Introduction
There were many requirements where we need to pass the value from ChildWindow to our Parent Window in Silverlight. In this article we will see a sample implementation of this requirement to pass the value from the ChildWindow to the Parent Window.
This is the idea what we are going to do. We will select a date from the calendar from the ChildWindow and pass the selected value to the Parent Window and display it.
Creating Silverlight Project
Fire up Visual Studio 2008 and create a new Silverlight 3 Project. Name it as ChildToParent.
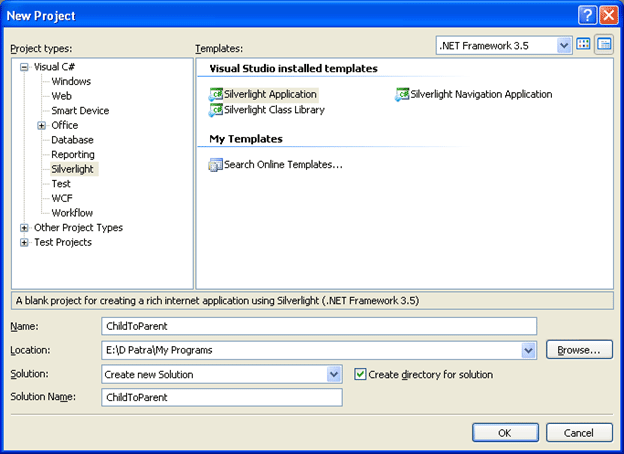
Now first things first add a ChildWindow to the Silverlight Project name the ChildWindow as DatePopUpWindow.
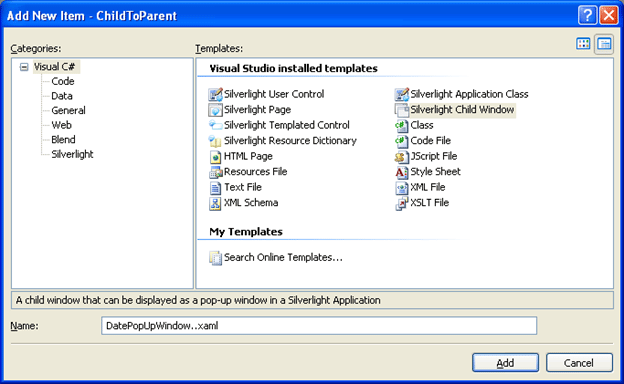
Now that we have added everything needed we will design our page in Blend as follows:
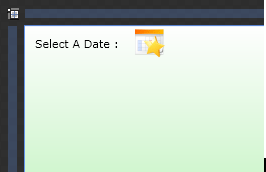
I have added one TextBlock to display the Selected Date and one Image; on it's click the ChildWindow will be fired up.
<TextBlock HorizontalAlignment="Left" VerticalAlignment="Top" Text="Select A Date : " TextWrapping="Wrap" Margin="10,10,0,0"/>
<TextBlock x:Name="txtDate" HorizontalAlignment="Left" VerticalAlignment="Top" TextWrapping="Wrap" Margin="10,30,0,0"/>
<Image x:Name="imgCalendar" Height="32" HorizontalAlignment="Left" VerticalAlignment="Top" Width="32" Margin="108,0,0,0" Source="Images/calendar.png" MouseLeftButtonDown="imgCalendar_MouseLeftButtonDown"/>
Now we will design our Childwindow as follows:
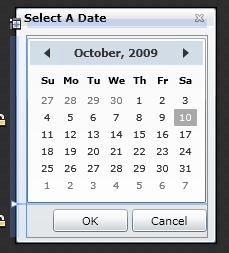
As you see from the above image; I have added a calendar to the ChildWindow.
<controls:Calendar x:Name="myCalendar" HorizontalAlignment="Left" d:LayoutOverrides="Height"/>
|<Button x:Name="CancelButton" Content="Cancel" Click="CancelButton_Click" Width="75" Height="23" HorizontalAlignment="Right" Margin="0" VerticalAlignment="Center" Grid.Row="1" d:LayoutOverrides="Height" />
<Button x:Name="OKButton" Content="OK" Click="OKButton_Click" Width="75" Height="23" HorizontalAlignment="Right" Margin="0,0,79,0" VerticalAlignment="Center" Grid.Row="1" d:LayoutOverrides="Height" />
Now go back to the VisualStudio IDE and open DatePopUpWindow.xaml.cs
Here we will add a event handler.
public event EventHandler OkClicked;
Add a property that can be accessed from the main window.
private DateTime _SelectedDate;
public DateTime SelectedDate
{
get { return _SelectedDate; }
set { _SelectedDate = value; }
}
Now in the OkButton_Click event add the following code:
private void OKButton_Click(object sender, RoutedEventArgs e)
{
if (OkClicked!=null)
{
_SelectedDate = (DateTime)myCalendar.SelectedDate;
OkClicked(this, new EventArgs());
}
this.DialogResult = true;
}
Now go back to the MainPage.xaml.cs and create an instance of ChildWindow:
DatePopUpWindow calendar;
In the click event of the Image (Calendar) display the ChildWindow and Initiate the Event.
private void imgCalendar_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
calendar = new DatePopUpWindow();
calendar.Show();
calendar.OkClicked += new EventHandler(cw_OkClicked);
}
In the event handler add the following code to access the data from ChildWindow.
void cw_OkClicked(object sender, EventArgs e)
{
txtDate.Text = "You have selected : " + calendar.SelectedDate.ToShortDateString();
}
That's it. We are done. Now run your application.
When you click on the Calendar Image the ChildWindow will be displayed.
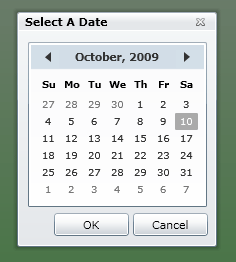
Select any date and press ok.
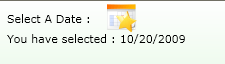
Hope you like this simple article.