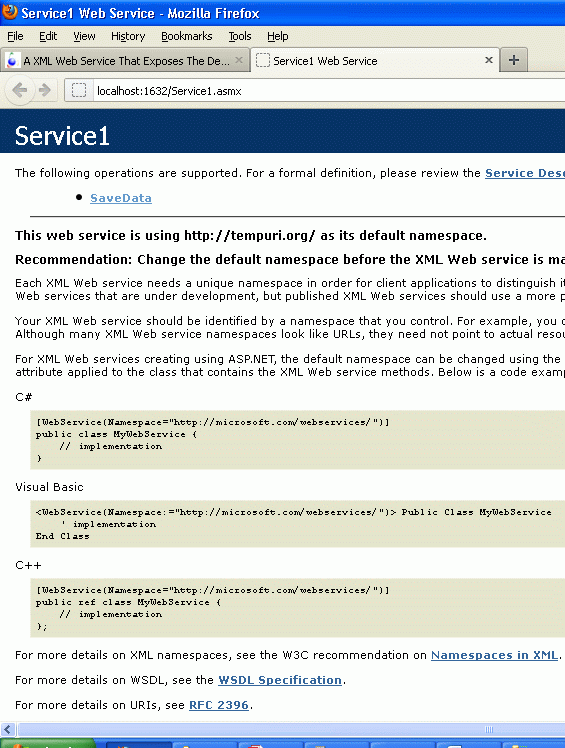
Copy the url of this web service for further
use.
Step 5 : Click on SaveData Button to test the web service.
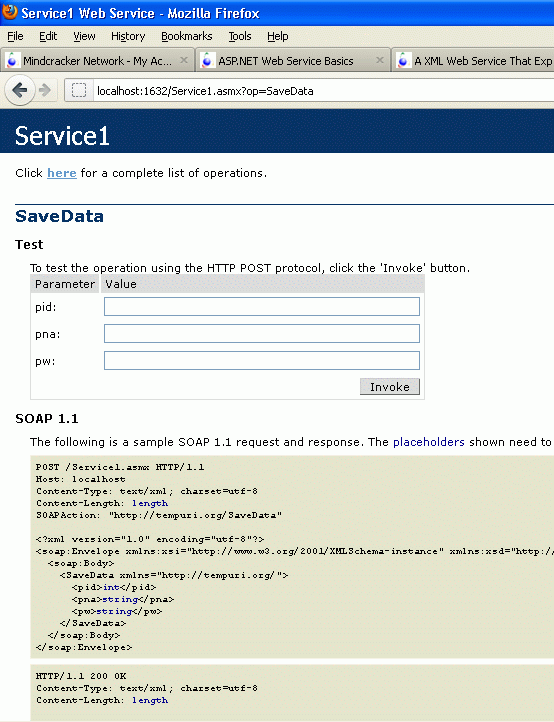
Enter the value of pid, pna and pw to test the web service
where [pid=id,pna=name and pw=addres ] is in the database table.
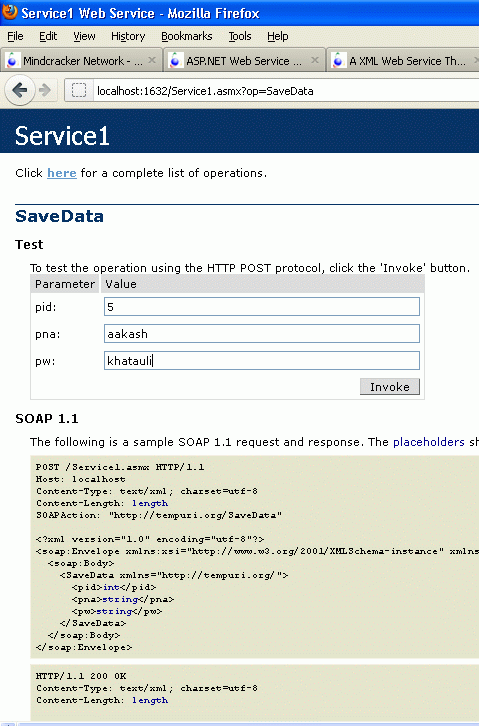
By pressing the "Invoke" button a XML file is
generated.
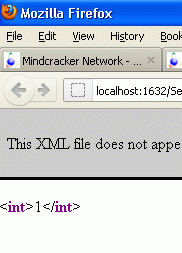
The '1' in the response to our data is inserted in specific
data base table (here "member") see here.
