Properties are accessor methods whose job it is to retrieve and set the values of fields. It provides a way to protect a field in a class by reading and writing and also support object-oriented concept of encapsulation. In other language user can use this feature using getter and setter methods. When user wants to wrap a value in attribute of an object or get the value from attribute of an object and when want to use attribute value. It is always useful to use setter method to wrap value in attribute and getter method to retrieve value from attribute of an object. Properties provide a level of abstraction to user.
Most of object-oriented programmer used getter and setter method. As in Java language user used accessor method as below.
Java Program
public class PersonAge
{
private int age; // private local variable
public void getAge() //get accessor method
{
return age;
}
public void setAge(int age) //set accessor method
{
myAge = age;
}
}
In main program user wants to use these set and get method to set and get person age. They will use it as
PersonAge boyAge = new PersonAge(); //create instance of object
boyAge.setAge(10); // set the age of boy by using setter method of object
Syetm.out.println("Boy age is "+boyAge.getAge()); // get the age of boy by using
// getter method of object
These setter and getter method was very useful in object-oriented world.
In .NET framework in C# these setter and getter method are presented under the heading properties. Now in .NET framework way of presentation of properties is very smoother and useful to the programmer.
Above code in C#
C# Code
public class PersonAge
{
private int age; // private local variable
public int humanAge
{
get //get accessor method
{
return age;
}
set //set accessor method
{
age = value;
}
}
}
So when in main program(C#) user wants to use these set and get method to set and get person age. They will use it as
PersonAge boyAge = new PersonAge(); //create instance of object
boyAge. humanAge = 10; // set the age of boy by using setter method of object
int bAge = boyAge. humanAge;// get the age of boy by
// using getter method of object
Console.WriteLine(" Boy age is {0}"+ bAge );
Class PersonAge is property holder class. In which humanAge is property implementation. I will try to explain about each line how it works.
value : In set method value variable is internal c# variable. User no needs to define it explicitly.
boyAge. humanAge = 10; calls set method and set the value for variable myAge as 10.
bAge = boyAge. humanAge ( last one in program) calls get method and get value 10;
Now you may notice that the setter doesn't take any arguments. The value being passed is automatically placed in a variable named value that is accessible inside the setter method.
Some one may be wonder that when they are not passing any data for set methods how it works internally.
I want also to show that how it internally works. I used my favorite ILDASM.exe (Intermediate Language Disassembler). (Read my article to know more about ILDAM)
Run ILDASM.exe XXXX.exe on command prompt
User will see output as below.
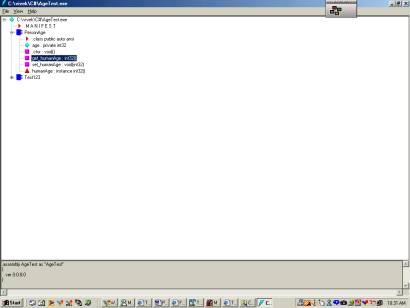
Two classes one main and other property class are displayed (by symbol
).
Click on first class user will see some two methods (by symbol
).
get_humaneAge: int32 and set_humanAge:void(int32)
You can see the name of the accessor methods because the compiler prefixes the property name with get_ (for a getter method) or set_ (for a setter method). So code
boyAge. humanAge = 10; is basically boyAge.set_humanAge(10). if you will click on set_ and get_ methods in ILDASM output you will get out put as
.method public hidebysig specialname instance int32
get_humanAge() il managed
{
// Code size 11 (0xb)
.maxstack 1
.locals (int32 V_0)
IL_0000: ldarg.0
IL_0001: ldfld int32 PersonAge::age
IL_0006: stloc.0
IL_0007: br.s IL_0009
IL_0009: ldloc.0
IL_000a: ret
} // end of method PersonAge::get_humanAge
And
.method public hidebysig specialname instance void
set_humanAge(int32 'value') il managed
{
// Code size 8 (0x8)
.maxstack 8
IL_0000: ldarg.0
IL_0001: ldarg.1
IL_0002: stfld int32 PersonAge::age
IL_0007: ret
} // end of method PersonAge::set_humanAge
I am not going to explain bit by bit of above code.
WARNING: Never try to use get_and set_method of ILDASM output in your code. Compiler will generate error.
If still you have some doubts about properties in your mind please mail me.
I do not write only because I want to write. I always try to present topics in easiest way so that beginner in C# could understand. -- Vivek