Introduction:
Basic intention of this article is to teach you how to build simple generic classes by our own. Like List<T>,Dictionary <T> ,Stack<T>are inbuilt Generic Classes .in this article we will create our own Stack<T> Generic Class like the provided in .net .that can Work with Any Data Types like String , Object ,Double ,etc to store.
Technologies
.net framework 2.0/3.5
Language
C#
Prerequisites
Basic Knowledge of Array Operations, Class, Constructor
Implementation
Before creating Stack<T> we will see what is Stack. Stack is linear data structure in which we can store/ delete element from only one end. It can have Tree Operations basically PUSH, POP, PEEP.
As real life example if we have so many dishes which are laying on one another the man will take only dish which is on the top of all dishes. Same like that scenario stack is working in which if we put new element that can be on top position only or if we remove element it can be on top only.
Here one variable TOP is maintained .top indicates.Last element in stack insertion of element and deletion of element can be done with the top position of stack.
Push Operation: Store element at top position of stack;
POP operation: Return the Element at top and decrement top by 1
PEEP Operation: return element at position specified by user
So let's start we make out Own Stack<T> Structure.That can store any data type element s in stack Fashion
Below is basic outline what methods we are going to develop in Stack<T> class
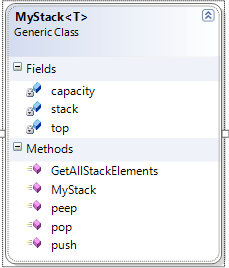
class MyStack<T>
{
int capacity;
T[] stack;
int top;
public MyStack(int MaxElements)
{
capacity = MaxElements;
stack = new T[capacity];
//initialize top with -1
}
public int push(T Element)
{
//Check Overflow
if (top == capacity - 1)
{
// return -1 if over flow is there
return -1;
}
else
{
// insert elementt into stack
top = top + 1;
stack[top] = Element;
}
return 0;
}
public T pop()
{
T RemovedElement;
T temp = default(T);
//check Underflow
if(!(top<=0))
{
RemovedElement = stack[top];
top = top-1;
return RemovedElement;
}
return temp;
}
public T peep(int position)
{
T temp = default(T);
//check if Position is Valid or not
if (position < capacity && position >= 0)
{
return stack[position];
}
return temp;
}
public T[] GetAllStackElements()
{
T[] Elements = new T[top+1];
Array.Copy(stack,0,Elements,0,top+1);
return Elements;
}
}
Now we can implement our Class MyStack<T> that we have coded in program...Like below...
static void Main(string[] args)
{
int capacity;
Console.WriteLine("Enter Capacity of Stack :");
capacity = int.Parse(Console.ReadLine());
MyStack<string> stack = new MyStack<string>(10);
while (true)
{
Console.WriteLine("1.Push");
Console.WriteLine("2.Pop");
Console.WriteLine("3.Peep");
Console.WriteLine("4.Print Stack Elements:");
Console.WriteLine("5.Exit");
Console.WriteLine("Eneter your Choice :");
int choice = int.Parse(Console.ReadLine());
switch (choice)
{
case 1:
{
Console.WriteLine("Enter String to Push :");
string temp = Console.ReadLine();
int result = stack.push(temp);
if ( result != -1)
{
Console.WriteLine("Element Pushed into Stack !");
}
else
{
Console.WriteLine("Stack Overflow !");
}
break;
}
case 2:
{
string Result = stack.pop();
if(Result != null)
{
Console.WriteLine("Delete Element :"+Result);
}
else
{
Console.WriteLine("Stack Underflow !");
}
break;
}
case 3:
{
Console.WriteLine("Enter Position of Element to Pop:");
int Position = int.Parse(Console.ReadLine());
string Result = stack.peep(Position);
if(Result != null)
{
Console.WriteLine("Element at Position"+Position+ " is "+Result);
}
else
{
Console.WriteLine("Entered Element is Out of Stack Range ");
}
break;
}
case 4:
{
string[] Elements = stack.GetAllStackElements();
Console.WriteLine("**************Stack Content **************");
foreach (string str in Elements)
{
Console.WriteLine(str);
}
break;
}
case 5:
{
System.Diagnostics.Process.GetCurrentProcess().Kill();
break;
}
default:
{
Console.WriteLine("You have Entered Wrong Choice ");
break;
}
}
}
}
Conclusion
This article explained how to create our own Stack<T> class using Generics.