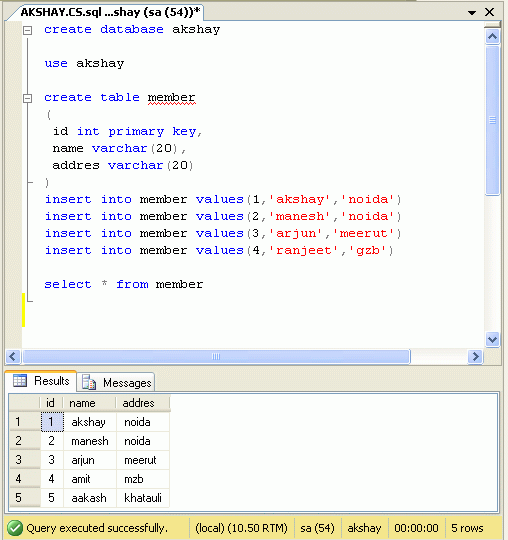
Now our web service is ready to use. We just need
to create a new web site to consume the web service.
Example of Testing Web Service in .Net
Step 6 : Create a Test Web Site by
File > New > Web Site > ASP.Net Web Site.
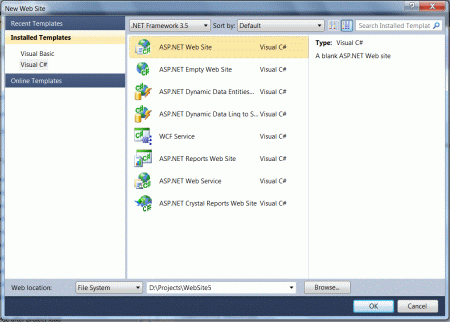
Named the web site, for example here I have chosen
name "MyTest" and click on 'ok' button.
Step 7 : Right Click Solution Explorer and Choose "Add Web Reference":
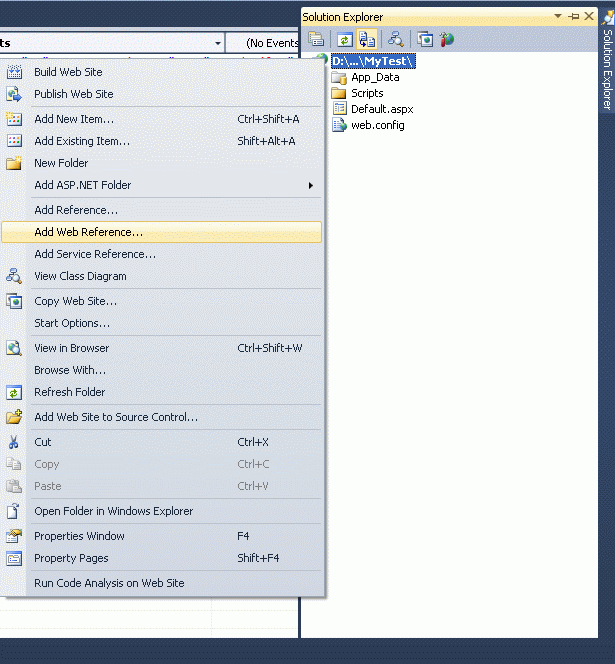
Step 8 : Past the url of the web service and click on "Green arrow" button and then "Add
reference".
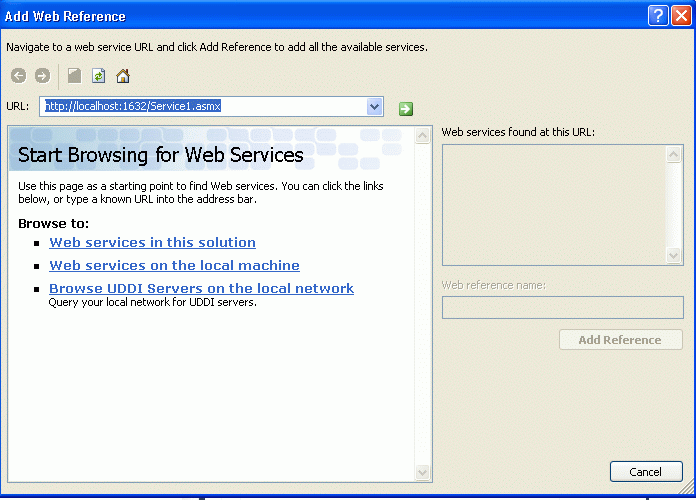
Step 9 : Now your web service is ready to
use; in the Solution Explorer you will see:
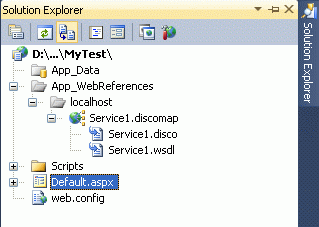
Step 10 : Go to the design of the
Default.aspx page; drag and drop three TextBoxes, one button and a Lable.
