This article has been excerpted from book "Graphics Programming with GDI+".
The Contains method is used to determining whether a rectangle or point is inside the current rectangle. If a point is inside the current rectangle, the Contains method returns true; otherwise it returns false. One of the common uses of Contains is to find out is a mouse button was clicked inside a rectangle.
Hit Test example
To see proper use of the Contains method, let's create a Windows application and draw a rectangle on the form. Whether the user clicks inside or outside of the rectangle, we will have the application generate an appropriate message.
First we define class-level Rectangle variable as follows:
Rectangle bigRect = new Rectangle(50, 50, 100, 100);
Then we use the form's paint event handler because we want to render graphics whenever the form needs to refresh. The form's paint event handler code look like this:
private void Form1_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
SolidBrush brush = new SolidBrush(Color.Green);
e.Graphics.FillRectangle(brush, bigRect);
brush.Dispose();
}
Our last step is to determine whether the user clicked inside the rectangle. We track the user's mouse-down event and write code for the left mouse button click event handler. The MouseEventArgs enumeration provides members to find out which mouse button is clicked. The MouseButtons enumeration has members that include Left, Middle, None, Right, Xbutton1, and Xbutton2, which represent the mouse buttons.
We check to see if the mouse button clicked was the left button, then create a rectangle, and (if the mouse button was the left button, then create a rectangle, and (if the mouse button was clicked) generate a message. Listing 6.5 shows the code for this process.
LISTING 6.5: Determining whether a mouse was clicked inside a rectangle
private void Form1_MouseDown(object sender, System.Windows.Forms.MouseEventArgs e)
{
if (e.Button == MouseButtons.Left)
{
if (bigRect.Contains(new Point(e.X, e.Y)))
MessageBox.Show("Clicked inside rectanlge");
else
MessageBox.Show("Clicked outside rectangle");
}
}
When you run the application and click on the rectangle, the output looks like Figure 6.4.
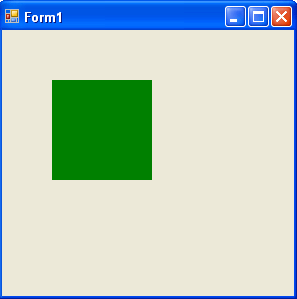
Figure 6.4
The Contains method also allows us to find out whether a rectangle fits inside another rectangle. Listing 6.6 checks whether smallRect is within bigRect.
LISTING 6.6: Checking if one rectangle is within another
Point pt = new Point (0,0);
Size sz = new Size (200,200);
Rectangle bigRect = new Rectangle (pt, sz);
Rectangle smallRect = new Rectangle (30, 20, 100, 100);
if(bigRect.Contains (smallRect))
MessageBox.Show ("Rectangle" + smallRect.ToString() + " is inside Rectangle " + bigRect.ToString());
Conclusion
Hope the article would have helped you in understanding the Contains Method and Hit Test in GDI+. Read other articles on GDI+ on the website.
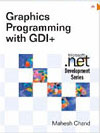 |
This book teaches .NET developers how to work with GDI+ as they develop applications that include graphics, or that interact with monitors or printers. It begins by explaining the difference between GDI and GDI+, and covering the basic concepts of graphics programming in Windows. |