Overview
Auto completing is one way to offer a productive and programmer friendly user interface to programmers and Intellisense feature of Visual Studio .NET is one of them. This example shows you an example of how to implement a feature like that.
In fact when we press comma, the editor gets a drop-down list of words we can use. This example is built with a MS SQL Server 2000, using ADO.NET
Description
First of all you have to copy the Intellisense.mdf and Intellisense.log in your database folder.
In this example, I used a textbox and listbox object. The first thing is to have a connection to the database. In this case I used MS SQL Server 2000, using ADO.NET. obviously the choice of what kind of data provider to use is extremely easy: SQLProvider. So the first step is to declare the SQLConnection and the SQLCommand. Then you need to set them: in this case I did it in the InizializeComponent. Lets have a look at the code:
//
// conn
//
this.conn.ConnectionString = "data source=rohan;initial catalog=Intellisense;integrated security=SSPI;persist security" + " info=False;workstation id=" + Environment.MachineName + ";packet size=" + conn.PacketSize;
//
// cmd
//
this.cmd.Connection = this.conn;
the connectionstring is really important, dont forget to change datasource=rohan with datasource = YourServerMachine.
Now, have look at the GUI:
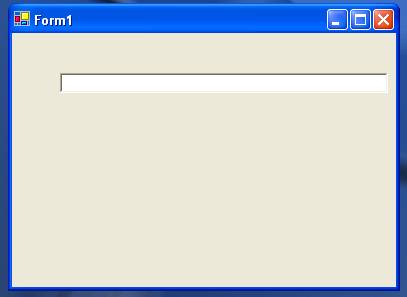
when you start typing:
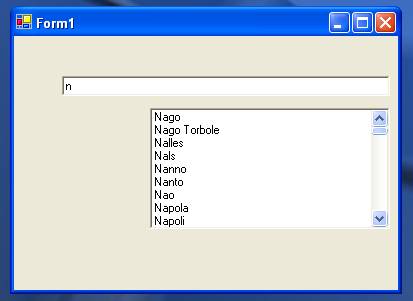
so, the code is all in the KeyPress event.
Source Code
private