Adding Child Controls to a ComboBox
I am building a Project Survival Forecaster application in Silverlight 4 that forecasts the chances of surviving a software project. In this application, I need a ComboBox control to host other child controls.
This article demonstrates how to host various child controls within a ComboBox control in Silverlight.
If you are new to Silverlight ComboBox, I recommed you read my previous tutorial:
ComboBox in Silverlight
Adding child controls to a ComboBox is similar to adding any item to a ComboBox. The ComboBox.Items represents a collection of items of a ComboBox. We can use ComboBox.Items.Add() method to add an object to a ComboBox. This object item can be a text, control or any other object.
The code snippet in Listing 1 adds a string, a Button, a TextBlock, a DateTime, a Rectangle, and a Panel with child controls to a ComboBox.
// Add a String
comboBox1.Items.Add("ComboBox with Child Controls");
// Add a Button
Button btn = new Button();
btn.Height = 50;
btn.Width = 150;
btn.Content = "Click ME";
btn.Background = new SolidColorBrush(Colors.Orange);
btn.Foreground = new SolidColorBrush(Colors.Black);
comboBox1.Items.Add(btn);
// Create a TextBlock and Add it to ComboBox
TextBlock textBlockItem = new TextBlock();
textBlockItem.TextAlignment = TextAlignment.Center;
textBlockItem.FontFamily = new FontFamily("Georgia");
textBlockItem.FontSize = 14;
textBlockItem.FontWeight = FontWeights.ExtraBold;
textBlockItem.Text = "Hello! I am a text block.";
// Add TextBlock to ComboBox
comboBox1.Items.Add(textBlockItem);
// Add a DateTime to a ComboBox
DateTime dateTime1 = new DateTime(2010, 10, 12, 8, 15, 55);
comboBox1.Items.Add(dateTime1);
// Add a Rectangle to a ComboBox
Rectangle rect1 = new Rectangle();
rect1.Width = 50;
rect1.Height = 50;
rect1.Fill = new SolidColorBrush(Colors.Red);
comboBox1.Items.Add(rect1);
// Add a panel that contains child controls
TextBlock textBlock1 = new TextBlock();
textBlock1.TextAlignment = TextAlignment.Center;
textBlock1.Text = "Panel with child controls";
Ellipse ellipse1 = new Ellipse();
ellipse1.Width = 50;
ellipse1.Height = 50;
ellipse1.Fill = new SolidColorBrush(Colors.Green);
StackPanel comboBoxPanel = new StackPanel();
comboBoxPanel.Width = 200;
comboBoxPanel.Background = new SolidColorBrush(Colors.Yellow);
comboBoxPanel.Children.Add(textBlock1);
comboBoxPanel.Children.Add(ellipse1);
// Add Panel to ComboBox
comboBox1.Items.Add(comboBoxPanel);
comboBox1.SelectedIndex = 0;
comboBox1.Padding = new Thickness(0);
comboBox1.MaxWidth = 200;
Listing 1
The output of Listing 1 looks like Figure 1.
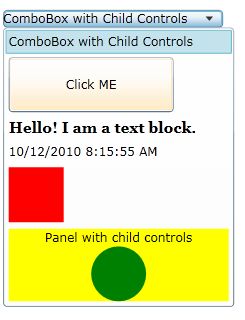
Figure 1
Further Readings
I recommend reading these articles: