Please, I really need help. I have code that ask user to key-in the target, random number and how much combination that user want. Then, the output must bigger than the target .
This is the output :
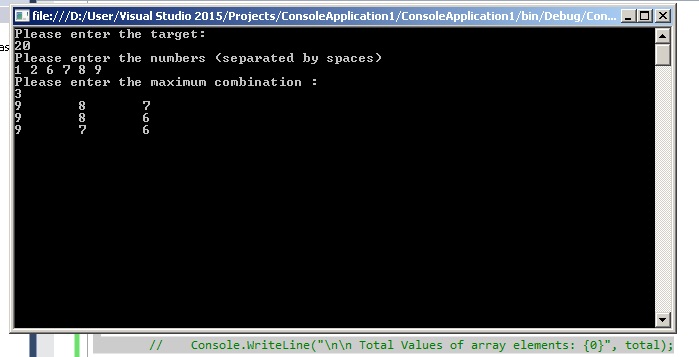
So how can i change this output to be like this :
9 8 7 = 24
9 8 6 = 23
9 7 6 = 22
And then after sum the number, I need to subtract the answer with the target that user key-in. Example like this:
24 - 20 = 4
23 - 20 = 3
22 - 20 = 2
Here is my code that I do to get the output like the image:
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using System.Threading.Tasks;
-
-
- class Program
- {
- static void Main(string[] args)
- {
-
- string input;
- int NoDisplay;
- decimal goal;
- decimal element;
-
- do
- {
- Console.WriteLine("Please enter the target:");
- input = Console.ReadLine();
- }
- while (!decimal.TryParse(input, out goal));
-
- Console.WriteLine("Please enter the numbers (separated by spaces)");
- input = Console.ReadLine();
- string[] elementsText = input.Split(' ');
- List elementsList = new List();
- foreach (string elementText in elementsText)
- {
- if (decimal.TryParse(elementText, out element))
- {
- elementsList.Add(element);
-
- }
-
- }
-
- int i;
- int j;
- decimal tmp;
- int[] arr1 = new int[10];
-
- for (i = 0; i < elementsList.Count; i++)
-
- {
- for (j = i + 1; j < elementsList.Count; j++)
- {
- if (elementsList[i] < elementsList[j])
- {
- tmp = elementsList[i];
- elementsList[i] = elementsList[j];
- elementsList[j] = tmp;
- }
- }
- }
-
-
-
-
- Console.WriteLine("Please enter the maximum combination :");
- NoDisplay = Convert.ToInt32(Console.ReadLine());
-
-
- Solver solver = new Solver();
- List> results = solver.Solve(goal, elementsList.ToArray());
-
-
-
- int counter = 0;
-
- Boolean recordexist = false;
- foreach (List result in results)
- {
-
- if (counter == 3) break;
- if (result.Count == NoDisplay)
- {
- recordexist = true;
- foreach (decimal value in result)
- {
- Console.Write("{0}\t", value);
- }
- if (recordexist == true)
- {
-
- Console.WriteLine();
- }
-
- counter++;
-
- }
- }
-
- if (recordexist == false)
- {
- Console.WriteLine("No record exist");
- }
-
- Console.ReadLine();
- }
- }
-
- public class Solver
- {
-
- private List> mResults;
-
- public List> Solve(decimal goal, decimal[] elements)
- {
-
- mResults = new List>();
-
- RecursiveSolve(goal, 0.0m,
- new List(), new List(elements), 0);
- return mResults;
- }
-
-
- private void RecursiveSolve(decimal goal, decimal currentSum,
- List included, List notIncluded, int startIndex)
- {
-
- for (int index = startIndex; index < notIncluded.Count; index++)
- {
-
- decimal nextValue = notIncluded[index];
- if (currentSum + nextValue > goal)
- {
-
- List newResult = new List(included);
- newResult.Add(nextValue);
- mResults.Add(newResult);
-
-
-
- }
-
- else if (currentSum + nextValue < goal)
- {
-
- List nextIncluded = new List(included);
- nextIncluded.Add(nextValue);
- List nextNotIncluded = new List(notIncluded);
- nextNotIncluded.Remove(nextValue);
- RecursiveSolve(goal, currentSum + nextValue,
- nextIncluded, nextNotIncluded, startIndex++);
- }
-
-
- }
-
-
-
- }
-
- }