Step 7: Now at the code behind write the code as given Below.
using System;
using System.Web;
using System.Collections.Generic;
using System.Linq;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.Adapters;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
using System.Text;
using System.Text.RegularExpressions;
using System.IO;
public partial class SearchNSortGrid : System.Web.UI.Page
{
string SearchString = "";
public string connection;
protected void Page_Load(object sender, EventArgs e)
{
connection = System.Configuration.ConfigurationManager.ConnectionStrings["Connectionstring"].ToString();
txtSearch.Attributes.Add("onkeyup", "setTimeout('__doPostBack(\'" + txtSearch.ClientID.Replace("_", "$") + "\',\'\')', 0);");
if (!IsPostBack)
{
Gridview2.DataBind();
}
}
protected void txtSearch_TextChanged(object sender, EventArgs e)
{
SearchString = txtSearch.Text;
}
public string HighlightText(string InputTxt)
{
string Search_Str = txtSearch.Text.ToString(); // Setup the regular expression and add the Or operator.
Regex RegExp = new Regex(Search_Str.Replace(" ", "|").Trim(), RegexOptions.IgnoreCase); // Highlight keywords by calling the //delegate each time a keyword is found.
return RegExp.Replace(InputTxt, new MatchEvaluator(ReplaceKeyWords)); // Set the RegExp to null.
RegExp = null;
}
public string ReplaceKeyWords(Match m)
{
return "<span class=highlight>" + m.Value + "</span>";
}
}
Step 8: Run application (Press F5).
Summary
The Final Layouts Will be as given Below.
Figure: 1.1: On Load At first time.
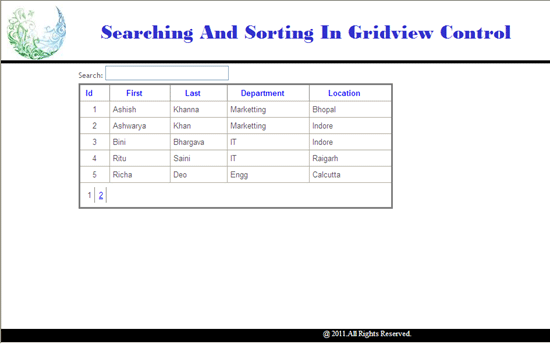
Figure 1.2: On Searching with initials 'As'
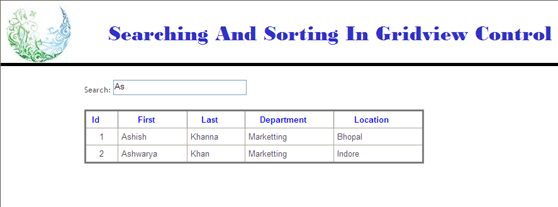
Figure 1.3: On Sorting with Department Column Field in ASC Order
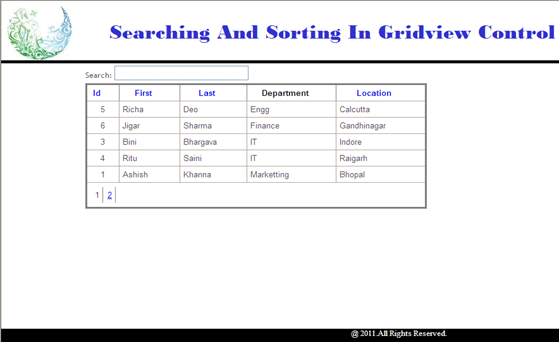
Figure 1.4: On Paging in Grid view.
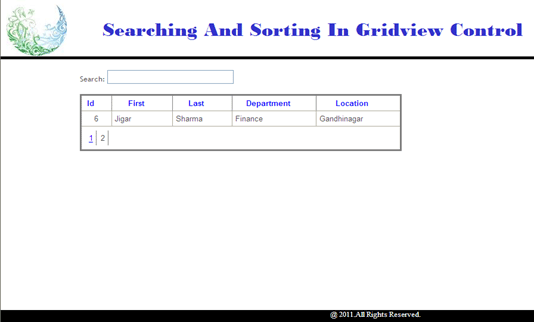