Step 1: In this example, there are three TextBoxes (txtid, txtfname, txtsubject) and a Button (btnshow). When we click on the Button we can get the value from the TextBoxes and show it in a line with the help of a <p> tag (pid, pname, psubject).
Id: <input id="txtid" runat="server" type="text" />
<br />
Name: <input id="txtfname" runat="server" type="text" />
<br />
Subject: <input id="txtsubject" type="text" runat="server"/>
<br />
<br />
<input id="btnshow" type="button" onclick="ShowText()" value="Show" />
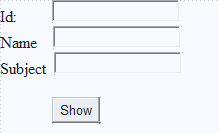
Step 2: When we click on the button (btnshow), it calls a function called ShowText( ), in this Function:
var xmlHttp
var arr;
function ShowText() {
xmlHttp = GetXmlHttpObject()
var url = "Default.aspx"
url = url + "?id=" + document.getElementById('txtid').value
xmlHttp.onreadystatechange = stateChanged
xmlHttp.open("GET", url, true)
xmlHttp.send(null)
return false;
}
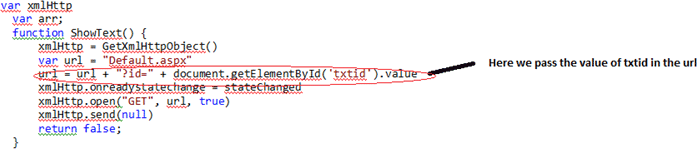
Now we can write the GetXmlHttpObject() to check the browser:
function GetXmlHttpObject() {
var objXMLHttp = null
if (window.XMLHttpRequest) {
objXMLHttp = new XMLHttpRequest()
}
else if (window.ActiveXObject) {
objXMLHttp = new ActiveXObject("Microsoft.XMLHTTP")
}
return objXMLHttp
}
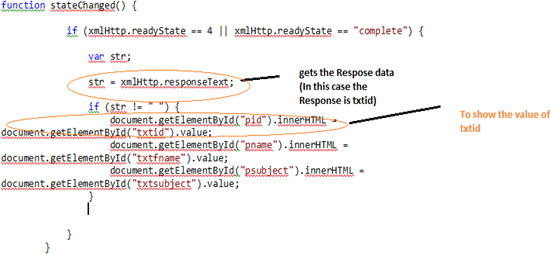
Step 3: In the Page_Load Event
if (!IsPostBack)
{
if (Request.QueryString["id"] != null)
{
string str = txtid.Value;
Response.Clear();
Response.Write(str);
Response.End();
}to show the value
}
It sets the value of txtid in str. Now we can look at this.
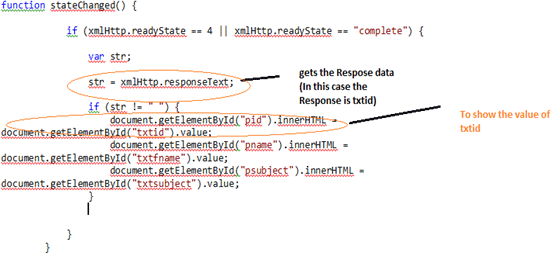
Here the response Text has the value of txtid.
In Default.aspx:
<head runat="server">
<title></title>
<script language="JavaScript" type="text/javascript" >
var xmlHttp
var arr;
function ShowText() {
xmlHttp = GetXmlHttpObject()
var url = "Default.aspx"
url = url + "?id=" + document.getElementById('txtid').value
xmlHttp.onreadystatechange = stateChanged
xmlHttp.open("GET", url, true)
xmlHttp.send(null)
return false;
}
function stateChanged() {
if (xmlHttp.readyState == 4 || xmlHttp.readyState == "complete") {
var str;
str = xmlHttp.responseText;
if (str != " ") {
document.getElementById("pid").innerHTML = document.getElementById("txtid").value;
document.getElementById("pname").innerHTML = document.getElementById("txtfname").value;
document.getElementById("psubject").innerHTML = document.getElementById("txtsubject").value;
}
}
}
function GetXmlHttpObject() {
var objXMLHttp = null
if (window.XMLHttpRequest) {
objXMLHttp = new XMLHttpRequest()
}
else if (window.ActiveXObject) {
objXMLHttp = new ActiveXObject("Microsoft.XMLHTTP")
}
return objXMLHttp
}
</script>
<style type="text/css">
#pid
{
height: 11px;
width: 43px;
}
</style>
</head>
<body>
<form id="form1" runat="server">
Id:
<input id="txtid" runat="server" type="text" />
<br />
Name
<input id="txtfname" runat="server" type="text" />
<br />
Subject
<input id="txtsubject" type="text" runat="server"/>
<br />
<br />
<input id="btnshow" type="button" onclick="ShowText()" value="Show" />
<br />
<br />
<br />
Your Id<p id="pid"></p>
Name: <p id="pname"></p> Subject: <p id="psubject"></p> </form>
</body>
</html>
In Default.aspx.cs
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
if (Request.QueryString["id"] != null)
{
string str = txtid.Value;
Response.Clear();
Response.Write(str);
Response.End();
}
}