First the output, Home will look like below.
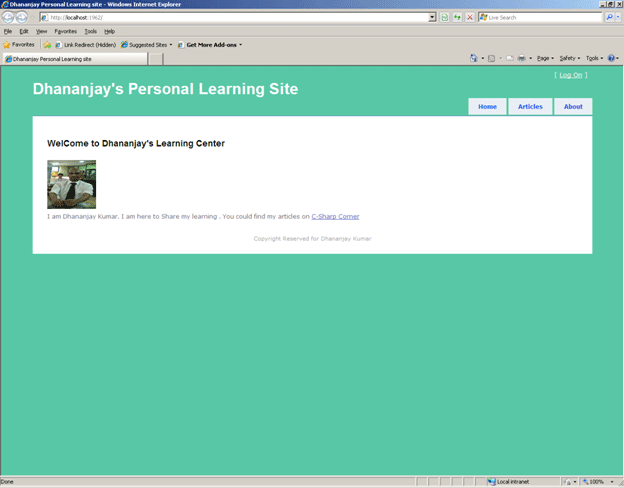
When user will select link to open a particular word document, output would be
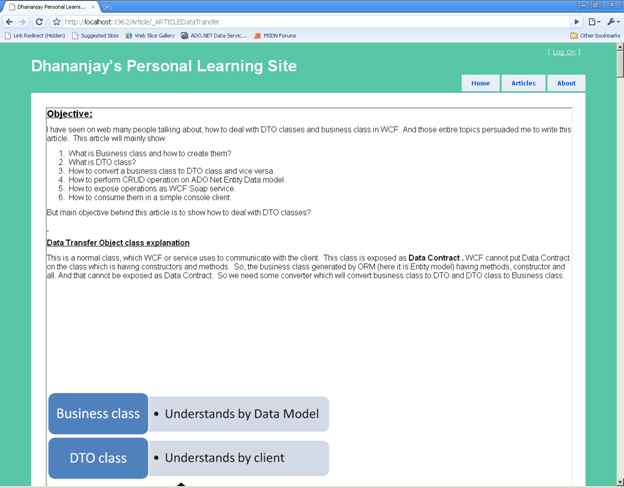
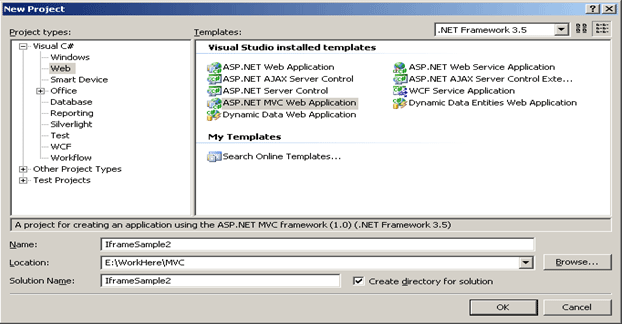
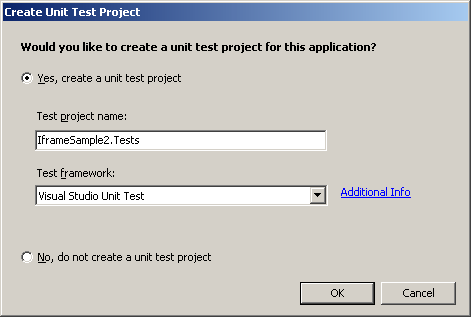
Step 2:
Click on Views->Shared->site.master and add the title and the footer. I have modified the code as below. Add one more ActionLink for the Article. The code which, I have added is in red rectangle in below code.
<body>
<div class="page">
-
We will create individual action for each individual article. What I mean to say here is for each article, there would be a corresponding action in Article Controller.
-
Index action will list all the articles.
-
Right click and add a new folder in project. Give any name of your choice. I am giving name here Articles Repository
-
Suppose , you want to publish article , DTO.doc
-
Open the document.
-
Save as the document as
html filtered type in a separate folder. I have created a folder called Data Transfer.
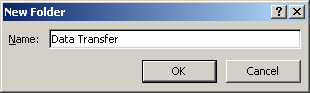
You need to convert all your word documents to type web page document to display it on asp.net MVC site.
-
Copy the folder where you saved html file of your word document and paste it inside
Article Repository folder. So now if you will see solution explore you have something like below image
-
After saving the word document as web page type, it is time to add an Action in Article controller.
