There may be scenario where we need to execute a particular query many times and repeatedly. LINQ allows us to make this task very easy by enabling us to create a query and make it compiled always. We call this type of query a compiled query.
Benefits of Compiled Queries
- Query does need to compiled each time so execution of the query is fast.
- Query is compiled once and can be used any number of times.
- Query does need to be recompiled even if the parameter of the query is being changed.
Steps to create a Compiled Query
- Create a static class
- Add namespace System.Data.Linq.
- Use CompiledQuery class to create complied LINQ query.
Let us say we want to create a compiled query to retrieve the entire Person from School database.
- Create Static class

- Define static query in the class
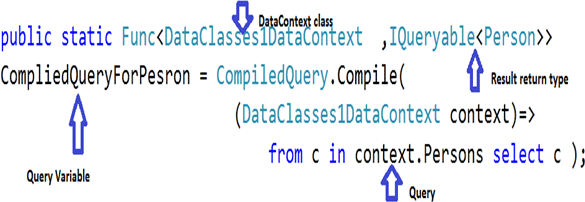
We can pass as many parameters in Func
- First parameter is always the name of the DataContext class created by LINQ to SQL. In our example the name of the DataContext class is DataClass1DataContext.
- Last parameter is the result parameter. This says the return type of the result of the query. This is always a generic IQueryable. In our example it is IQueryable<Person>
- In between first and last parameter we can have parameters to apply against the query.

- Keyword to create complied query is

Let us write a complied query to fetch all the Persons from DataContext
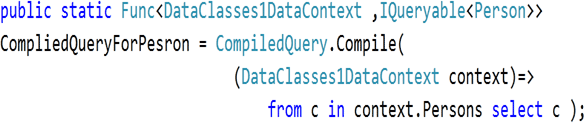
And we will call this compiled query as below; MyCompliedQueries is name of the static class.

Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data.Linq ;
namespace ConsoleApplication5
{
class Program
{
static void Main(string[] args)
{
DataClasses1DataContext context = new DataClasses1DataContext();
var result = MyCompliedQueries.CompliedQueryForPesron(context);
foreach (var r in result)
{
Console.WriteLine(r.FirstName + r.LastName);
}
Console.ReadKey(true);
}
}
static class MyCompliedQueries
{
public static Func<DataClasses1DataContext ,IQueryable<Person>>
CompliedQueryForPesron = CompiledQuery.Compile(
(DataClasses1DataContext context)=>
from c in context.Persons select c );
}
}
Output
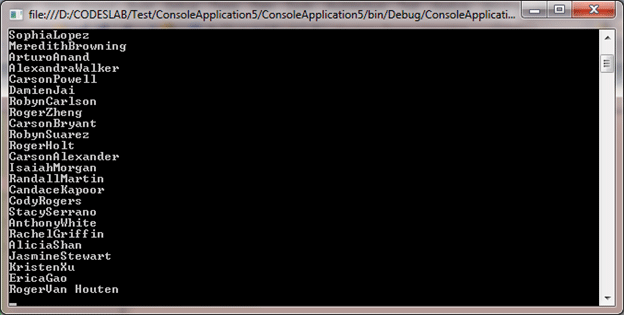
Now if we want to pass some parameter in compiled query
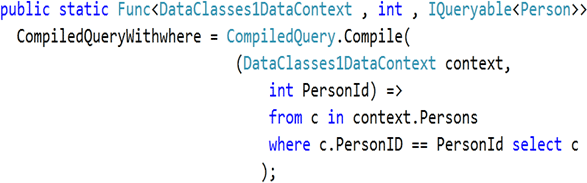
And we will call this query as below,

Program.cs
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data.Linq ;
namespace ConsoleApplication5
{
class Program
{
static void Main(string[] args)
{
DataClasses1DataContext context = new DataClasses1DataContext();
var result = MyCompliedQueries.CompliedQueryForPesron(context);
foreach (var r in result)
{
Console.WriteLine(r.FirstName + r.LastName);
}
Console.ReadKey(true);
}
}
static class MyCompliedQueries
{
public static Func<DataClasses1DataContext ,IQueryable<Person>>
CompliedQueryForPesron = CompiledQuery.Compile(
(DataClasses1DataContext context)=>
from c in context.Persons select c );
}
}
Output
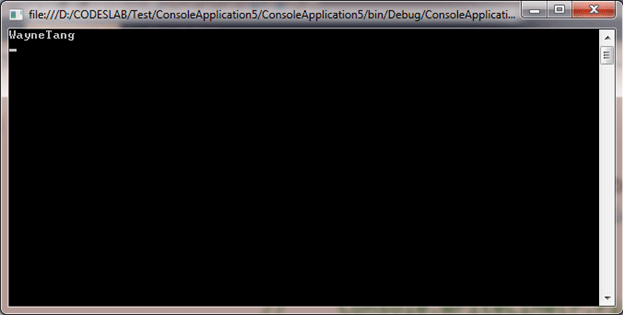