Objective
This article will show how to iterate through SharePoint document library and list out all the file names and bind the list to a drop down list.
Working Screen
When user will click on button name of the files will get loaded from document library to drop down list.
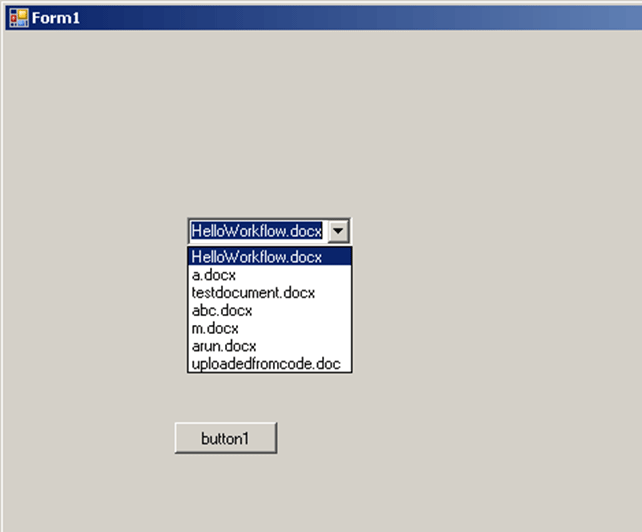
Step 1
Create a window application. Drag and drop one Button and DropDown list.
Step 2
Add reference of Windows SharePoint Services
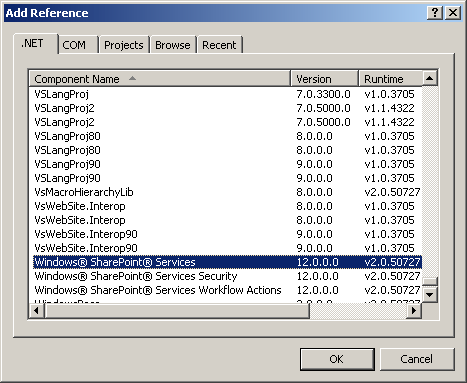
Step 3
On click event of button write the below code
private void button1_Click(object sender, EventArgs e)
{
listName = new List<string>();
using (SPSite site = new SPSite("http://adfsaccount:2222/"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["My Documents"];
SPListItemCollection items = list.Items;
foreach (SPListItem item in items)
{
//MessageBox.Show(item.Name);
listName.Add(item.Name);
}
}
}
comboBox1.DataSource = listName;
}
Explanation
-
Returning SharePoint site using SPSite.
-
Returning current web using SPWeb.
-
My Documents is name of the document library.
-
We are iterating through the document library and fetching all the file names and adding them to a string list.
Full Code
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Microsoft.SharePoint;
namespace WindowsFormsApplication2
{
public partial class Form1 : Form
{
List<string> listName = null;
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
listName = new List<string>();
using (SPSite site = new SPSite("http://adfsaccount:2222/"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["My Documents"];
SPListItemCollection items = list.Items;
foreach (SPListItem item in items)
{
listName.Add(item.Name);
}
}
}
comboBox1.DataSource = listName;
}
}
}
Output
Conclusion
I have shown in this article, How to iterate through the Document Library. Thanks for reading.