Objective
In this article, I am going to show, how we could achieve projection in LINQ to object. I will explain different types of projection and how to achieve them over a collection using LINQ.
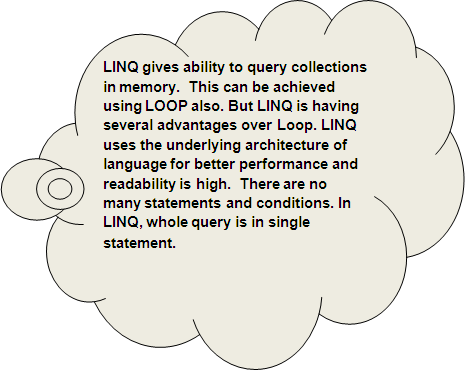
I have created two classes for my explanation purpose. Student class is having details of students and Hostel class is having details of hostel. Both classes are having a properties HostelNumber common. I will be using this property to perform join operations in later articles. Both classes are as below.
Student.cs
namespace LINQtoOBJECT1
{
public class Student
{
public int RollNumber { get; set; }
public string Name { get; set; }
public int Section { get; set; }
public int HostelNumber { get; set; }
}
}
Hostel.cs
namespace LINQtoOBJECT1
{
public class Hostel
{
public int HostelNumber { get; set; }
public int NumberofRooms { get; set; }
}
}
To create collection of students and hostels, I am creating two static functions.
Function to return collection of students.
static List<Student> GetStudents()
{
List<Student> students = new List<Student>
{
new Student() { RollNumber = 1,Name ="Alex " , Section = 1 ,HostelNumber=1 },
new Student() { RollNumber = 2,Name ="Jonty " , Section = 2 ,HostelNumber=2 },
new Student() { RollNumber = 3,Name ="Samba " , Section = 3 ,HostelNumber=1 },
new Student() { RollNumber = 4,Name ="Donald " , Section = 3 ,HostelNumber=2 },
new Student() { RollNumber = 5,Name ="Kristen " , Section = 2 ,HostelNumber=1 },
new Student() { RollNumber = 6,Name ="Mark " , Section = 1 ,HostelNumber=2},
new Student() { RollNumber = 7,Name ="Gibbs " , Section = 1 ,HostelNumber=1 },
new Student() { RollNumber = 8,Name ="Peterson " , Section = 2 ,HostelNumber=2 },
new Student() { RollNumber = 9,Name ="collingwood " , Section = 3 ,HostelNumber=1 },
new Student() { RollNumber = 10,Name ="Brian " , Section = 3 ,HostelNumber=2 }
};
return students;
}
Function to return collection of hostels
static List<Hostel> GetHostel()
{
List<Hostel> hostels = new List<Hostel>
{
new Hostel(){HostelNumber=1 ,NumberofRooms = 100},
new Hostel(){HostelNumber= 2 ,NumberofRooms = 200}
};
return hostels;
}
Now have a look on both codes below, one is using LINQ and other is using LOOP to retrieve data from the list and print.
Note: I will be using above two classes and functions for my entire sample below.
Comparison between LINQ and LOOP
Using LINQ
List<Student> lstStudents = GetStudents();
var students = from r in lstStudents where r.RollNumber >= 3 select r;
foreach (Student student in students)
{
Console.WriteLine(student.Name);
}
Using LOOP
List<Student> lstStudents = GetStudents();
for (int i = 0; i < lstStudents.Count; i++)
{
if (lstStudents[i].RollNumber >= 3)
{
Console.WriteLine(lstStudents[i].Name);
}
}
Output
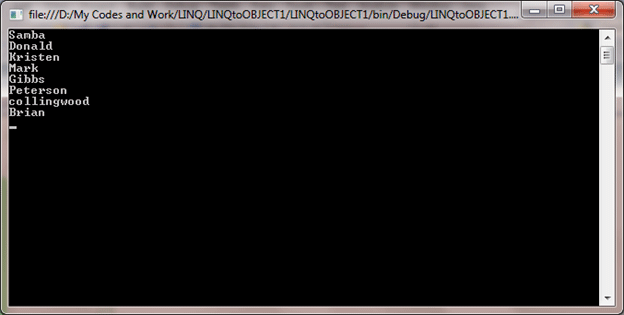
If we see the above code we can say about LINQ
-
It is simple
-
It is single line of statement.
-
It is having good readability.
-
It is IMPERATIVE. It does mean, we need to tell code only what to do not how to do.
And by looking at LOOP, we can say
-
It is not simple
-
It is having many statements.
-
Readability is complex
-
It is DECLARATIVE, it does mean, we need to tell code what to do as well how to do.
