Objective:
In this article, I am going to show how we could work with SharePoint items using object model or in other words using .Net code. I will show you
- How to add item into the SharePoint list?
- How to retrieve item from the SharePoint list?
- How to update item into the SharePoint list?
- How to delete item from the SharePoint list?
Assumption:
- I have created a custom list called Reader.
- There are two columns. One is Reader'sName. This is a single line text column. Second is Reader'sArticle. This is numeric column.
- I have put some item also in Reader list.
In SharePoint site, Reader list look like as below,
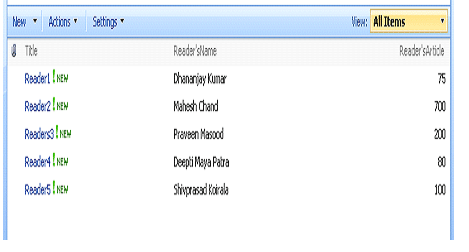
Here, I assume you know how to create a custom list in a SharePoint site and how to put some data into that. If you do not know, read my article on the same here on this site. Now I am going to manipulate the above list through code.
I am going to follow the below steps.
- Create a window application
- Add reference of Microsoft.SharePoint dll.
- Add few controls like buttons and text boxes. I am a bad UI designer. So don't follow my window form here. You design of yours.
- Return a site collection using SPSite.
- Return particular website where list is added using SPWeb.
- Return collection of list items using SPListItemCollection.
- Work with a particular list item using SPListItem.
Adding Microsoft.SharePoint dll
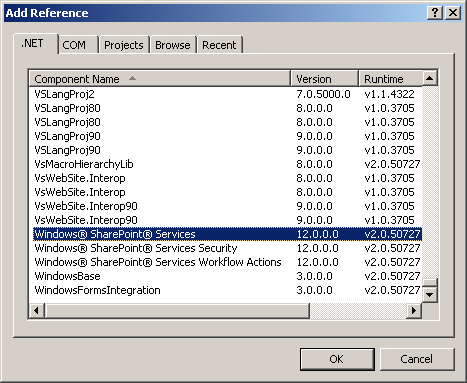
Design of the Form
- There are two text boxes.
- Three buttons to add, delete and update.
Explanation
- I am returning the site collection on the form load.
- I am returning the top level site also on form load.
Adding to the List
- Readers is name of the list
- I am returning the List collection and adding the item into that.
- I am reading the items to be added from the textboxes.
private void btnAdd_Click(object sender, EventArgs e)
{
string Name = txtName.Text;
int Numofarticle = Convert.ToInt32(txtNumberofArticle.Text);
_ItemCollection = _MyWeb.Lists["Readers"].Items ;
_MyItem = _ItemCollection.Add();
_MyItem["Reader'sName"] = Name;
_MyItem["Reader'sArticle"] = Numofarticle;
_MyItem.Update();
txtName.Text = "";
txtNumberofArticle.Text = "";
MessageBox.Show("Item Added");
}
Updating to the List
-
Readers is name of the list
-
I am returning the List collection and updating the item into that.
-
I am reading the items to be added from the textboxes.
-
I am iterating through the collection and searching for the Reader'sName to be updated.
private void btnEdit_Click(object sender, EventArgs e)
{
_ItemCollection = _MyWeb.Lists["Readers"].Items;
int count = _ItemCollection.Count;
for (int i = 0; i < count; i++)
{
SPListItem item = _ItemCollection[i];
if (item["Reader'sName"].ToString() == txtName.Text.ToString())
{
item["Reader'sArticle"] = Convert.ToInt32(txtNumberofArticle.Text);
item.Update();
}
}
MessageBox.Show("Updated");
}
}
Deleting from the List
-
Readers is name of the list
-
I am returning the List collection and updating the item into that.
-
I am reading the items to be added from the textboxes.
-
I am iterating through the collection and searching for the Reader'sName to be deleted
private void btnDelete_Click(object sender, EventArgs e)
{
_ItemCollection = _MyWeb.Lists["Readers"].Items;
int count = _ItemCollection.Count;
for (int i = 0; i < count; i++)
{
SPListItem item = _ItemCollection[i];
if (item["Reader'sName"].ToString() == txtName.Text.ToString())
{
_ItemCollection.Delete(i);
count--;
}
}
MessageBox.Show("Deleted");
}
For reference the whole code is as below,
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Microsoft.SharePoint;
namespace ProgrammingList
{
public partial class Form1 : Form
{
SPSite _MySite = null;
SPWeb _MyWeb = null;
SPListItemCollection _ItemCollection = null;
SPListItem _MyItem = null;
public Form1()
{
InitializeComponent();
}
private void btnAdd_Click(object sender, EventArgs e)
{
MessageBox.Show(_MyWeb.Title);
string Name = txtName.Text;
int Numofarticle = Convert.ToInt32(txtNumberofArticle.Text);
_ItemCollection = _MyWeb.Lists["Readers"].Items ;
_MyItem = _ItemCollection.Add();
_MyItem["Reader'sName"] = Name;
_MyItem["Reader'sArticle"] = Numofarticle;
_MyItem.Update();
txtName.Text = "";
txtNumberofArticle.Text = "";
MessageBox.Show("Item Added");
}
private void Form1_Load(object sender, EventArgs e)
{
_MySite = new SPSite("http://adfsaccount:2222/");
// _MyWeb = _MySite.AllWebs["Document Center"];
_MyWeb =_MySite.OpenWeb();
}
private void btnDelete_Click(object sender, EventArgs e)
{
_ItemCollection = _MyWeb.Lists["Readers"].Items;
int count = _ItemCollection.Count;
for (int i = 0; i < count; i++)
{
SPListItem item = _ItemCollection[i];
if (item["Reader'sName"].ToString() == txtName.Text.ToString())
{
_ItemCollection.Delete(i);
count--;
}
}
MessageBox.Show("Deleted");
}
private void btnEdit_Click(object sender, EventArgs e)
{
_ItemCollection = _MyWeb.Lists["Readers"].Items;
int count = _ItemCollection.Count;
for (int i = 0; i < count; i++)
{
SPListItem item = _ItemCollection[i];
if (item["Reader'sName"].ToString() == txtName.Text.ToString())
{
item["Reader'sArticle"] = Convert.ToInt32(txtNumberofArticle.Text);
item.Update();
}
}
MessageBox.Show("Updated");
}
}
}
Conclusion:
In this article, I showed how to manipulate SharePoint list using code. Thanks for reading.
Happy Coding