Hello all :).
I've been tasked with trying to implement secret sharing in image files on a pixel by pixel basis. I am trying to implement Shamir's secret sharing with at least 3 out of 5 shares required in order to reconstruct the original image.
However, I have a few logical issues with my algorithm and design. First off, I will start by saying I have been using the following wikipedia article as a guideline: http://en.wikipedia.org/wiki/Shamir%27s_Secret_Sharing.
So, here is the code I have so far:
[code]
private void buttonCreateShares_Click(object sender, EventArgs e)
{
int a1 = generateRandomNumber();
Thread.Sleep(generateRandomNumber() * 10); //Needed to ensure second random number is different from first.
int a2 = generateRandomNumber();
Bitmap original = new Bitmap(pictureBoxOriginal.Image);
Bitmap share1 = new Bitmap(pictureBoxOriginal.Image);
Bitmap share2 = new Bitmap(pictureBoxOriginal.Image);
Bitmap share3 = new Bitmap(pictureBoxOriginal.Image);
Bitmap share4 = new Bitmap(pictureBoxOriginal.Image);
Bitmap share5 = new Bitmap(pictureBoxOriginal.Image);
Color currentPixel;
int[] newPixelVals = new int[4];
Color newPixel;
for(int x = 0; x < original.Width; x++)
{
for (int y = 0; y < original.Height; y++)
{
currentPixel = original.GetPixel(x, y);
for(int i = 1; i <= 5; i++)
{
newPixelVals[0] = (currentPixel.A + (a1 * i) + (a2* (i*i))) % 255;
newPixelVals[1] = (currentPixel.R + (a1 * i) + (a2* (i*i))) % 255;
newPixelVals[2] = (currentPixel.G + (a1 * i) + (a2* (i*i))) % 255;
newPixelVals[3] = (currentPixel.B + (a1 * i) + (a2* (i*i))) % 255;
newPixel = Color.FromArgb(newPixelVals[0], newPixelVals[1], newPixelVals[2], newPixelVals[3]);
if (i == 1)
{
share1.SetPixel(x, y, newPixel);
}
if (i == 2)
{
share2.SetPixel(x, y, newPixel);
}
if (i == 3)
{
share5.SetPixel(x, y, newPixel);
}
if (i == 4)
{
share4.SetPixel(x, y, newPixel);
}
if (i == 5)
{
share5.SetPixel(x, y, newPixel);
}
}
}
}
pictureBoxShare1.Image = share1;
pictureBoxShare2.Image = share2;
pictureBoxShare3.Image = share3;
pictureBoxShare4.Image = share4;
pictureBoxShare5.Image = share5;
}
[/code]
This (as I could have predicted) only changes the colours of the pixels, and even then not very well, so the original image is still plainly visible thus rendering secret sharing useless. I'm really not entirely sure how to approach this where I am processing the image pixel by pixel.
Here is a sample output:
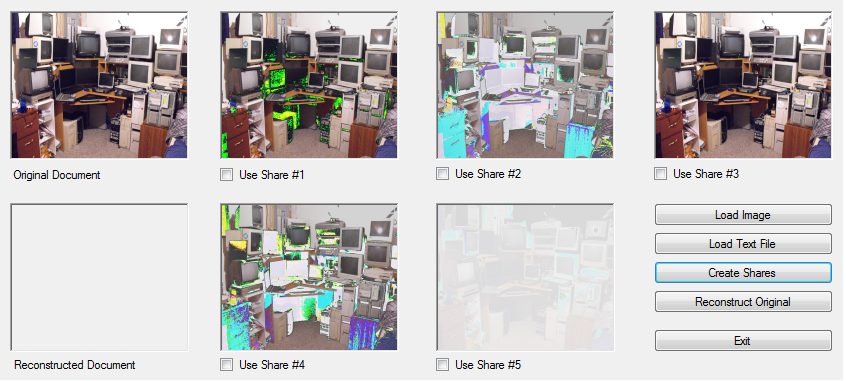
For some reason as you can see, Share #3 always is identitical to the original document, which doesn't seem to make a whole lot of sense to me.
Thanks for any help, advice and tips :).
- David