A ViewBox control is used to stretch and scale a single
child item to fill the available space.
Creating a ViewBox
The Viewbox element
represents a WPF ViewBox control in XAML.
<Viewbox />
Viewbox has a Stretch
property that defines how contents are fit in the space and its value can be –
Fill, None, Uniform, or UniformToFill.
The code snippet in
Listing 1 creates a Viewbox control and sets its stretch to fill. The output
looks like Figure 1 where child control is filled in the Viewbox area.
<Viewbox Height="200" HorizontalAlignment="Left" Margin="19,45,0,0"
Name="viewbox1" VerticalAlignment="Top" Width="300"
Stretch="Fill">
<Ellipse Width="100" Height="100" Fill="Red" />
</Viewbox>
Listing 1
The output looks like Figure 1.
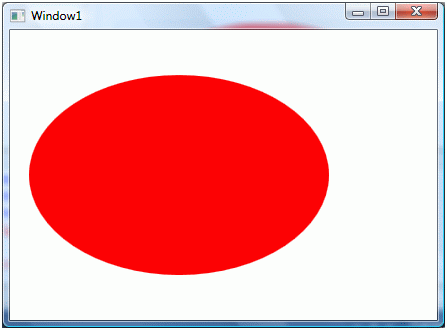
Figure 1
Creating a ViewBox Dynamically
The following code
snippet creates a Viewbox at run-time.
private void CreateViewboxDynamically()
{
Viewbox
dynamicViewbox = new Viewbox();
dynamicViewbox.StretchDirection = StretchDirection.Both;
dynamicViewbox.Stretch = Stretch.Fill;
dynamicViewbox.MaxWidth = 300;
dynamicViewbox.MaxHeight = 200;
Ellipse
redCircle = new Ellipse();
redCircle.Height = 100;
redCircle.Width = 100;
redCircle.Fill = new
SolidColorBrush(Colors.Red);
dynamicViewbox.Child = redCircle;
RootLayout.Children.Add(dynamicViewbox);
}