This article has been excerpted from book "The Complete Visual C# Programmer's Guide" from the Authors of C# Corner.
Another very useful class, FileSystemWatcher, acts as a watchdog for file system changes and raises an event when a change occurs. You must specify a directory to be monitored. The class can monitor changes to subdirectories and files within the specified directory. If you have Windows 2000, you can even monitor a remote system for changes. (Only remote machines running Windows NT or Windows 2000 are supported at present.) The option to monitor files with specific extensions can be set using the Filter property of the FileSystemWatcher class. You can also fine-tune FileSystemWatcher to monitor any change in file Attributes, LastAccess, LastWrite, Security, and Size data.
The FileSystemWatcher class raises the events described in Table 6.3.
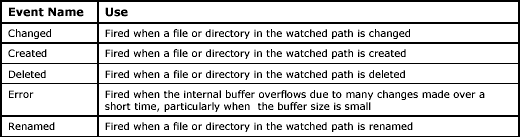
Table 6.3: FileSystemWatcher events
Listing 6.6 illustrates the use of the FileSystemWatcher class to capture changes to files and directories and report them on the console screen.
Listing 6.6: Using the FileSystemWatcher Class
using System;
using System.IO;
public class FileWatcher
{
public static void Main(string[] args)
{
// If a directory is not specified, exit program.
if (args.Length != 1)
{
// Display the proper way to call the program.
Console.WriteLine("Usage: FileWatcher.exe <directory>");
return;
}
try
{
// Create a new FileSystemWatcher and set its properties.
FileSystemWatcher watcher = new FileSystemWatcher();
watcher.Path = args[0];
// Watch both files and subdirectories.
watcher.IncludeSubdirectories = true;
// Watch for all changes specified in the NotifyFilters
//enumeration.
watcher.NotifyFilter = NotifyFilters.Attributes |
NotifyFilters.CreationTime |
NotifyFilters.DirectoryName |
NotifyFilters.FileName |
NotifyFilters.LastAccess |
NotifyFilters.LastWrite |
NotifyFilters.Security |
NotifyFilters.Size;
// Watch all files.
watcher.Filter = "*.*";
// Add event handlers.
watcher.Changed += new FileSystemEventHandler(OnChanged);
watcher.Created += new FileSystemEventHandler(OnChanged);
watcher.Deleted += new FileSystemEventHandler(OnChanged);
watcher.Renamed += new RenamedEventHandler(OnRenamed);
//Start monitoring.
watcher.EnableRaisingEvents = true;
//Do some changes now to the directory.
//Create a DirectoryInfo object.
DirectoryInfo d1 = new DirectoryInfo(args[0]);
//Create a new subdirectory.
d1.CreateSubdirectory("mydir");
//Create some subdirectories.
d1.CreateSubdirectory("mydir1\\mydir2\\mydir3");
//Move the subdirectory "mydir3 " to "mydir\mydir3"
Directory.Move(d1.FullName + "file://mydir1//mydir2//mydir3",
d1.FullName + "\\mydir\\mydir3");
//Check if subdirectory "mydir1" exists.
if (Directory.Exists(d1.FullName + "\\mydir1"))
{
//Delete the directory "mydir1"
//I have also passed 'true' to allow recursive deletion of
//any subdirectories or files in the directory "mydir1"
Directory.Delete(d1.FullName + "\\mydir1", true);
}
//Get an array of all directories in the given path.
DirectoryInfo[] d2 = d1.GetDirectories();
//Iterate over all directories in the d2 array.
foreach (DirectoryInfo d in d2)
{
if (d.Name == "mydir")
{
//If "mydir" directory is found then delete it recursively.
Directory.Delete(d.FullName, true);
}
}
// Wait for user to quit program.
Console.WriteLine("Press \'q\' to quit the sample.");
Console.WriteLine();
//Make an infinite loop till 'q' is pressed.
while (Console.Read() != 'q') ;
}
catch (IOException e)
{
Console.WriteLine("A Exception Occurred :" + e);
}
catch (Exception oe)
{
Console.WriteLine("An Exception Occurred :" + oe);
}
}
// Define the event handlers.
public static void OnChanged(object source, FileSystemEventArgs e)
{
// Specify what is done when a file is changed.
Console.WriteLine("{0}, with path {1} has been {2}", e.Name, e.FullPath, e.ChangeType);
}
public static void OnRenamed(object source, RenamedEventArgs e)
{
// Specify what is done when a file is renamed.
Console.WriteLine(" {0} renamed to {1}", e.OldFullPath, e.FullPath);
}
}
The code starts with the parameter obtained from the user in the form of a path to a directory. Then it creates a FileSystemWatcher instance to monitor all changes in files and directories. Once the FileSystemWatcher is enabled, we are ready to make some changes. In this example, a file and few directories are created and later deleted. Figure 6.2 contains the output displayed on the console screen.
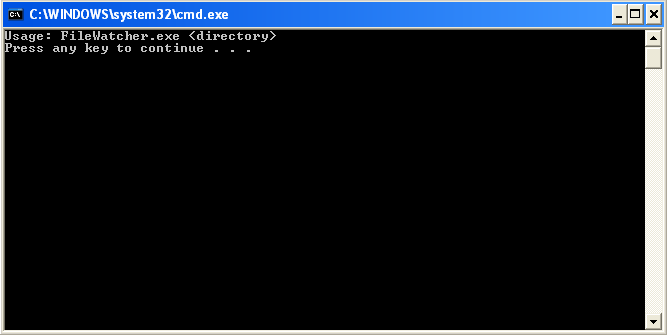
Figure 6.2: Output of the FileWatcher class
As you can see, the FileSystemWatcher captured every change made. If you start Windows Explorer and browse the directory you have provided as the parameter, you will find that every change you make in Explorer is reflected in the console. Since input is obtained from the user, chances are high that invalid arguments could raise exceptions; therefore, all the file manipulation code is placed within the try-catch block.
IOException Class
The IOException class is the base class for exceptions under the System.IO namespace. All other exceptions under this namespace-DirectoryNotFoundException, EndOfStreamException, FileNotFoundException, and FileLoadException-derive from the IOException class. I/O operations deal with various resources like hard disk, floppy disk, and network sockets, which have a greater chance of failing. Therefore, you should always encapsulate your I/O code within the trycatch block to intercept any exceptions that might occur.
Conclusion
Hope this article would have helped you in understanding FileSystemWatcher and IOException Class in C#. See other articles on the website on .NET and C#.
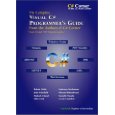 |
The Complete Visual C# Programmer's Guide covers most of the major components that make up C# and the .net environment. The book is geared toward the intermediate programmer, but contains enough material to satisfy the advanced developer. |
More Readings
Here are some more practical examples of FileSystemWatcher.