This is a technique in Silverlight to create a Virtual File System in the client machine where a Silverlight application is running. This is used to have direct restricted access to a client operating system file system.
Why Isolated Storage?
Silverlight applications are partially trusted applications, which are running in a sandbox environment. So, Silverlight applications are not allowed to access the file system of the client where it is running, for the security reason. Because it is not safe to allow any Silverlight application to access a client computer, this may harm many important system files. Because we know that a Silverlight application might be a potential malicious application. And while browsing we don't want any harmful Silverlight application to access our file system and harm them.
But sometimes a Silverlight application needs some restricted access to the client local file system for business requirements. Like Reading and Writing user specific file at client location or saving the personal information specific to user at client side etc.
So the above purpose is achieved by Silverlight application using ISOLATED STORAGE.
Cookies and Isolated Storage
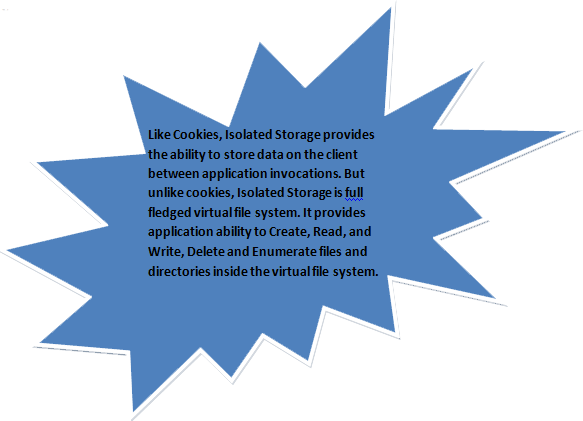
Isolation
Isolated storage is composed of many different unique stores, each of which can be thought of as its own virtual file system. This virtual file system is in isolation with the file system of operating system, such that a malicious Silverlight application can not have access to the original file system.
Location of the Isolated Storage
Operating System |
Location |
Windows VISTA |
<SYSDRIVE>\Users\<user>\AppData\LocalLow\Microsoft\Silverlight\is |
Windows XP |
<SYSDRIVE>\Document and Settings\<user>\Local Setting\Application data\Microsoft\Silverlight\is |
A Silverlight application has access to two different stores.

