The Singleton is a design pattern, which instantiates one instance of the class once it is first called, and maintains that single instance throughout the lifetime of the program. Once it is instantiated, all future requests will be through a single global point of access.

Singleton provides a global, single instance by:
- Making the class create a single instance of itself.
- Allowing other objects to access this instance through a class method that returns a reference to the instance. A class method is globally accessible.
- Declaring the class constructor as private so that no other object can create a new instance.
Implementation in c#
Method 1:
- Make a public class.
- Declare a Private constructor.
- Inside Method create the Instance of the class.
Create a console application and give name singelton1 as follows.
Add a class like below in Singelton1 class. And give its name Singeltonsample1.cs
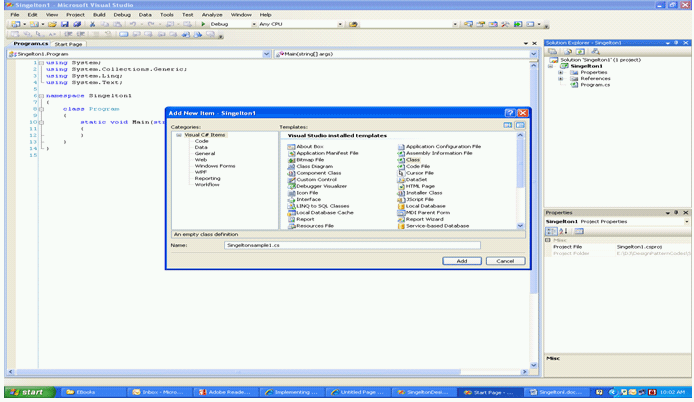
Type this code in SingeltonSample1. Cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Singelton1
{
public class Singeltonsample1
{
public int checkValue = 0;
private static Singeltonsample1 instance;
private Singeltonsample1()
{
}
public static Singeltonsample1 CreateInstance()
{
if (instance == null)
{
instance = new Singeltonsample1();
}
return instance;
}
}
}
Now in Singelton1 class type the below code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Singelton1
{
class Program
{
static void Main(string[] args)
{
Singeltonsample1 instance1 = Singeltonsample1.CreateInstance();
Singeltonsample1 instance2 = Singeltonsample1.CreateInstance();
if (instance1 == instance2)
{
Console.WriteLine("Both Instance are Same, Due to Singelton Pattern");
}
instance1.checkValue = 100;
Console.WriteLine("Value of ChekValue = {0} by instance 1", instance1.checkValue);
instance2.checkValue = 990;
Console.WriteLine("Value of Checkvale = {0} by Instance 2 ", instance2.checkValue);
Console.WriteLine("Final value of CheckValue= {0} and {1}", instance1.checkValue, instance2.checkValue);
Console.WriteLine("Both instnace is updating Same Check Value");
Console.Read();
}
}
}
Press F5 to run in debug mode, Output will come as follows
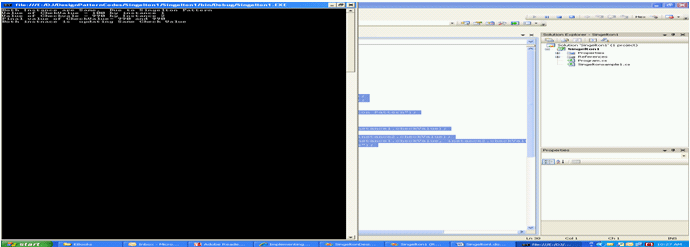
Multithreading Singleton class.
Static initialization is suitable for most situations. When application must delay the instantiation. , use a non-default constructor or perform other tasks before the instantiation, and to work in a multithreaded environment, a different solution is needed. Cases do exist, where Instantiation cannot rely on the common language runtime to ensure thread safety, as in the Static Initialization example. In such cases, specific language capabilities should be used to ensure that only one instance of the object is created in the presence of multiple threads.
To achieve this add one more class. Name it SingeltonSample2.cs Add Class by right clicking on Project and then selecting Add New Class.
Type the below code in this class.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Singelton1
{
public sealed class SingeltonSample2
{
public int checkValue = 0;
private static SingeltonSample2 instance;
private static object _SyncRoot = new object();
private SingeltonSample2()
{
}
public static SingeltonSample2 CreateInstance()
{
if (instance == null)
{
lock (_SyncRoot)
{
if (instance == null)
{
instance = new SingeltonSample2();
}
}
}
return instance;
}
}
}
After this open the Singelton1.Cs and modify the code as follows
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Singelton1
{
class Program
{
static void Main(string[] args)
{
Singeltonsample1 instance1 = Singeltonsample1.CreateInstance();
Singeltonsample1 instance2 = Singeltonsample1.CreateInstance();
if (instance1 == instance2)
{
Console.WriteLine("Both Instance are Same, Due to Singelton Pattern");
}
instance1.checkValue = 100;
Console.WriteLine("Value of ChekValue = {0} by instance 1", instance1.checkValue);
instance2.checkValue = 990;
Console.WriteLine("Value of Checkvale = {0} by Instance 2 ", instance2.checkValue);
Console.WriteLine("Final value of CheckValue= {0} and {1}", instance1.checkValue, instance2.checkValue);
Console.WriteLine("Both instnace is updating Same Check Value");
Console.Read();
Console.WriteLine(" Acheiving Singelton Pattern using Thread implemenetaion");
SingeltonSample2 instance3 = SingeltonSample2.CreateInstance();
SingeltonSample2 instance4 = SingeltonSample2.CreateInstance();
if (instance3 == instance4)
{
Console.WriteLine("Both Instance are Same, Due to Singelton Pattern");
}
instance3.checkValue = 100;
Console.WriteLine("Value of ChekValue = {0} by instance 1", instance3.checkValue);
instance4.checkValue = 990;
Console.WriteLine("Value of Checkvale = {0} by Instance 2 ", instance4.checkValue);
Console.WriteLine("Final value of CheckValue= {0} and {1}", instance3.checkValue, instance4.checkValue);
Console.WriteLine("Both instnace is updating Same Check Value");
Console.Read();
Console.Read();
}
}
}
Code highLighted in yellow is added code there.
Run to get the Output.
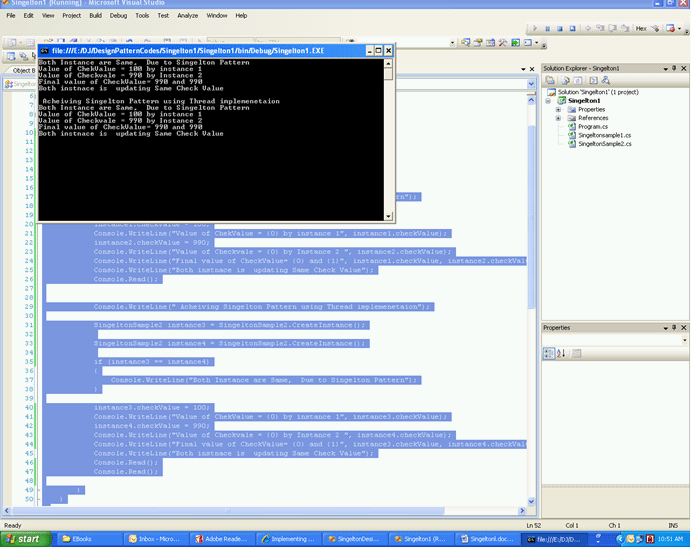