-
First we will add a controller called "Article".
-
Right click at controller folder in solution explorer and select Add Controller.
-
Change
Default1 to
Article. Don't check the checkbox and click on
Add. After clicking Add , code will generated as below
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Mvc.Ajax;
namespace IframeSample2.Controllers
{
public class ArticleController : Controller
{
public ActionResult Index()
{
return View();
}
}
}
-
Now we will create a View for Index Controller. So to do this right click at
Index action and select
Add View. This will add a view for Index action of Article controller.
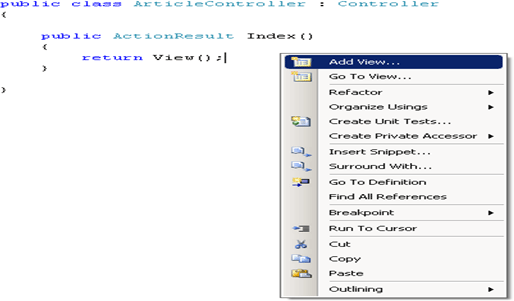
-
Go to View the Article and then Index.aspx. And modify the code as below.
<%@ Page Title="" Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="System.Web.Mvc.ViewPage" %>
<asp:Content ID="Content1" ContentPlaceHolderID="TitleContent" runat="server">
Dhananjay Personal Learning site
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="MainContent" runat="server">
<h1>Article List</h1>
</asp:Content>
-
Press F5 to run. Up to this step expected output on clicking of Article tab is as below
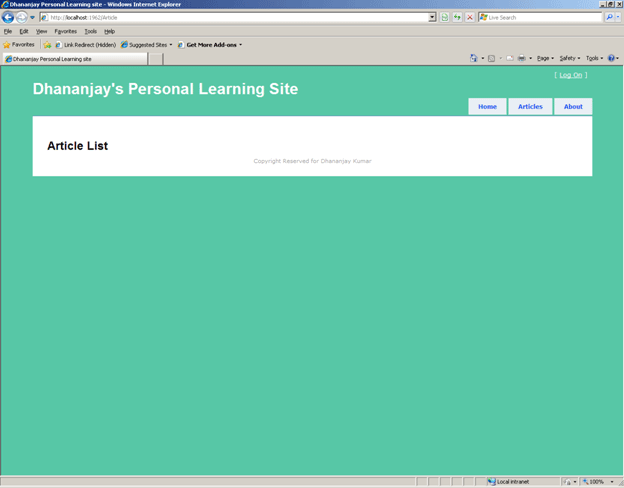
-
Now we will be adding actions to display all the documents. So modify the Article controller as below,
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Mvc.Ajax;
namespace IframeSample2.Controllers
{
public class ArticleController : Controller
{
public ActionResult Index()
{
return View();
}
public ActionResult _ARTICLEDataTransfer()
{
return View();
}
public ActionResult _ARTICLERestService()
{
return View();
}
}
}
Since, I am going to have only three articles as of now, so there are only three actions are there.
-
Now add View for each action. So to do so just right click at each action and select Add View. After creating views for each action three aspx file will get created inside View/Article folder.
-
All the view for document will have the same code. Only one difference would be source for the
Iframe.
Java Script for resizing of Iframe
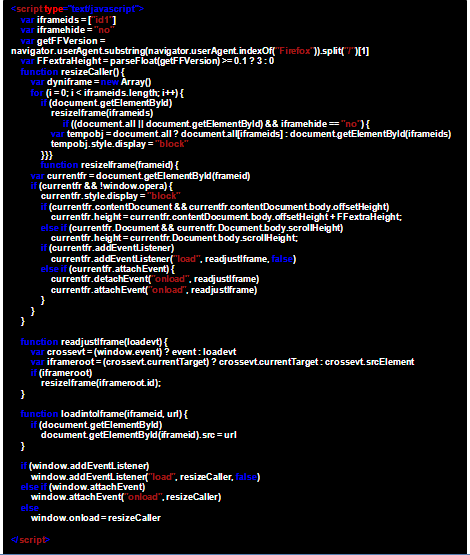
I will use the same java script in all view of documents.
-
Go to View/Article and open _ARTICLEDataTransfer.aspx.
-
Modify the code for VIEW as below,
-
ARTICLEDataTransfer.aspx.
