Here is Part 1
A JQ Grid is a light-weight open source grid developed in JavaScript Jquery to fill data from the database.
To download the JQ Grid js file go to the following site:
http://www.trirand.com/blog/?page_id=6.
Now I will create an ASP.Net application where I will display the record from an array through the JQ Grid.
Step 1:
In Visual Studio 2010 first create a simple ASP.Net MVC3 web application project named "mvc3test1".
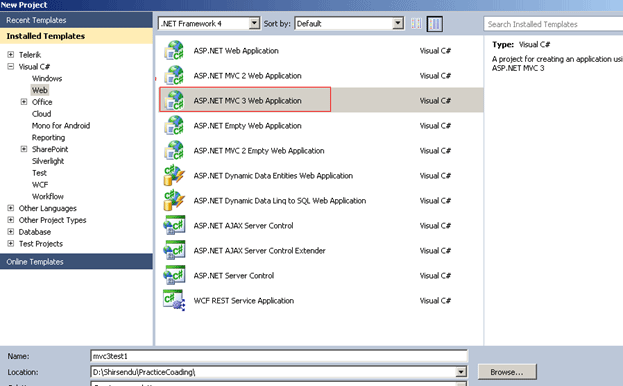
Figure 1:
Step 2:
After that add the required JS file and folder for the JQ grid. The folder and file structure will look as shown in the following figure1 marked with red.
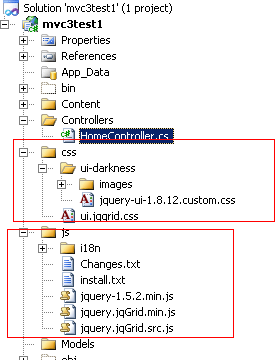
Figure 2:
See here in the "css" folder we have a style sheet named "jquery-ui-1.8.12.custom.css" and also under the "js" folder see the required "js" file for jq grid.
Step 3:
Now go to the "Index.cshtml" under the "Views" folder.
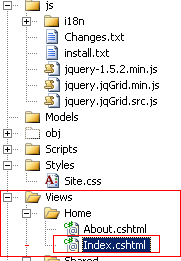
Now the required Jquery file for jq grid is:

Step 4:
Now copy the following code there:
@{
ViewBag.Title = "Home Page";
}
<link href="../css/ui-darkness/jquery-ui-1.8.12.custom.css" rel="stylesheet" type="text/css" />
<link href="../css/ui.jqgrid.css" rel="stylesheet" type="text/css" />
<script src="../js/jquery-1.5.2.min.js" type="text/javascript"></script>
<script src="../js/i18n/grid.locale-en.js" type="text/javascript"></script>
<script src="../js/jquery.jqGrid.min.js" type="text/javascript"></script>
<script src="../Scripts/json2-min.js" type="text/javascript"></script>
<script src="../js/jquery.jqGrid.src.js" type="text/javascript"></script>
<script type="text/javascript">
$(document).ready(function () {
jQuery("#list1").jqGrid({
datatype: "local",
colNames: ['Inv No', 'Date', 'Client', 'Amount', 'Tax', 'Total', 'Notes'],
colModel: [
{ name: 'id', index: 'id', width: 60, sorttype: "int" },
{ name: 'invdate', index: 'invdate', width: 90, sorttype: "date" },
{ name: 'name', index: 'name', width: 100 },
{ name: 'amount', index: 'amount', width: 80, align: "right", sorttype: "float" },
{ name: 'tax', index: 'tax', width: 80, align: "right", sorttype: "float" },
{ name: 'total', index: 'total', width: 80, align: "right", sorttype: "float" },
{ name: 'note', index: 'note', width: 150, sortable: false }
],
multiselect: true,
rowNum: 10,
rowList: [5, 10, 20, 50, 100],
pager: jQuery('#pager1'),
sortorder: "desc",
viewrecords: true,
subGrid: true,
subGridUrl: 'Default.aspx',
