Creating a Button
The Button element represents a WPF Button control in XAML.
<Button></Button>
The Width and Height attributes of the Button element represent the width and the height of a Button. The Content property of the Button element sets the text of a button. The x:Name attribute represents the name of the control, which is a unique identifier of a control.
The code snippet in Listing 1 creates a Button control and sets the name, height, width, and content of a Button control.
Button x:Name="DrawCircleButton" Height="80" Width="150"
Content="Draw Circle" >
</Button>
Listing 1
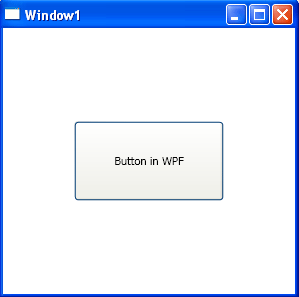
Figure 1
As you can see from Figure 1, by default the Button is place in the center of the page. We can place a Button control where we want by using the Margin, VerticalAlignment and HorizontalAlignment attributes that sets the margin, vertical alignment, and horizontal alignment of a control.
The code snippet in Listing 2 sets the position of the Button control in the left top corner of the page.
<Button x:Name="DrawCircleButton" Height="30" Width="100"
Content="Draw Circle"
Margin="10,10,0,0" VerticalAlignment="Top"
HorizontalAlignment="Left">
</Button>
Listing 2
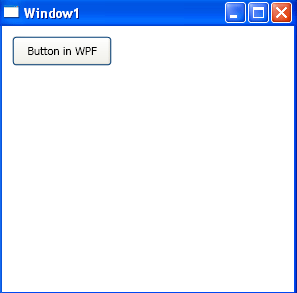
Figure 2
Adding a Button Click Event Handler
The Click attribute of the Button element adds the click event handler. The following code adds the click event handler for a Button.
<Button x:Name="Random Number" Click="RandomNumber_Click">
</Button>
The code for the click event handler looks like following.
private void RandomNumber_Click(object sender, RoutedEventArgs e)
{
}
Now, whatever code you write in the click event handler that will be executed on the Button click. The code listed in Listing 3 creates a random number the Button click event handler.
public void RandomNumber_Click(object sender, EventArgs e)
{
Random generator = new Random();
int randomValue;
randomValue = generator.Next(1, 10);
textBlock1.Text += " " + randomValue.ToString();
}
Listing 3
The Code of the Windows.xaml :
<Window x:Class="HelloWPF.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Window1" Height="300" Width="300">
<Grid Background="Azure">
<Button Height="23" Margin="15,15,125,0" Name="RandomNumber" VerticalAlignment="Top" Click="RandomNumber_Click" Background="Blue">Random Number
</Button>
<ScrollViewer Margin="0,50,0,0">
<TextBlock Name="textBlock1" TextWrapping="Wrap" FontSize="20" FontWeight="Bold" />
</ScrollViewer>
</Grid>
</Window>
The Code of the Windows.xaml.cs:
public void RandomNumber_Click(object sender, EventArgs e)
{
Random generator = new Random();
int randomValue;
randomValue = generator.Next(1, 10);
textBlock1.Text += " " + randomValue.ToString();
}
The Output is :
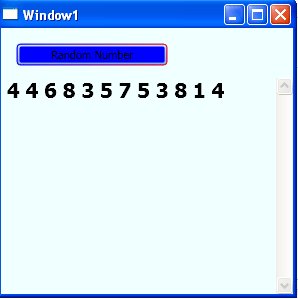
Figure 3
Formatting a Button
Not let's get a little creative. How about we create a Button control with a border formatting, background, and foreground of the Button?
The BorderBrush property of the Button sets a brush to draw the border of a Button. You may use any brush to fill the border. The following code snippet uses a linear gradient brush to draw the border with a combination of red and blue color.
<Button.BorderBrush>
<LinearGradientBrush StartPoint="0,0" EndPoint="1,1" >
<GradientStop Color="Blue" Offset="0" />
<GradientStop Color="Red" Offset="1.0" />
</LinearGradientBrush>
</Button.BorderBrush>
The Background and Foreground properties of the Button set the background and foreground colors of a Button. You may use any brush to fill the border. The following code snippet uses linear gradient brushes to draw the background and foreground of a Button.
<Button.Background>
<LinearGradientBrush StartPoint="0,0" EndPoint="1,1" >
<GradientStop Color="Blue" Offset="0.1" />
<GradientStop Color="Orange" Offset="0.25" />
<GradientStop Color="Green" Offset="0.75" />
<GradientStop Color="Red" Offset="1.0" />
</LinearGradientBrush>
</Button.Background>
<Button.Foreground>
<LinearGradientBrush StartPoint="0,0" EndPoint="1,1" >
<GradientStop Color="Orange" Offset="0.25" />
<GradientStop Color="Green" Offset="1.0" />
</LinearGradientBrush>
</Button.Foreground>
Summary
In this article, I discussed how we can create a Button control in WPF and C#.