<%@ Page Title="" Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="System.Web.Mvc.ViewPage" %>
<asp:Content ID="Content1" ContentPlaceHolderID="TitleContent" runat="server">
Dhananjay Personal Learning Site
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="MainContent" runat="server">
<script type="text/javascript">
var iframeids = ["id1"]
var iframehide = "no"
var getFFVersion = navigator.userAgent.substring(navigator.userAgent.indexOf("Firefox")).split("/")[1]
var FFextraHeight = parseFloat(getFFVersion) >= 0.1 ? 3 : 0
function resizeCaller() {
var dyniframe = new Array()
for (i = 0; i < iframeids.length; i++) {
if (document.getElementById)
resizeIframe(iframeids)
if ((document.all || document.getElementById) && iframehide == "no") {
var tempobj = document.all ? document.all[iframeids] : document.getElementById(iframeids)
tempobj.style.display = "block"
}
}
}
function resizeIframe(frameid) {
var currentfr = document.getElementById(frameid)
if (currentfr && !window.opera) {
currentfr.style.display = "block"
if (currentfr.contentDocument && currentfr.contentDocument.body.offsetHeight)
currentfr.height = currentfr.contentDocument.body.offsetHeight + FFextraHeight;
else if (currentfr.Document && currentfr.Document.body.scrollHeight)
currentfr.height = currentfr.Document.body.scrollHeight;
if (currentfr.addEventListener)
currentfr.addEventListener("load", readjustIframe, false)
else if (currentfr.attachEvent) {
currentfr.detachEvent("onload", readjustIframe)
currentfr.attachEvent("onload", readjustIframe)
}
}
}
function readjustIframe(loadevt) {
var crossevt = (window.event) ? event : loadevt
var iframeroot = (crossevt.currentTarget) ? crossevt.currentTarget : crossevt.srcElement
if (iframeroot)
resizeIframe(iframeroot.id);
}
function loadintoIframe(iframeid, url) {
if (document.getElementById)
document.getElementById(iframeid).src = url
}
if (window.addEventListener)
window.addEventListener("load", resizeCaller, false)
else if (window.attachEvent)
window.attachEvent("onload", resizeCaller)
else
window.onload = resizeCaller
</script>
<iframe id="id1" src="../../Articles Repository/Data Transfer/DTO.htm" name="I1" scrolling="no" height="100%" width="100%" marginwidth ="0" marginheight="0" />
<h2>_ARTICLEDataTransfer</h2>
</asp:Content>
Explanation of code
-
Java script is same as in all view for the Action, which is used to display the document.
-
To select source of iframe, follow the steps depicted in image below.
-
Select Pick URL then browse to directory and select the HTML file.
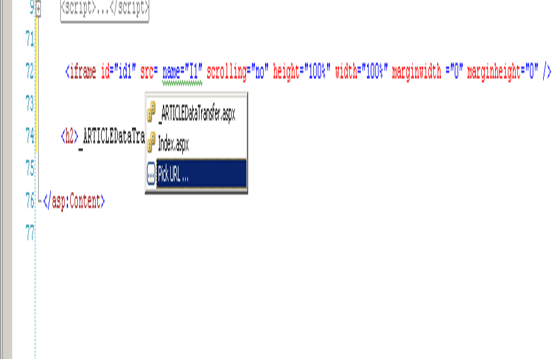
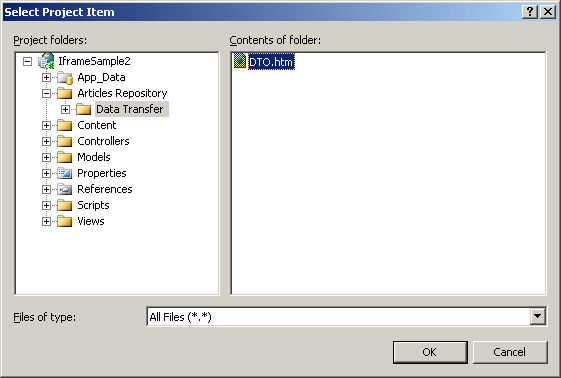
So, the Article controller looks like now,
ArticleController.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Mvc.Ajax;
namespace IframeSample2.Controllers
{
public class ArticleController : Controller
{
public ActionResult Index()
{
return View();
}
public ActionResult _ARTICLEDataTransfer()
{
return View();
}
public ActionResult _ARTICLERestService()
{
return View();
}
}
}
For your reference, codes for the entire aspx file is as below. You could download the zip file also for code reference.