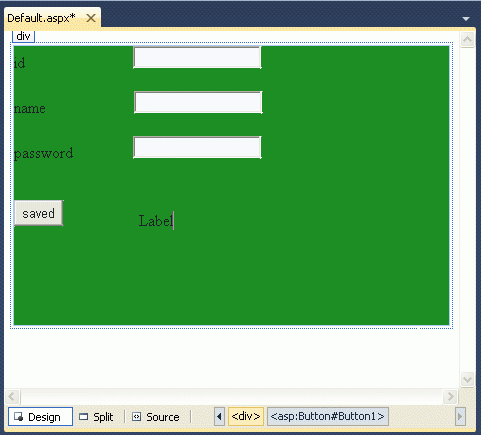
Rename the Button as 'saved'.
step 11 : Go to Default.cs page and on the
button click event use the following code.
protected void
Button1_Click(object sender,
EventArgs e)
{
int id =
Convert.ToInt32(TextBox1.Text);
string na = TextBox2.Text;
string ad = TextBox3.Text;
localhost.Service1 myservice =
new localhost.Service1();
// you need to create the object of the web
service
int roweffected=myservice.SaveData(id,na,ad);
if (roweffected == 1)
{
Label1.Text = "Record is saved";
}
else
{
Label1.Text = "sorry record is not saved
try again";
}
}
}
Step 12 : Pressing the F5 function key to run the website, you will see:
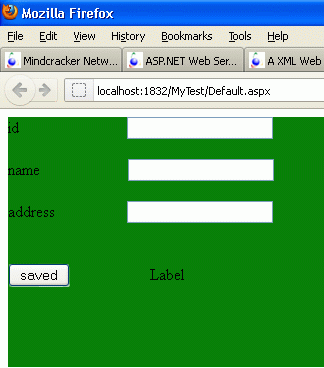
Enter the value of textbox
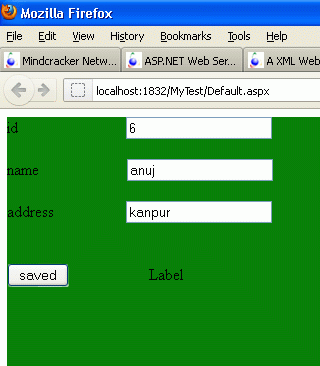
Press the saved button.
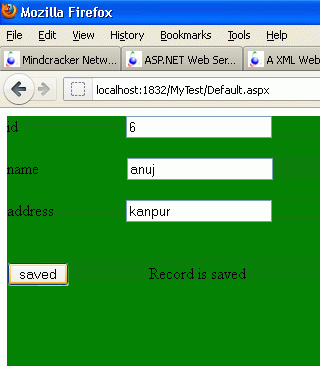
The record is saved; you can check it from your database.
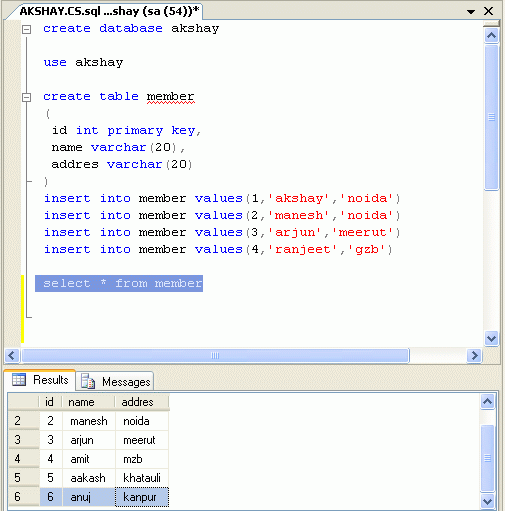
Resources