subGridModel: [{ name: ['No', 'Item', 'Qty', 'Unit', 'Line Total'],
width: [55, 200, 80, 80, 80]
}
],
caption: "Manipulating Array Data"
});
jQuery("#list1").jqGrid('navGrid', '#pager1', { del: false, add: false, edit: false }, {}, {}, {}, { multipleSearch: true });
var mydata = [
{ id: "1", invdate: "2007-10-01", name: "test", note: "note", amount: "200.00", tax: "10.00", total: "210.00" },
{ id: "2", invdate: "2007-10-02", name: "test2", note: "note2", amount: "300.00", tax: "20.00", total: "320.00" },
{ id: "3", invdate: "2007-09-01", name: "test3", note: "note3", amount: "400.00", tax: "30.00", total: "430.00" },
{ id: "4", invdate: "2007-10-04", name: "test", note: "note", amount: "200.00", tax: "10.00", total: "210.00" },
{ id: "5", invdate: "2007-10-05", name: "test2", note: "note2", amount: "300.00", tax: "20.00", total: "320.00" },
{ id: "6", invdate: "2007-09-06", name: "test3", note: "note3", amount: "400.00", tax: "30.00", total: "430.00" },
{ id: "7", invdate: "2007-10-04", name: "test", note: "note", amount: "200.00", tax: "10.00", total: "210.00" },
{ id: "8", invdate: "2007-10-03", name: "test2", note: "note2", amount: "300.00", tax: "20.00", total: "320.00" },
{ id: "9", invdate: "2007-09-01", name: "test3", note: "note3", amount: "400.00", tax: "30.00", total: "430.00" }
];
for (var i = 0; i <= mydata.length; i++)
jQuery("#list1").jqGrid('addRowData', i + 1, mydata[i]);
});
</script>
<h2>@ViewBag.Message</h2>
<p>
JQ Grid in ASP.Net MVC3 razor
</p>
<div id="pager" ></div>
<br />
<br />
<table id="list1" ></table>
<div id="pager1" ></div>
Step 5:
Now the coding screen shot for the search facility in jq grid is:

jQuery("#list1").jqGrid('navGrid', '#pager1', { del: false, add: false, edit: false }, {}, {}, {}, { multipleSearch: true });
Now run the application; it will look like that.
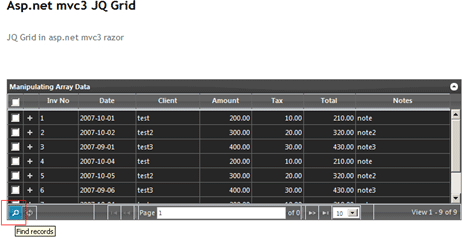
See the grid. Now click the search Button which I marked with red. After clicking that you will see:
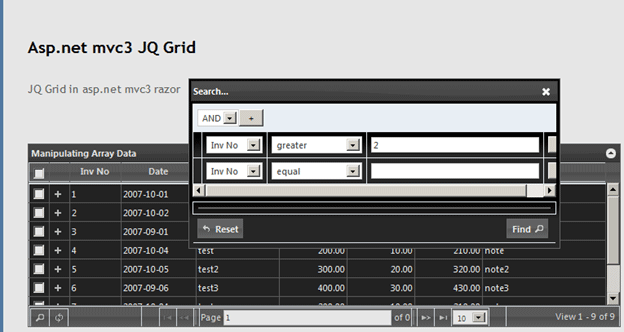
See here the "search" Window Opens. Now we are giving the condition that "Inv No" greater than 2 will display. Now press the "Find" Button. It will look like the following figure:
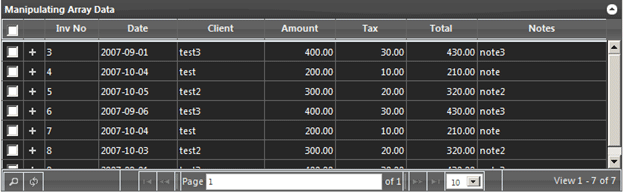
See here the only records showing are the ones with the "inv no" greater than 1.
Conclusion: So in this application we have seen how to do a search operation in a jq grid using a razor view engine in ASP.Net MVC 3.