We can use different type identifiers when the queues are opened. Table 1 shows the syntax of the queue name for specific types.
Format Name
Format name can be given to open queue. The formatname is used for searching the queue in the Active Directory to get the host where the queue is located. In a disconnected environment, where the queue cannot be reached at the time the message is sent, it is necessary to use the format name.
Syntax of the format name queue is:
MessageQueue queue= new MessageQueue( @ "FormatName : Public=023423DFG-0984-3w45-K987-12638NU979HH")
Table 1 |
Queue Type |
Syntax |
Private queue |
MachineName\Private$\QueueName |
Public queue |
MachineName\QueueName |
Journal queue |
MachineName\QueueName\Journal$ |
Machine Journal queue |
MachineName\Journal$ |
Machine dead letter queue |
MachineName\DeadLetter$ |
Machine transactional dead-letter queue |
MachineName\XactDeadLetter$ |
Sending a Message
By using the Send method of the MessageQueue class, you can send the message to the queue. The object passed as an argument of the Send() Method is serialized queue. The Send() method is overloaded so that a Label and a MessageQueueTransaction object can be passed. Now we will write a small example to check if the queue exists and if it doesn't exist, a queue is created. Then the queue is opened and the message "First Message" is sent to the queue using the Send() method.
The path name specifies "." for the server name, which is the local system. Path name to private queues only works locally. Add the following code 4 in your Visual Studio C# console environment.
Code 4:
using System;
using System.Messaging;
namespace FirstQueue
{
class Program
{
static void Main(string[] args)
{
try
{
if (!MessageQueue.Exists(@".\Private$\FirstQueue"))
{
MessageQueue.Create(@".\Private$\FirstQueue");
}
MessageQueue queue = new MessageQueue(@".\Private$FirstQueue");
queue.Send("First Message ", " Label ");
}
catch (MessageQueueException ex)
{
Console.WriteLine(ex.Message);
}
}
}
}
Build the application and view and run. You can view the message in the Component Management admin tool as shown in figure 3. By opening the message and selecting the body tab of the dialog, you can see the message was formatted using XML.
Receiving Messages
MessageQueue class can be used for reading messages. With the Receive() method, a single message is read and removed from the queue. We will write a small example to read the Private queue FirstQueue.
When you read a message using the XmlMessageFormatter, we have to pass the types of the objects that are read to the constructor or the formatter. In this example (Code 5), the type System.String is passed to the argument array if the XmlMessageFormatter constructor. The following program is read with the Receive() method and then the message body is written to the console.
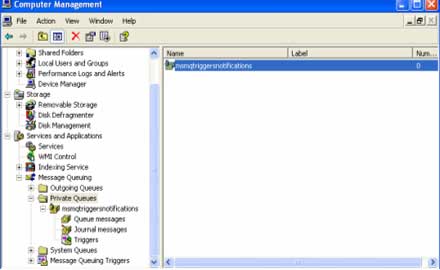
Figure 3
Code 5:
using System;
using System.Messaging;
namespace FirstQueue
{
class Program
{
static void Main(string[] args)
{
MessageQueue queue = new MessageQueue (@".\Private$\FirstQueue");
Queue.Formatter = new XmlMessageFormatter( new string[]("System.String"));
Message Mymessage = queue.Receive();
Console.WriteLine(Mymessage.Body);
}
}
}
Receive() message behaves synchronously and waits until a message is in the queue if there is none. Try out the rest in Visual Studio 2005.
Conclusion
Message Queues can be created with MessageQueue.Create() method. But to create a Message queue you should have administrative rights.