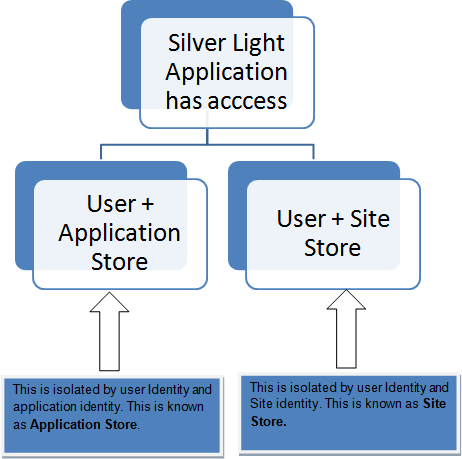

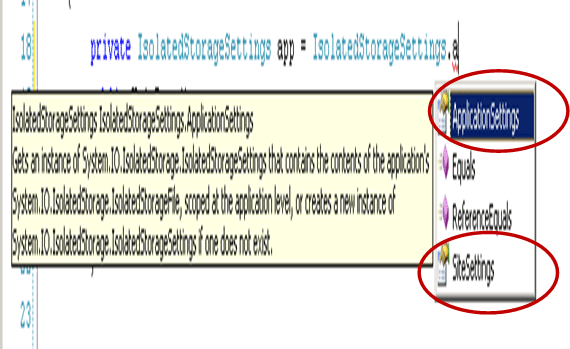
Few Important points
- A Silverlight Application can access only its own application store. It cannot access the store of another application.
- The Application Store is based on the, identity of the user and identity of the application.
- The identity of the application is the full URL of the XAP file of the Silverlight Application. For example www.c-sharpcorner.com\Application1.xap
- Application identity is case insensitive.
Sample:
Objective:
This sample will demonstrate how to use with Application level setting of IsolatedStorageSetting class.
- After entering User Name in the textbox, then the user will click Save Me. That user name will get added in the isolated file created for the application.
- On clicking the Reset Me button, the value saved for the UserName key, will get deleted from the isolated file.
- Each time the application loads, the saved UserName will be displayed in the textbox.
Step 1:
Create a private global variable of the type IsolatedStorageSetting for the Application level.
private IsolatedStorageSettings app = IsolatedStorageSettings.ApplicationSettings;
Step 2:
On the Save button click, we will be checking if checkbox is checked: we will check whether:
- UserName key is existing in App or not. If not, we will add UserName key.
- We will set value for UserName key from the user name text box.
private void btnSave_Click(object sender, System.Windows.RoutedEventArgs e)
{
if (chkSave.IsChecked == true)
{
if (!(app.Contains("UserName")))
{
app.Add("UserName","User Name has not Set ");
}
app["UserName"] = txtUname.Text;
}
}
- App.add() is used to add new key in the application.
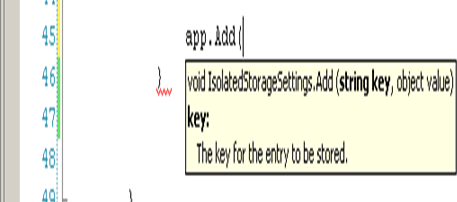
Step 3:
On the Reset button, we are simply removing the key from the app setting.
private void btnReset_Click(object sender, System.Windows.RoutedEventArgs e)
{
app.Remove("UserName");
txtUname.Text = "";
}
Step 4:
On the Page Load, I am displaying the saved value for the key UserName from the isolated storage.
void MainPage_Loaded(object sender, RoutedEventArgs e)
{
if (app.Contains("UserName"))
{
txtUname.Text = app["UserName"].ToString();
}
}
So, the complete code is as below:
MainPage.Xaml
<UserControl x:Class="IsolatedStorageSample1.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Width="Auto" Height="Auto">
<Grid x:Name="LayoutRoot" Background="#FF7F4343" Width="400" Height="400">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="0.385*"/>
<ColumnDefinition Width="0.615*"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="0.25*"/>
<RowDefinition Height="0.157*"/>
<RowDefinition Height="0.593*"/>
</Grid.RowDefinitions>
<TextBox x:Name="txtUname" Margin="10,10,10,10" Grid.Column="1" Text="" TextWrapping="Wrap" Background="#FF230303" BorderThickness="2,2,2,2" FontSize="16" FontWeight="Bold" Foreground="#FFF81616"/>
<TextBlock Margin="8,8,8,8" Text="UserName" TextWrapping="Wrap" FontSize="12" FontWeight="Bold" Foreground="#FF342A2A" FontFamily="Arial Rounded MT Bold"/>
<CheckBox x:Name="chkSave" Margin="10,10,10,10" Grid.Row="1" Content="Save User Name" IsChecked="True" FontWeight="Bold" FontSize="12" BorderThickness="2,2,2,2" Foreground="#FF150808" FontFamily="Arial Rounded MT Bold"/>
<Grid Margin="8,10,12,8" Grid.Column="1" Grid.Row="1">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="0.385*"/>
<ColumnDefinition Width="0.615*"/>
</Grid.ColumnDefinitions>
<Button x:Name="btnSave" Margin="2,2,2,2" Content="Save Me" Grid.Row="0" Grid.Column="0" Click="btnSave_Click"/>
<Button x:Name="btnReset" Margin="2,2,2,2" Content="Reset Me " Grid.Row="0" Grid.Column="1" Click="btnReset_Click"/>
</Grid> </Grid></UserControl>
MainPage.Xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.IO.IsolatedStorage;
namespace IsolatedStorageSample1
{
public partial class MainPage : UserControl
{
private IsolatedStorageSettings app = IsolatedStorageSettings.ApplicationSettings;
public MainPage()
{
InitializeComponent();
Loaded += new RoutedEventHandler(MainPage_Loaded);
}
void MainPage_Loaded(object sender, RoutedEventArgs e)
{
if (app.Contains("UserName"))
{
txtUname.Text = app["UserName"].ToString();
}
}
private void btnSave_Click(object sender, System.Windows.RoutedEventArgs e)
{
if (chkSave.IsChecked == true)
{
if (!(app.Contains("UserName")))
{
app.Add("UserName","User Name has not Set ");
}
app["UserName"] = txtUname.Text;
}
}
private void btnReset_Click(object sender, System.Windows.RoutedEventArgs e)
{
app.Remove("UserName");
txtUname.Text = "";
}
}
}
Output:
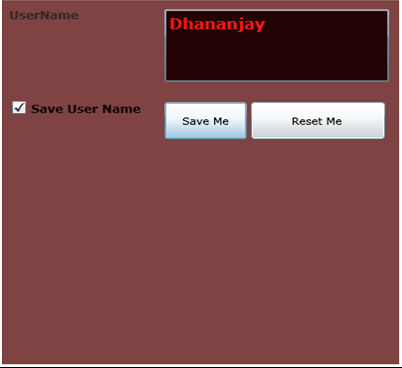
After clicking Refresh again we will get the same output. If we click Reset and then press F5, we will get output as below.
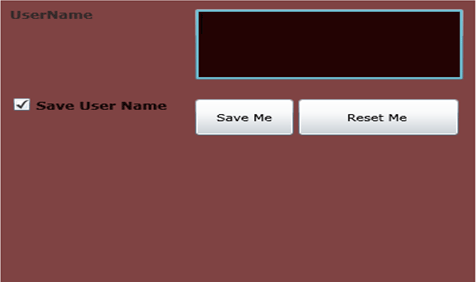
Quota in Isolated Storage
-
A quota is a limit on the amount of isolated storage space that can be used by the application.
-
A Quota Group is a group of isolated Stores that shares the same quota.
-
In Silverlight, stores are grouped by the site. So all the application with same site identity shares the same quota.
-
Isolated Storage can have zero or more quota groups.
-
An Application can request more space for the quota group.

