I've spent the last week trying to get up to speed with F# and am very exicted about the language.
It's tough to change from thinking OOP (which I've been doing for years) to thinking functionally. If you haven't taken the plunge, I would definitely recommend learning F# because not only is it a VERY useful tool to have for certain problems, it will make you a better C# coder especially since C# has become much more "functional" with the 3.5 release.
One of the really cool things is that if you have been working with C# for a while, all of the libraries you have learned will still be there once you get F# under your fingers.
I'll continue to write about the ways I come to understand different facets of F# coming from being a C# coder for years. If you are with me, hold on to you hat... you are in for a real ride!
To get started, you have to download the compiler and get your environment set up. I'm using VisualStudio2008, but you can use 2005 as well. The main thing for now is to get the scripting host plug-in.
Download the compiler, scripting utility and VisualStudio plugin from http://research.microsoft.com/fsharp/release.aspx
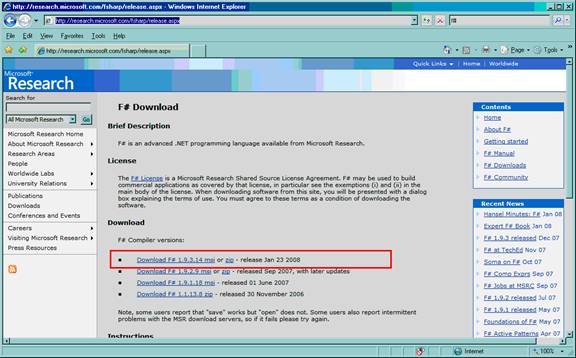
NOTE: At the time this article was written I was using the the "1.9.3.14" release
After you download the F# compiler and install it you can make a new F# project. The template is in the "Other Project Types" tree branch of the "New Project" dialog.
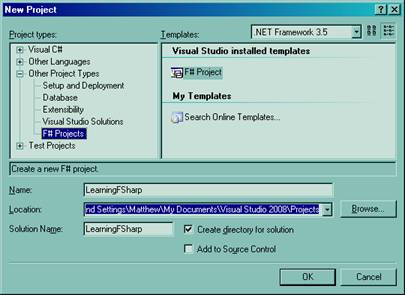
Once you create the project, we have to hook up the plugin, so go to Tools > Add-in Manager...
Then check the boxes on the "F# Interactive for Visual Studio" line. If this hasn't shown up for you, you probably have a problem with the install.
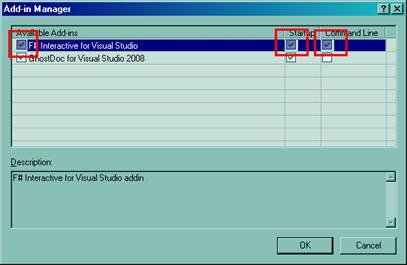
Click "OK"
Now we are ready to get started....
From your solution explorer... right-click on the project and select Add > New Item...
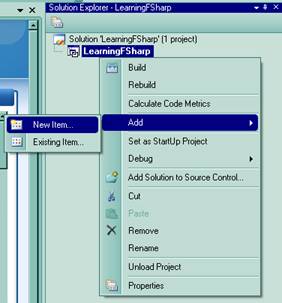
We'll just be poking around for now and I'll probably get into all the file types another time. For now, just choose "F# Source File"
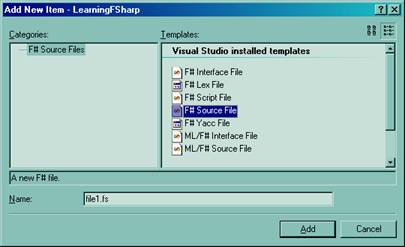
And click on "Add" and you'll get a bunch of template code that is interesting to look through but was a little overwhelming for me at first.
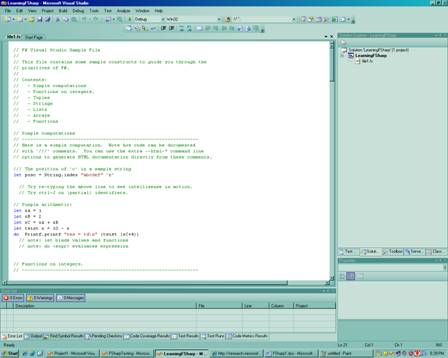
So select all the code and delete it so we have a clean slate to start with.
