App.cs
{ #if DEBUG if (System.Diagnostics.Debugger.IsAttached) { this.DebugSettings.EnableFrameRateCounter = true; } #endif Frame rootFrame = Window.Current.Content as Frame; // Do not repeat app initialization when the Window already has content, // just ensure that the window is active if (rootFrame == null) { // Create a Frame to act as the navigation context and navigate to the first page
rootFrame = new Frame(); var viewModel = new MainWindowModel(); MainPage main = new MainPage(); main.DataContext = viewModel;
// TODO: change this value to a cache size that is appropriate for your application rootFrame.CacheSize = 1; if (e.PreviousExecutionState == ApplicationExecutionState.Terminated) { // TODO: Load state from previously suspended application } // Place the frame in the current Window Window.Current.Content = rootFrame; }
DataAccess.cs
{ readonly List<Menu> _menu; public MenuRespository() { if(_menu==null) { _menu = new List<Menu>(); } _menu.Add(Menu.CreateMenu("/Photos/Gas-50.png", "t")); _menu.Add(Menu.CreateMenu("/Photos/Gas-50.png", "t")); _menu.Add(Menu.CreateMenu("/Photos/Gas-50.png", "t")); _menu.Add(Menu.CreateMenu("/Photos/Gas-50.png", "t")); _menu.Add(Menu.CreateMenu("/Photos/Gas-50.png", "t")); _menu.Add(Menu.CreateMenu("/Photos/Gas-50.png", "t")); _menu.Add(Menu.CreateMenu("/Photos/Gas-50.png", "t")); } public List<Menu> GetMenu() { return new List<Menu>(_menu); } }
Menu.cs
public class Menu { public static Menu CreateMenu(string iconthumb, string text) { return new Menu { IconThumb = iconthumb, Text = text }; } public string IconThumb { get; set; } public string Text { get; set; } }
MainWindowsModel
public class MainWindowModel :ViewModelBase { readonly MenuRespository _menuRepository; ObservableCollection<ViewModelBase> _viewModels; public MainWindowModel() { _menuRepository = new MenuRespository(); //create an instance of our viewmodel add it to our collection MenuListViewModel viewModel = new MenuListViewModel(_menuRepository); this.ViewModels.Add(viewModel); } public ObservableCollection<ViewModelBase> ViewModels { get { if(_viewModels==null) { _viewModels = new ObservableCollection<ViewModelBase>(); } return _viewModels; } } }
ViewBase
public abstract class ViewModelBase :INotifyPropertyChanged, IDisposable { protected ViewModelBase() { } public event PropertyChangedEventHandler PropertyChanged; protected virtual void OnPropertyChanged(string propertyName) { PropertyChangedEventHandler hander = this.PropertyChanged; if(hander!=null) { var e = new PropertyChangedEventArgs(propertyName); hander(this, e); } } public void Dispose() { this.Dispose(); } protected virtual void OnDispose() { } }
MenuListBox.xaml into
"<UserControl x:Class="MixNokia.View.MenuListBox" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local="using:MixNokia.View" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" mc:Ignorable="d"> <StackPanel> <ListBox ItemsSource="{Binding AllMenu}"> <ListBox.ItemTemplate> <DataTemplate> <StackPanel Orientation="Horizontal"> <Image Source="{Binding IconThumb}" Width="40" Height="40" VerticalAlignment="Center" HorizontalAlignment="Left"></Image> <TextBlock Text="{Binding Text}" FontSize="15" Foreground="#CCCCCC" VerticalAlignment="Center" HorizontalAlignment="Center"></TextBlock> </StackPanel> </DataTemplate> </ListBox.ItemTemplate> </ListBox> </StackPanel> </UserControl> The final
<Page x:Class="MixNokia.MainPage" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local="using:MixNokia" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:vm="using:MixNokia.ViewModel" xmlns:v="using:MixNokia.View" mc:Ignorable="d"> <Page.DataContext> <vm:MainWindowModel /> </Page.DataContext>Mainpages.
<ListBox x:Name="lstMainMenu" SelectionChanged="lstMainMenu_SelectionChanged" Background="Transparent" ItemsSource="{Binding ViewModels}">I'm developing on universal..
When debuger is loading then listbox show off "mixnokia.viewmodel.
menulistviewmodel "
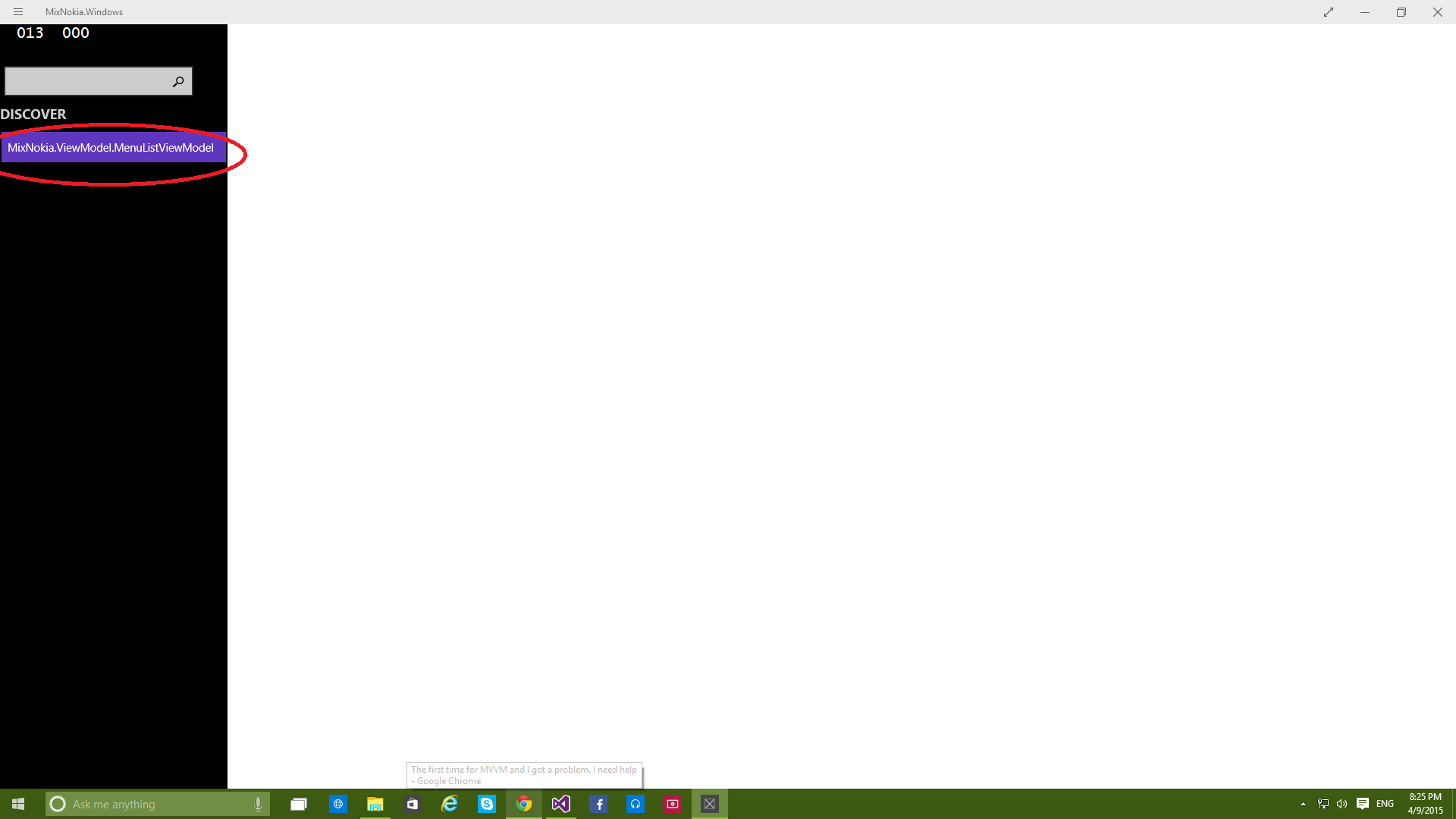