Objective
This article will discuss about static class in c#. Different feature of static class and different way of creating static class also will be discussed.
Finally one sample will give a hand on of the static class.
Static class is type of classes which cannot be instantiated. Instance of static class cannot be created.
10 Features of Static class
-
A static class cannot be instantiated
-
Static class can have only static members.
-
Member of the Static class can be accessed by class name itself.
-
Static class is sealed. So static class cannot be inherited.
-
Static class contains only static constructors.
-
Static class cannot have instance constructors.
-
Static class can only be inherited only from object class.
-
Static class is preceded by keyword static.
-
Static constructor of static class called only once.
-
Static class has private constructors.
How CLR deals with static class?
-
Information of Static class is loaded by CLR, when the Program accessing or referencing Static class is being loaded.
-
The program referencing static class has no control to specify when static class should be loaded by CLR.
-
Static class remains in memory for the lifetime of application domain in which program resides.
How to create Static class?
-
Create a class with keyword Static.
-
Create a private constructor inside class.
-
Make all members as static.
Creating a Static class
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Staticclass
{
public static class MyMath
{
public static int Add(int number1, int number2)
{
return number1 + number2;
}
public static int Sub(int number1, int number2)
{
return number1 - number2;
}
public static int Mul(int number1, int number2)
{
return number1 * number2;
}
public static int Div(int number1, int number2)
{
return number1 / number2;
}
}
}
-
Class is preceded with keyword Static.
-
Class is having 4 static methods.
-
Each method is also preceded by keyword Static.
Using Static class in Program
On adding of reference of Static class, Intellisense will show all the static methods of static class. MyMath is name of the static class.
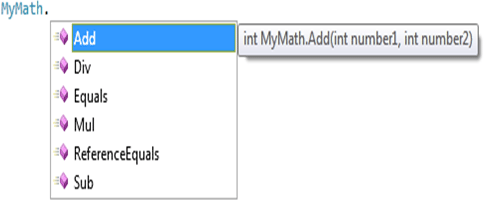
We do not need to create instance of the class , directly static methods could be called with class name.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Staticclass
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Sumation= "+ MyMath.Add(7, 2).ToString());
Console.WriteLine("Substraction = " + MyMath.Sub(7, 2).ToString());
int mul = MyMath.Mul(7,2);
Console.WriteLine("Multiply = " + mul );
Console.WriteLine("Divison = " + MyMath.Div(7, 2).ToString());
Console.Read();
}
}
}
Output
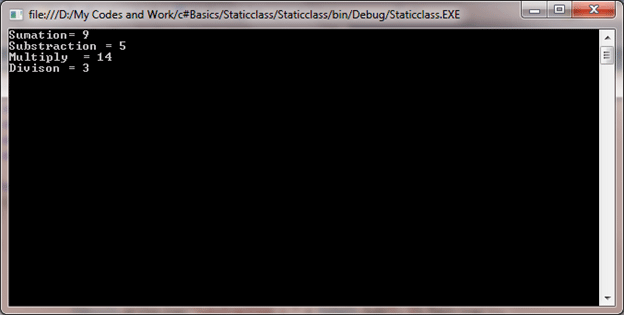
Conclusion
In this article, we saw what Static class is and how to work with this class. Thanks for Reading.