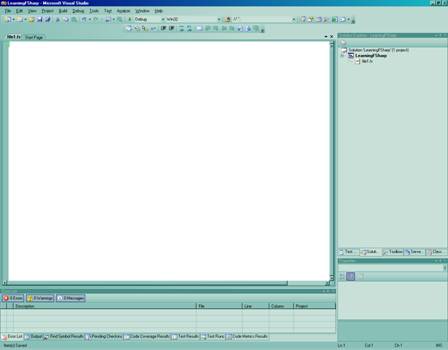
On the first line of your code page type "3+4" select what you have typed and while holding down the [Alt] key hit the [Enter]. [Alt + Enter] will execute whatever code you have selected in your main code window in the interactive environment (the plug-in we added earlier).
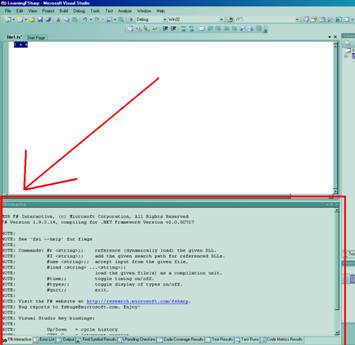
This is the environment we'll be using from now on. After all the introductory text in the F# interactive window, you should see
> val it : int = 7
Meaning the value of our expression has evaluated to 7 (the value of it is 7)
You can right-click anywhere in the F# interactive window and select "clear" to clean up the text.
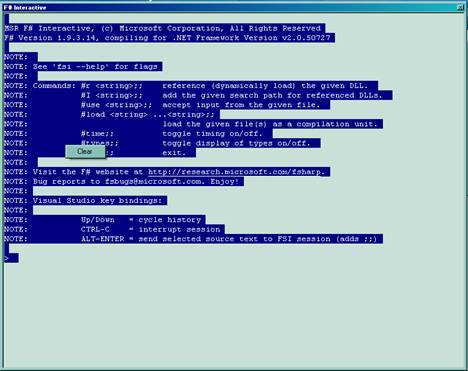
So we have a clean slate to start with.
From now on, we'll just be using the F# interactive window just to get familiar with the language.
Whenever you type a command into the F# interactive window, you need to terminate it with two semi-colons ";;"
Let's look at the simplest parts of the language right now. Every time you type a command with a terminating ";;" in the interactive window, you will get a response back which makes it an ideal environment for playing with the language when getting started.
If you type in
3;;
You will get the type of the thing you entered and the value of it,
3;;
val it : int = 3
So we can see we have an integer (int) type with a value of 3.
If you type in "3.0;;" you see we have a float
3.0;;
val it : float = 3.0
If you type in "3+4;;" you see we have another int
3+4;;
val it : int = 7
and if you type in "3+4.0;;" what do you think we get?
That's right... an error. (Exciting, isn't it?)
3+4.0;;
> 3+4.0;;
--^^^^
stdin(6,2): error: FS0001: This expression has type
float
but is here used with type
int
stopped due to error
This is because F# is strongly typed and our types on both sides of the ‘+' sign have to match.
Let's look at the plus sign a little closer. Type in "(+);;"
(+);;
val it : (int -> int -> int) = <fun:it@8>
Ok... now it starts to get interesting. What the heck does that mean?
Basically we have a new "type" which is a function. The value of ‘+' is actually a function that takes two integers and outputs an integer which gives it a type of "int -> int -> int". The far right hand side of the "->" chain is the output, so if we were to input two integers A and B and get a result C this is how it would line up with our function syntax:
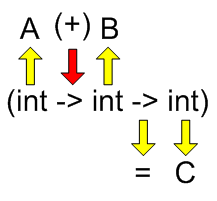
Since we are dealing with a functional language, functions are really first-class variables, no different from integers, floats, doubles, or strings.
Anyways... you get the idea. Using F# interactive to explore is a great way get a feel of the language.
I'll be back with more later and start to dive a bit deeper.
Until next time,
happy coding