_ARTICLERestService.aspx
<%@ Page Title="" Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="System.Web.Mvc.ViewPage" %>
<asp:Content ID="Content1" ContentPlaceHolderID="TitleContent" runat="server">
Dhananjay Personal Learning site
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="MainContent" runat="server">
<script type="text/javascript">
var iframeids = ["id1"]
var iframehide = "no"
var getFFVersion = navigator.userAgent.substring(navigator.userAgent.indexOf("Firefox")).split("/")[1]
var FFextraHeight = parseFloat(getFFVersion) >= 0.1 ? 3 : 0 //extra height in px to add to iframe
function resizeCaller() {
var dyniframe = new Array()
for (i = 0; i < iframeids.length; i++) {
if (document.getElementById)
resizeIframe(iframeids)
if ((document.all || document.getElementById) && iframehide == "no") {
var tempobj = document.all ? document.all[iframeids] : document.getElementById(iframeids)
tempobj.style.display = "block"
}
}
}
function resizeIframe(frameid) {
var currentfr = document.getElementById(frameid)
if (currentfr && !window.opera) {
currentfr.style.display = "block"
if (currentfr.contentDocument && currentfr.contentDocument.body.offsetHeight) currentfr.height =
currentfr.contentDocument.body.offsetHeight + FFextraHeight;
else if (currentfr.Document && currentfr.Document.body.scrollHeight)
currentfr.height = currentfr.Document.body.scrollHeight;
if (currentfr.addEventListener)
currentfr.addEventListener("load", readjustIframe, false)
else if (currentfr.attachEvent) {
currentfr.detachEvent("onload", readjustIframe)
currentfr.attachEvent("onload", readjustIframe)
}
}
}
function readjustIframe(loadevt) {
var crossevt = (window.event) ? event : loadevt
var iframeroot = (crossevt.currentTarget) ? crossevt.currentTarget : crossevt.srcElement
if (iframeroot)
resizeIframe(iframeroot.id);
}
function loadintoIframe(iframeid, url) {
if (document.getElementById)
document.getElementById(iframeid).src = url
}
if (window.addEventListener)
window.addEventListener("load", resizeCaller, false)
else if (window.attachEvent)
window.attachEvent("onload", resizeCaller)
else
window.onload = resizeCaller
</script>
<iframe id="id1" src="../../Articles Repository/rest/IsolatedStorage.htm" name="I1" scrolling="no" height="100%" width="100%" marginwidth ="0" marginheight="0" />
</asp:Content>
-
Modify the view of Index action as follows
/Article/Index.aspx
<%@ Page Title="" Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="System.Web.Mvc.ViewPage" %>
<asp:Content ID="Content1" ContentPlaceHolderID="TitleContent" runat="server">
Dhananjay Personal Learning site
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="MainContent" runat="server">
<h1>Article List</h1>
<h3> This Article will expalin about Business object and Data Transfer Object in WCF Click </h3> <%= Html.ActionLink("DTO in WCF", "_ARTICLEDataTransfer", "Article")%> to read more<br />
<h3> This Article will expalin about Isolated Storage in SilverLight Click </h3><%= Html.ActionLink("Isolated Storage in SilverLight", "_ARTICLERestService", "Article")%>
</asp:Content>
-
Now modify the Home/Index.aspx as below
Home/Index.aspx
<%@ Page Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="System.Web.Mvc.ViewPage" %>
<asp:Content ID="indexTitle" ContentPlaceHolderID="TitleContent" runat="server">
Dhananjay Personal Learning site
</asp:Content>
<asp:Content ID="indexContent" ContentPlaceHolderID="MainContent" runat="server">
<h2> WelCome to Dhananjay's Learning Center</h2>
<p>
<img src="../../D.jpg" width="100" height="100" alt="Dhananjay Kumar"/><br />
I am Dhananjay Kumar. I am here to Share my learning . You could find my articles on
<a href="http://www.c-sharpcorner.com/Authors/AuthorDetails.aspx?AuthorID=dhananjaycoder" title="Dhananjay's article "> C-Sharp Corner</a>
</p>
</asp:Content>