Introduction
In this article, I am going to show you how we can achieve filtering and sorting using LINQ to object.Here is the first part
I have created two classes for explanation purposes, Student and Hostel. The Student class has details about a student and the Hostel class has details about a hostel. Both classes have the property HostelNumber in common. I will be using this property to perform join operations in later articles. Both classes are shown below.
Student.cs
namespace LINQtoOBJECT1
{
public class Student
{
public int HostelNumber { get; set; }
public int RollNumber { get; set; }
public string Name { get; set; }
public int Section { get; set; }
}
}
Hostel.cs
namespace LINQtoOBJECT1
{
public class Hostel
{
public int HostelNumber { get; set; }
public int NumberofRooms { get; set; }
}
}
To create and obtain collections of students and hostels, I have created two static functions: GetStudents
and GetHostel .
Function to return collection of students
static List<Student> GetStudents()
{
List<Student> students = new List<Student>
{
new Student() { RollNumber = 1,Name ="Alex " , Section = 1 ,HostelNumber=1 },
new Student() { RollNumber = 2,Name ="Jonty " , Section = 2 ,HostelNumber=2 },
new Student() { RollNumber = 3,Name ="Samba " , Section = 3 ,HostelNumber=1 },
new Student() { RollNumber = 4,Name ="Donald " , Section = 3 ,HostelNumber=2 },
new Student() { RollNumber = 5,Name ="Kristen " , Section = 2 ,HostelNumber=1 },
new Student() { RollNumber = 6,Name ="Mark " , Section = 1 ,HostelNumber=2},
new Student() { RollNumber = 8,Name ="Peterson " , Section = 2 ,HostelNumber=2 },
new Student() { RollNumber = 9,Name ="collingwood " , Section = 3 ,HostelNumber=1 },
new Student() { RollNumber = 10,Name ="Brian " , Section = 3 ,HostelNumber=2 }
};
return students;
}
Function to return collection of hostels
static List<Hostel> GetHostel()
{
List<Hostel> hostels = new List<Hostel>
{
new Hostel(){HostelNumber=1 ,NumberofRooms = 100},
new Hostel(){HostelNumber= 2 ,NumberofRooms = 200}
};
return hostels;
}
Now have a look at both code fragments below. One is using LINQ and the other is using a loop to retrieve and print data from the list.
Note: I will be using the two classes(Student and Hostel) and corresponding functions(GetStudents and GetHostel) for my entire sample below.
Filtering in LINQ
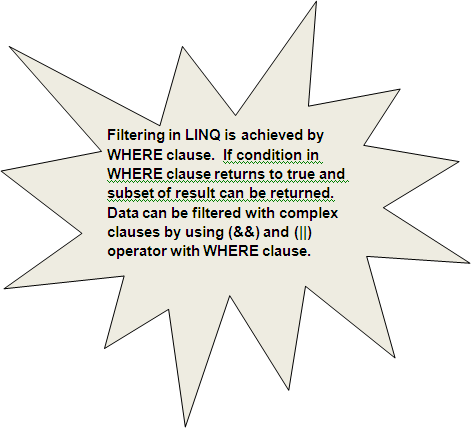
In the code below, I am filtering the result. I am only fetching details from the student with RollNumber = 1.
List<Student> lstStudents = GetStudents();
Student student = (from r in lstStudents where r.RollNumber == 1 select r).First();
Console.WriteLine(student.Name + student.RollNumber + student.HostelNumber + student.Section);
If you examine the LINQ query closely, I am using the First extension method in order to return a single Student. Without the First extension, the default return type for a LINQ query is IEnumerable. If I modify the LINQ statement above by removing the First extension,
Student student = (from r in lstStudents where r.RollNumber == 1 select r);
At compile time we can expect the error shown below:
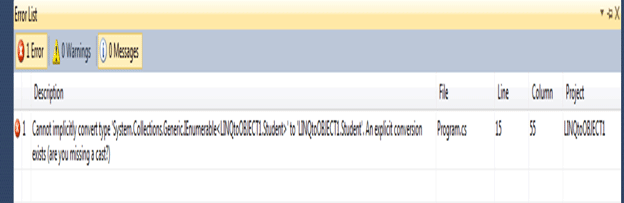
When we run the code containing the First extension above, we get the following output:
Output
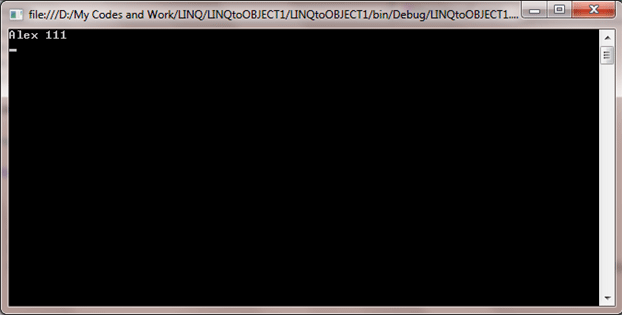
Let's say I want to retrieve the names of all the students whose roll number is more than 3 and who resides in hostel number 2. We can alter our LINQ query where clause to include these conditions:
List<Student> lstStudents = GetStudents();
IEnumerable<string> lstStudentName = from r in lstStudents where r.RollNumber > 3 &&
r.HostelNumber== 2 select r.Name ;
foreach (string name in lstStudentName)
Console.WriteLine(name);
In above LINQ query, I am applying both filtering and projection. The filter is the condition in the where clause that produces a subset of my initial student collection lstStudents. Projection is the select clause which maps the result set of students to the result set of student names.