Projection in LINQ
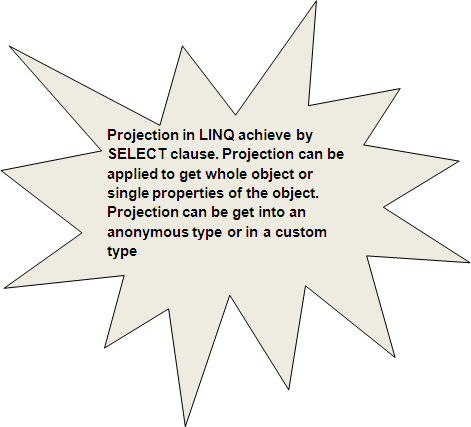
Projecting a single field property
In this projection, we only select single public properties from the resulted object.
List<Student> lstStudents = GetStudents();
IEnumerable<string> students = from r in lstStudents select r.Name;
foreach (string s in students)
Console.WriteLine(s);
While selecting a single property intellisense will work. In above query, I am selecting Name property of Student object and returning it to ther IEnumerable of string.
Output
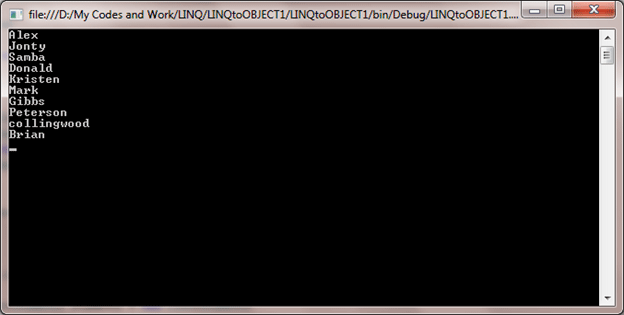
Projecting into anonymous types
When there is requirement to use only part of the resultant object, Anonymous type can come into action. In this we can project part of resultant object into an anonymous type and return to the type var.
List<Student> lstStudents = GetStudents();
var student = from r in lstStudents select new { r.RollNumber, r.Name };
foreach (var a in student)
{
Console.WriteLine(a.RollNumber + ", " + a.Name);
}
If we want we can give property name to anonymous type as below ,
List<Student> lstStudents = GetStudents();
var student = from r in lstStudents select new {AnonRoolNumber= r.RollNumber, AnonName= r.Name };
foreach (var a in student)
{
Console.WriteLine(a.AnonRoolNumber + ", " + a.AnonName );
}
The problem with anonymous type projection is that, we cannot return collection of anonymous type from the LINQ query.
Output
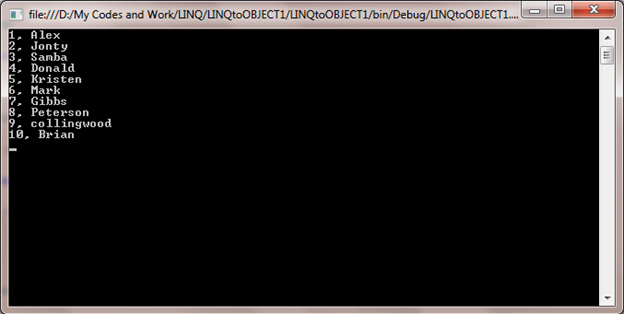
Projecting into custom types
Let, we suppose we need to retrieve result from LINQ query to a custom class. In this case projection into custom class comes into play. Suppose we want to fetch hostelnumber and rollnumber from student object into a new custom class called studenthostel.
class StudentHostel
{
public int CustomRollNumber { get; set; }
public int CustomHostelNumber { get; set; }
}
}
Now we will project the result of LINQ query into this custom class.
List<Student> lstStudents = GetStudents();
IEnumerable<StudentHostel> student = from r in lstStudents select new StudentHostel { CustomHostelNumber = r.HostelNumber , CustomRollNumber = r.RollNumber };
foreach (StudentHostel a in student)
{
Console.WriteLine(a.CustomRollNumber + ", " + a.CustomHostelNumber );
}
In above query I am projecting the resulted object into a custom class and returning that in IEnumerable of custom class StudentHostel in our case.
Output
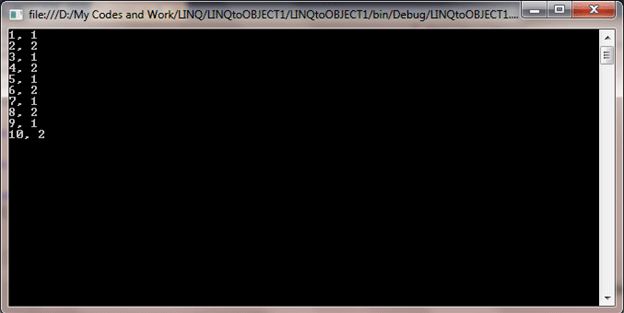
Conclusion
In next part, I will be explaining sorting and filtering. I hope this article was useful to you. Thanks for reading.