Sample on how to modify the quota?
I have added the following code on the click event of the Save Button. So now the save Button click event is as below. I have modified the above code sample.
private void btnSave_Click(object sender, System.Windows.RoutedEventArgs e)
{
if (chkSave.IsChecked == true)
{
if (!(app.Contains("UserName")))
{
app.Add("UserName","User Name has not Set ");
}
app["UserName"] = txtUname.Text;
}
using (var store = IsolatedStorageFile.GetUserStoreForApplication())
{
int spaceneed = 1024 * 1024 * 5;
if (store.AvailableFreeSpace < spaceneed)
{
if (store.IncreaseQuotaTo(store.Quota + spaceneed))
{
MessageBox.Show("User Has approved the quota Increase");
MessageBox.Show(store.AvailableFreeSpace.ToString());
}
else
{
MessageBox.Show("User Has Not Approved the quota Increase ");
MessageBox.Show(store.AvailableFreeSpace.ToString());
}
}
}
}
Output:
User is getting prompt for Approval.
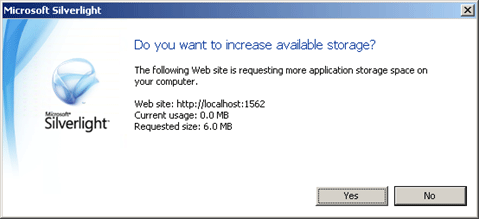
When the user is not approved means selecting "No". The default size is getting displayed in the message box.

When the user is approved means selecting "Yes". The increased size is getting displayed in the message box.

Conclusion:
This article gave a basic introduction of Isolated Storage in Silverlight. It gave a theoretical introduction as well as two samples. Please find the attached source code for your reference.
Thank you for Reading. Happy Coding!