My earlier post was about the basic CRUD operations on entities with relations. We had discussed about creating simple association and querying as well as Invoking CRUD operation onto them. As I have mentioned there, here in this article we will have an in-depth look into Presentation Model and it's mode of handling related multiple entities.
Introduction; What is Presentation Model??
The Presentation Model deals with data from more than one entities which are related to each other and projecting it as one entity.
We usually come across situations where there is not a need to expose all the entities to the client side either for security reasons or else to avoid unnecessary code at client side. So instead of exposing everything as separate entities we can aggregate the data as a separate dummy to client or for presentation layer and can invoke operations onto it.
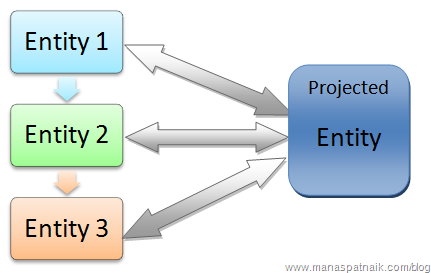
Case Study
Let's check a Data Model from AdventureWorksLT Database for online transactions. AdventureWorksLT is a sample SQL Server database from Microsoft. The following image shows the database schema.
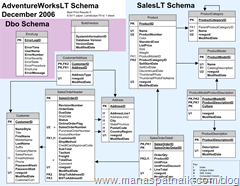
More details regarding the database can be found here. Also you can download database samples from codeplex with following links AdventureWorksLT 2005 Version, AdventureWorksLT 2008 Version. For Presentation model scenario I am going to use a part of the Data Model which involves Customer and Address.
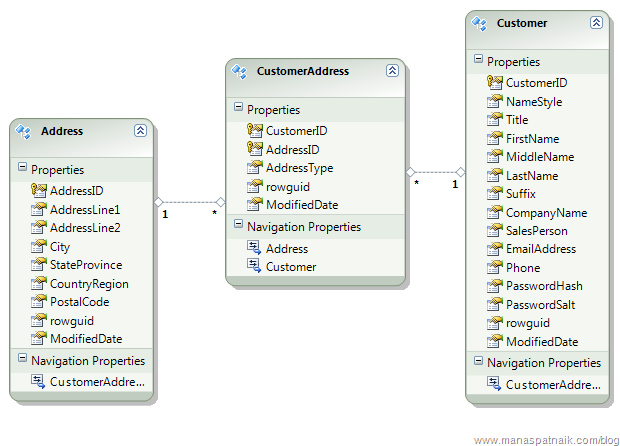
Untill now normally we exposed Customer, CustomerAddress and Address to the client side as 3 separate entities and applied an association between them and then showed the results by joining them over by LINQ at client side. Instead of above procedure we will have a look into how to create a dummy entity and allowing only required combined property to the client side.
Setting Up the Project
The first steps involved here are to create a class for entity at server side project which will hold the custom properties we want to propagate. Let's name it CustomerPresentationModel.cs.
- public class CustomerPresentationModel
- {
- [Key]
- public int CustomerID { get; set; }
- public string FirstName { get; set; }
- public string LastName { get; set; }
- public string EmailAddress { get; set; }
- public string Phone { get; set; }
- public string AddressLine1 { get; set; }
- public string AddressLine2 { get; set; }
- public string City { get; set; }
- public string StateProvince { get; set; }
- public string PostalCode { get; set; }
- public int AddressID { get; set; }
- public DateTime AddressModifiedDate { get; set; }
- public DateTime CustomerModifiedDate { get; set; }
- }
Well the basic step needs no elaboration, you can refer to my previous post for setting up a project using Entity Framework and RIA. Download the AdventureLT database and create a project as mentioned over my earlier articles. Add a domain service named ADVWORKDomainService with out selecting entity from the wizard.
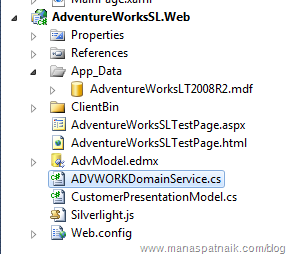
Querying Data from Data Model
The first step involves adding a method that will return all the Customers with properties we want to expose. So create a method within the domainservice at server side project that will fetch the data from the Customer, CustomerAddress and Address entities and project it as CustomerPresentationModel.
- public IQueryable<CustomerPresentationModel> GetCustomersWithAddress()
- {
- return from c in this.ObjectContext.Customers
- join ca in this.ObjectContext.CustomerAddresses on c.CustomerID equals ca.CustomerID
- join a in this.ObjectContext.Addresses on ca.AddressID equals a.AddressID
- select new CustomerPresentationModel()
- {
- CustomerID = c.CustomerID,
- FirstName = c.FirstName,
- LastName = c.LastName,
- EmailAddress = c.EmailAddress,
- Phone = c.Phone,
- AddressLine1 = a.AddressLine1,
- AddressLine2 = a.AddressLine2,
- City = a.City,
- StateProvince = a.StateProvince,
- PostalCode = a.PostalCode,
- AddressID = a.AddressID,
- AddressModifiedDate = a.ModifiedDate,
- CustomerModifiedDate = c.ModifiedDate
- };
- }
Build the project and we can find the CustomerPresentationModel only is propagated to client side instead of all 3 separate entities.
