This article has been excerpted from book "Graphics Programming with GDI+".
There are several ways to create a Rectangle object. For example, you can create a Rectangle object from four integer values representing the starting point and size of the rectangle, or from Point and Size structures. Listing 6.1 creates Rectangle objects from Size, Point, and direct values. As this code shows a Rectangle constructor can take a Point and a Size object or, alternatively, the starting point (as separate variables x and y), width, and height.
LISTING 6.1: Constructing Rectangle objects
int x = 20;
int y = 30;
int height = 30;
int width = 30;
//Create a starting point
Point pt = new Point(10, 10);
//Create a size
Size sz = new Size(60, 40);
//Create a rectangle from a point and a size
Rectangle rect1 = new Rectangle(pt, sz);
Rectangle rect2 = new Rectangle(x, y, width, height);
Constructing a RectangleF Object
You can also create a RectangleF object in several ways: from four floating point numbers with the starting and ending points and height and width of the rectangle, or from a point and a size. RectangleF is a mirror of Rectangle, including properties and methods. The only difference is that RectangleF takes floating point values. For example, instead of Size and Point, RectangleF uses SizeF and PointF. Listing 6.2 creates RectangleF object in two different ways.
LISTING 6.2: Constructing RectangleF objects
//Create a starting point
PointF pt = new PointF(30.08f, 20.7f);
//Create a Size
SizeF sz = new SizeF(60.0f, 40.0f);
//Create a rectangle from a point and a size
RectangleF rect1 = new RectangleF(pt, sz);
//Create a rectangle from floating points
RectangleF rect2 = new RectangleF(40.2f, 40.6f, 100.5f, 100.0f);
Rectangle Properties and Methods
The Rectangle structure provides properties that include Bottom, Top, Left, Right, Height, Width, IsEmpty, Location, Size, X, and Y. Listing 6.3 create two rectangle (rect1 and rect2), reads these properties, and displays their values in a message box.
LISTING 6.3: Using the Rectangle Structure properties
private void PropertiesMenu_Click(object sender, System.EventArgs e)
{
//Create a point
PointF pt = new PointF(30.8f, 20.7f);
//Create a Size
SizeF sz = new SizeF(60.0f, 40.0f);
//Create rectangle from a point and
//a size
RectangleF rect1 = new RectangleF(pt, sz);
RectangleF rect2 =
new RectangleF(40.2f, 40.6f, 100.5f, 100.0f);
//If rectangle is empty
//set its Location, Width, and Height properties
if (rect1.IsEmpty)
{
rect1.Location = pt;
rect1.Width = sz.Width;
rect1.Height = sz.Height;
}
//Read properties and display
string str = "Location:" + rect1.Location.ToString();
str += "X:" + rect1.X.ToString() + "\n";
str += "Y:" + rect1.Y.ToString() + "\n";
str += "Left:" + rect1.Left.ToString() + "\n";
str += "Right:" + rect1.Right.ToString() + "\n";
str += "Top:" + rect1.Top.ToString() + "\n";
str += "Bottom:" + rect1.Bottom.ToString() + "\n";
MessageBox.Show(str);
}
The Rectangle structure provides methods that include Round, Truncate, Inflate, Ceiling, Intersect, and Union.
- The Round method converts a RectangleF object to a Rectangle object by rounding off the values of RectangleF to the nearest integer.
- The Truncate method converts a RectangleF object to a Rectangle object by truncating the values of RectangleF.
- The Inflate method creates a rectangle inflated by the specified amount.
- The Ceiling method converts a RectangleF object to a Rectangle object by rounding to the next higher integer values.
- The Intersect method replaces a rectangle by its intersection with a supplied rectangle.
- The Union method gets a rectangle that contains the union of two rectangles.
Listing 6.4 shows how to use the Round, Truncate, Inflate, Ceiling, Intersect, and Union methods.
LISTING 6.4: Using the Rectangle structure methods.
private void MethodsMenu_click (object sender, System.EventArgs e)
{
//Create a Graphics object
Graphics g = this.CreateGraphics();
//Create a point and a size
PointF pt = new PointF(30.08f, 20.7f);
SizeF sz = new SizeF(60.0f, 40.0f);
//Create two rectangles
RectangleF rect1 = new RectangleF(pt, sz);
RectangleF rect2 = new RectangleF(40.2f, 40.6f, 100.5f, 100.0f);
//Ceiling a rectangle
Rectangle rect3 = Rectangle.Ceiling(rect1);
//Truncate a rectangle
Rectangle rect4 = Rectangle.Truncate(rect1);
//Round a rectangle
Rectangle rect5 = Rectangle.Round(rect2);
//Draw rectangles
g.DrawRectangle(Pens.Black, rect3);
g.FillRectangle(Brushes.Red, rect5);
//Intersect a rectangle
Rectangle isectRect =
Rectangle.Intersect(rect3, rect5);
//Fill rectangle
g.FillRectangle(
new SolidBrush(Color.Blue), isectRect);
//Inflate a rectangle
Size inflateSize = new Size(0, 40);
isectRect.Inflate(inflateSize);
//Draw rectanlge
g.DrawRectangle(Pens.Blue, isectRect);
//Empty rectangle and set its properties
rect4 = Rectangle.Empty;
rect4.Location = new Point(50, 50);
rect4.X = 30;
rect4.Y = 40;
//Union rectangles
Rectangle unionRect =
Rectangle.Union(rect4, rect5);
//Draw rectangle
g.DrawRectangle(Pens.Green, unionRect);
//Dispose of objects
g.Dispose();
}
Figure 6.3 shows the output of Listing 6.3
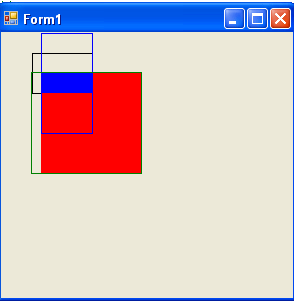
FIGURE 6.3: Using Rectangle methods
Conclusion
Hope the article would have helped you in understanding Constructing a Rectangle Object in GDI+. Read other articles on GDI+ on the website.
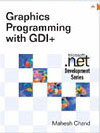 |
This book teaches .NET developers how to work with GDI+ as they develop applications that include graphics, or that interact with monitors or printers. It begins by explaining the difference between GDI and GDI+, and covering the basic concepts of graphics programming in Windows. |