Objective:
Here in this tutorial we are going to bind data from a collection (LIST) to XAML.
We are going to display Image, Name, Age and Email id of a Person.
Output would be something like below.
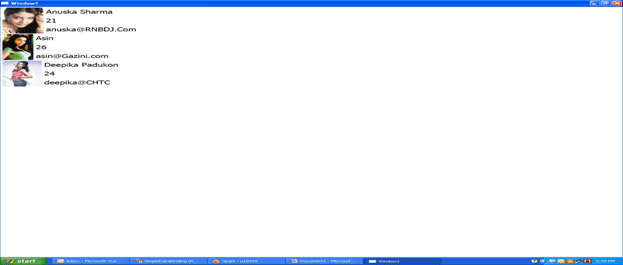
Step 1:
Open Visual Studio 2008; create a new project as WPF application. Give this name as Displayed here.
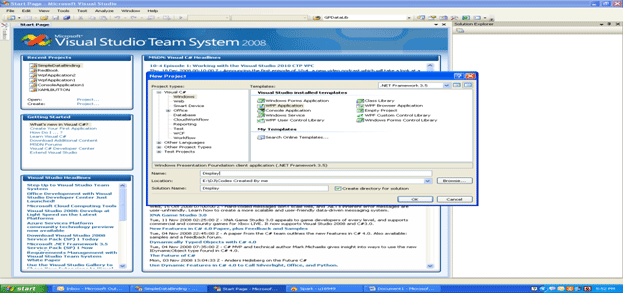
Step 2:
Add a business logic class.
Right click in solution explorer then add a new class called Person.
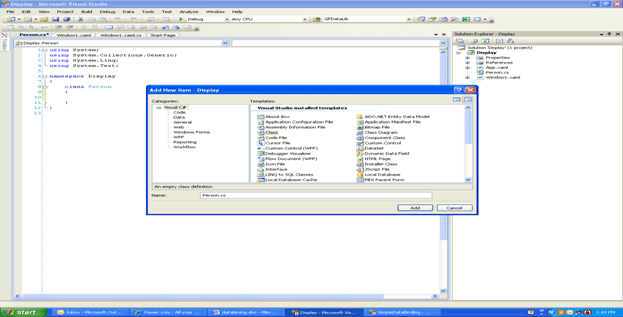
Step 3:
Add following code in Person.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace SimpleDataBinding
{
class Person
{
public string Name { get; set; }
public int Age { get; set; }
public string Image { get; set; }
public string Email { get; set; }
}
}
Step 4:
Add image in Project Folder
Right Click on Solution Explorer and add existing Item. Then browse for image on your PC. Add all images in project.
I have added here, Anuska.jpg, Deepika.jpg, Asin.jpg
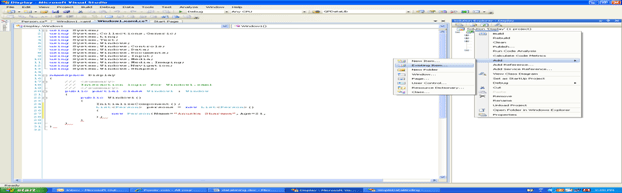
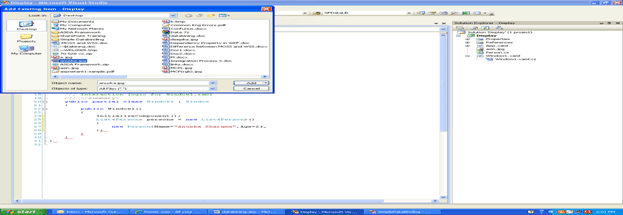
Step 5:
Create a List of persons. Make sure you are creating list inside constructor of Window. Here I am creating list persons as follows.
Add the following code in Window.xaml.cs file.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace Display
{
/// <summary>
/// Interaction logic for Window1.xaml
/// </summary>
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
List<Person> persons = new List<Person>()
{
new Person{Name="Anuska Sharama",Age=21,Email="[email protected]",Image="anuska.jpg"},
new Person {Name="Asin",Age=26,Email="[email protected]",Image="asin.jpg"},
new Person{Name="Deepika",Age=25,Email="[email protected]",Image="deepika.jpg"}
};
}
}
}
Step 6:
Now it is time to write XAML code and bind collection persons to List of XAML
Create a Listbox in XAML as follows
<ListBox x:Name="list1">
</ListBox>
Name it as list1. Then bind this list1 to collection persons. So again go to Windows.xaml.cs file and add this line of code after creating collection persons
list1.ItemsSource = persons;
Window1.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace Display
{
/// <summary>
/// Interaction logic for Window1.xaml
/// </summary>
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
List<Person> persons = new List<Person>()
{
new Person{Name="Anuska Sharama",Age=21,Email="[email protected]",Image="anuska.jpg"},
new Person {Name="Asin",Age=26,Email="[email protected]",Image="asin.jpg"},
new Person{Name="Deepika",Age=25,Email="[email protected]",Image="deepika.jpg"}
};
list1.ItemsSource = persons;
}
}
}
Step 7:
Now add a Item Template and Data template inside listbox in xaml file.
<ListBox x:Name="list1">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
Step 8:
Bind images to List box.
Add following code
<Image Source="{Binding Image}" Height="100" Stretch="UniformToFill" />
If you run at this point, you should be able to see images as output.
Step 9:
Now again add Stack panel with orientation Vertical. Then add three labels to display Name, age and email.
<StackPanel Orientation="Vertical">
<Label FontFamily="Tahoma" FontSize="20" Content="{Binding Name}" />
<Label FontFamily="Tahoma" FontSize="20" Content="{Binding Age}" >
<Label FontFamily="Tahoma" FontSize="20" Content="{Binding Email}" />
</StackPanel>
Complete Xaml code will look like
<Window x:Class="Display.Window1"
xmlns=http://schemas.microsoft.com/winfx/2006/xaml/presentation
xmlns:x=http://schemas.microsoft.com/winfx/2006/xaml
Title="Window1" Height="300" Width="300">
<Grid>
<ListBox x:Name="list1">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<Image Source="{Binding Image}" Height="100" Stretch="UniformToFill" />
<StackPanel Orientation="Vertical">
<Label FontFamily="Tahoma" FontSize="20" Content="{Binding Name}" />
<Label FontFamily="Tahoma" FontSize="20" Content="{Binding Age}" />
<Label FontFamily="Tahoma" FontSize="20" Content="{Binding Email}" />
</StackPanel>
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
</Window>
Step 10: Run the code to get the desired output.