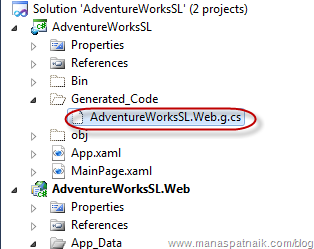
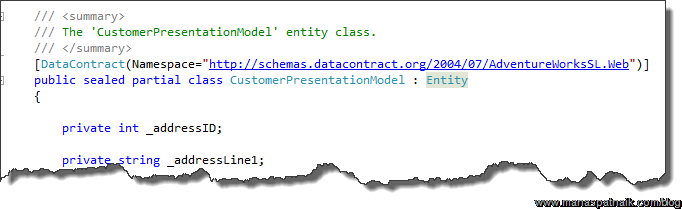
Execute this method asynchronously from Silverlight project and bind the result to data grid.
- private void LayoutRoot_Loaded(object sender, RoutedEventArgs e)
- {
- biLoading.IsBusy = true;
- //Module lvel DomainContext
- cont=new ADVWORKDomainContext();
- //Call the Domain Service Method to fill the CustomerPresentationModel from 3 entities
- System.ServiceModel.DomainServices.Client.LoadOperation<CustomerPresentationModel> lp=
- cont.Load(cont.GetCustomersWithAddressQuery());
- //Attach OnCompleted Event handaler
- lp.Completed+=new EventHandler(lp_Completed);
- //Create Pagination for Grid
- System.Windows.Data.PagedCollectionView pagedcollview = new System.Windows.Data.PagedCollectionView(lp.Entities);
- dpCustomers.Source = pagedcollview;
- //Assign Paged Collection to the Grid
- dgCustomers.ItemsSource = pagedcollview;
- }
On executing the project we can find the result as follows.
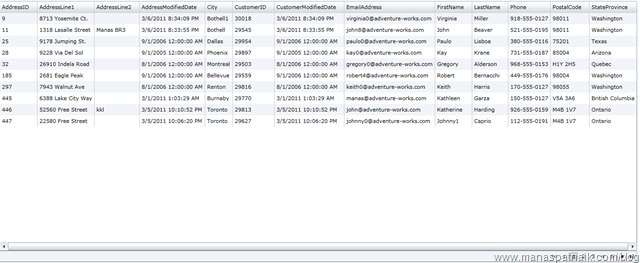
Updating the Presentation Model
Until now we were able to fetch the data from the Data layer using a presentation model. Obiviously the next step involves updating the entities from the presented model from Silverlight page. So we need to add another method to domainservice class on server side to save the changes back to the model and database. Let's add a method with Update Attribute wrapper.
- [Update]
- public void UpdateCustomer(CustomerPresentationModel customerPM)
- {
- Customer customerEntity = this.ObjectContext.Customers.Where(c => c.CustomerID == customerPM.CustomerID).FirstOrDefault();
- CustomerAddress customerAddressEntity = this.ObjectContext.CustomerAddresses.Where(ca => ca.CustomerID == customerPM.CustomerID && ca.AddressID == customerPM.AddressID).FirstOrDefault();
- Address addressEntity = this.ObjectContext.Addresses.Where(a => a.AddressID == customerPM.AddressID).FirstOrDefault();
- customerEntity.FirstName = customerPM.FirstName;
- customerEntity.LastName = customerPM.LastName;
- customerEntity.EmailAddress = customerPM.EmailAddress;
- customerEntity.Phone = customerPM.Phone;
- addressEntity.AddressLine1 = customerPM.AddressLine1;
- addressEntity.AddressLine2 = customerPM.AddressLine2;
- addressEntity.City = customerPM.City;
- addressEntity.StateProvince = customerPM.StateProvince;
- addressEntity.PostalCode = customerPM.PostalCode;
- CustomerPresentationModel originalValues = this.ChangeSet.GetOriginal(customerPM);
- if (originalValues.FirstName != customerPM.FirstName ||
- originalValues.LastName != customerPM.LastName ||
- originalValues.EmailAddress != customerPM.EmailAddress ||
- originalValues.Phone != customerPM.Phone)
- {
- customerEntity.ModifiedDate = DateTime.Now;
- }
- if (originalValues.AddressLine1 != customerPM.AddressLine1 ||
- originalValues.AddressLine2 != customerPM.AddressLine2 ||
- originalValues.City != customerPM.City ||
- originalValues.StateProvince != customerPM.StateProvince ||
- originalValues.PostalCode != customerPM.PostalCode)
- {
- addressEntity.ModifiedDate = DateTime.Now;
- }
- this.ObjectContext.SaveChanges();
- }
The following piece of code of the method above creates 3 entities based on their ID.
- Customer customerEntity = this.ObjectContext.Customers.Where(c => c.CustomerID == customerPM.CustomerID).FirstOrDefault();
- CustomerAddress customerAddressEntity = this.ObjectContext.CustomerAddresses.Where(ca => ca.CustomerID == customerPM.CustomerID && ca.AddressID == customerPM.AddressID).FirstOrDefault();
- Address addressEntity = this.ObjectContext.Addresses.Where(a => a.AddressID == customerPM.AddressID).FirstOrDefault();
and then the next steps follows with assigning the changed values, followed by saving the changes.
Conclusion
Hence the Presentation Model are the Custom Entities built for the presentation layer instead directly the direct data model structure. As I used to say earlier the implementation is completely dependent on the scenario and security. Hope this article is detailed enough to explain how to start with presentation model. Keep suggesting
.