private void SerializeDataSet(SerializationInfo info, StreamingContext context, SerializationFormat remotingFormat)
{
//Implementation goes here
}
Binary Serialization provides enormous improvement in terms of performance and the gain is more significant when we deal with larger dataset (with large number of rows). Following diagram illustrates the same fact.
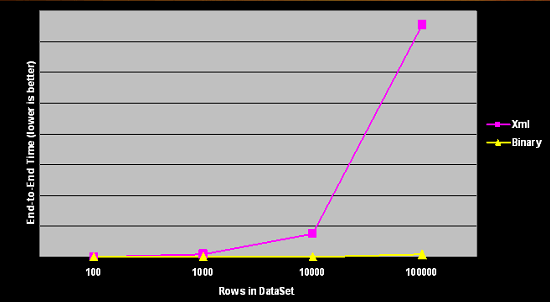
3. New and Much Improved DataTable
DataTable was definitely one of the key classes in ADO.net APIs; however it had a limitation that it was dependent of parent DataSet class it is associated with. Whenever programmers required to work with DataTable, they had to assign the parent DataSet as well. In Framework 2.0, DataTable is an independent entity, it supports all basic XML related functionality provided by the DataSet like ReadXML, ReadXMLSchema, WriteXML, WriteXMLSchema etc. Also now DataTable can be serialized independently using the RemotingFormat property explained in Performance section.
Another new addition in framework 2.0 is the Load Method.
DataTable can be loaded using IDataReader object as described below
DataTable dt =
new DataTable();
dt.Load(reader);
The Load method also supports other overload which accepts LoadOption as described below.
dt.Load(reader, LoadOption.OverwriteChanges);
The LoadOption enum has two other options i.e. PreserveChanges and Upsert. There is one more overload for Load method which also allows the programmer to specify the FillErrorEventHandler delegate to be called when error occurs while loading data.
DataTable also provides a new method for creating a DataTableReader as described below.
DataTableReader dtReader = dt.CreateDataReader();
Please note that DataTableReader class derives from DbDataReader.
public
sealed class DataTableReader : DbDataReader
CreateDataReader method of DataTable makes it easy for programmers who require data not in the DataTable but in a DataReader object. Load and CreateDataReader method can be used to exchange data between DataTable and DataReader.