Output
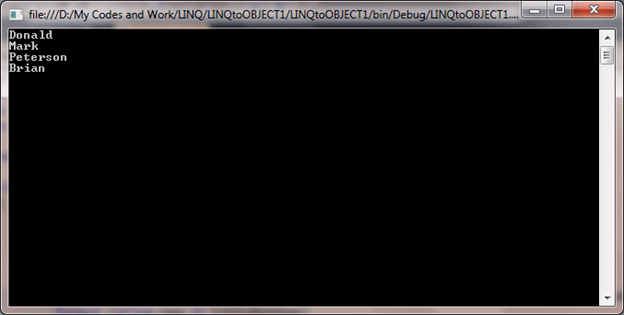
Intermediate values
If I want to have intermediate values in my LINQ query then we introduce the LET clause into action. The LET clause helps us to reduce redundancy in the WHERE clause by allowing us to substitute a variable for an element of the LINQ statement.
IEnumerable<string> lstStudentName = from r in lstStudents let condition = r.RollNumber where condition > 3 && r.HostelNumber== 2 select r.Name ;
The Let clause can have even more complex statements than the simple example shown above, making our LINQ query more readable.
Sorting in LINQ
The OrderBy clause is used for purpose of sorting in LINQ. Let's examine an example of using OrderBy in our LINQ query:
Sorting a single property
The OrderBy clause is used directly before the where clause in order to sort the query result. In the LINQ code shown below, the name of the students will be displayed alphabetically in ascending order.
List<Student> lstStudents = GetStudents();
IEnumerable<string> lstStudentName = from r in lstStudents let condition = r.RollNumber orderby r.Name where condition > 3 && r.HostelNumber== 2 select r.Name ;
foreach (string name in lstStudentName)
Console.WriteLine(name);
Output
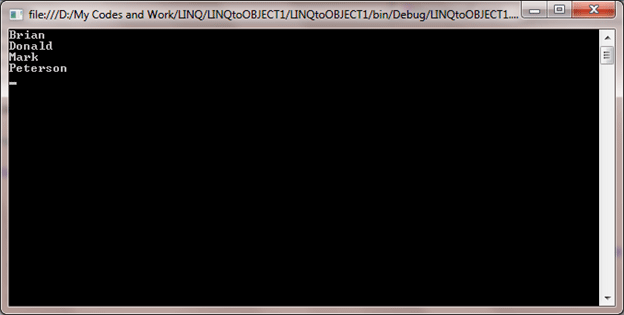
Sorting multiple properties
By putting commas between properties of a student in the OrderBy clause, we can achieve primary and secondary sorting. Sorting will be done left to right. Because I want to sort the roll number property in descending order, I'll use the descending keyword in my LINQ statement. Sorting is performed in ascending order by default.
List<Student> lstStudents = GetStudents();
IEnumerable<Student> lstStudentName = from r in lstStudents let condition = r.RollNumber orderby r.Name,r.RollNumber descending where condition > 3 && r.HostelNumber== 2 select r ;
foreach (Student name in lstStudentName)
Console.WriteLine(name.RollNumber + name.Name);
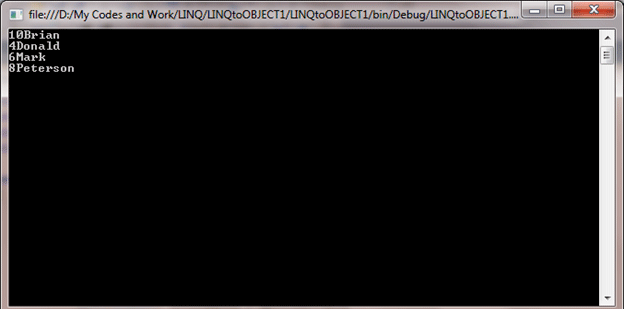
Conclusion
In the next article on this topic, I will explain Grouping using LINQ. I hope this article was useful to you in helping you understand some of the features of LINQ technology. Thanks for reading!