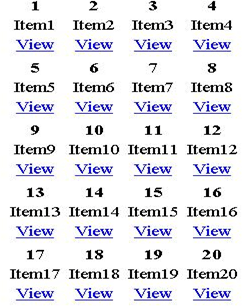
#region Events
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
//set control settings
PagingControl1.NoRecordsPerPage = 20;
PagingControl1.CurrentPageIndex = 1;
PagingControl1.NavigationLinkesCount = 12;
//Get Items data
ViewState["_Items"] = GetItems();
ViewPage(PagingControl1.CurrentPageIndex);
}
}
protected void PagingControl1_PageIndexClick(object sender, EventArgs e)
{
ViewPage(PagingControl1.CurrentPageIndex);
} #endregion
#region Methods
private void ViewPage(int PageNo)
{
if (ViewState["_Items"] != null)
{
PagedDataSource objPds = new PagedDataSource();
DataTable dt = (DataTable)ViewState["_Items"];
objPds.DataSource = new DataView(dt);
objPds.AllowPaging = true;
objPds.PageSize = PagingControl1.NoRecordsPerPage;
objPds.CurrentPageIndex = PageNo - 1;
dlstItems.DataSource = objPds;
dlstItems.DataBind();
double d = Math.Ceiling(Convert.ToDouble(dt.Rows.Count) / PagingControl1.NoRecordsPerPage);
PagingControl1.PagesCount = Convert.ToInt32(d);
dlstItems.Visible = true;
PagingControl1.Visible = true;
lblResultMessage.Visible = false;
}
else
{
dlstItems.Visible = false;
lblResultMessage.Visible = true;
PagingControl1.Visible = false;
lblResultMessage.Text = "No Result";
}
}
private DataTable GetItems()
{
DataTable dt = new DataTable();
dt.Columns.Add("ItemId");
dt.Columns.Add("ItemName");
for (int i = 1; i <= 400; i++)
{
DataRow dr = dt.NewRow();
dr["ItemId"] = i.ToString();
dr["ItemName"] = "Item" + i;
dt.Rows.Add(dr);
}
return dt;
} #endregion
The Control Properties and events (Source Code):
public class PagingControl : WebControl
{
#region Variables
Label lblCount = new Label();
#endregion
#region Properties
#region Appearance
public enum Alignment
{
Center,
Right,
Left,
}
public enum Position
{
Top,
Bottom,
Right,
Left,
}
[Bindable(true)]
[Category("Appearance")]
[DefaultValue("")]
[Localizable(true)]
public string Text
{
get
{
String s = Convert.ToString(ViewState["Text"]);
if (s == null || s.Length==0)
{
return String.Empty;
}
else
{
return s;
}
}
set
{
ViewState["Text"] = value;
}
}
[Bindable(true), Category("Appearance"), DefaultValue("||"), Localizable(true)]
[Browsable (true)]
public string LinkSeparatorText
{
get
{
string s = Convert.ToString(ViewState["_LinkSeparatorText"]);
if (s == null || s.Length==0)
{
return "||";
}
else
{
return s;
}
}
set
{
ViewState["_LinkSeparatorText"] = value;
}
}
[Bindable(true), Category("Appearance"), DefaultValue("Prev"), Localizable(true)]
[Browsable(true)]
public String PreviousButtonText
{
get
{
String s = Convert.ToString(ViewState["_PreviousButtonText"]);
if (s == null || s.Length==0)
{
return "Prev";
}
else
{
return s;
}
}
set
{
ViewState["_PreviousButtonText"] = value;
}
}
[Bindable(true), Category("Appearance"), DefaultValue("Next"), Localizable(true)]
public String NextButtonText
{
get
{
String s = Convert.ToString(ViewState["_NextButtonText"]);
if (s == null || s.Length==0)
{
return "Next";
}
else
{
return s;
}
}
set
{
ViewState["_NextButtonText"] = value;
}
}
[Bindable(true), Category("Appearance"), DefaultValue("First"), Localizable(true)]
public String FirstButtonText
{
get
{
String s = Convert.ToString(ViewState["_FirstButtonText"]);
if (s == null || s.Length==0)
{
return "First";
}
else
{
return s;
}
}
set
{
ViewState["_FirstButtonText"] = value;
}
}
[Bindable(true), Category("Appearance"), DefaultValue("Last"), Localizable(true)]
public String LastButtonText
{
get
{
String s = Convert.ToString(ViewState["_LastButtonText"]);
if (s == null || s.Length==0)
{
return "Last";
}
else
{
return s;
}
}
set
{
ViewState["_LastButtonText"] = value;
}
}
[Bindable(true), Category("Appearance"), DefaultValue(" Of "), Localizable(true)]
public String SeparatedCountText
{
get
{
String s = Convert.ToString(ViewState["_SeparatedCountText"]);
if (s == null || s.Length==0)
{
return " Of ";
}
else
{
return s;
}
}
set
{
ViewState["_SeparatedCountText"] = value;
}
}
[Bindable(true), Category("Appearance"), DefaultValue("("), Localizable(true)]
public String BeforeCountText
{
get
{
String s = Convert.ToString(ViewState["_BeforeCountText"]);
if (s == null || s.Length==0)
{
return "(";
}
else
{
return s;
}
}
set
{
ViewState["_BeforeCountText"] = value;
}
}
[Bindable(true), Category("Appearance"), DefaultValue(")"), Localizable(true)]
public String AfterCountText
{
get
{
String s = Convert.ToString(ViewState["_AfterCountText"]);
if (s == null || s.Length==0)
{
return ")";
}
else
{
return s;
}
}
set
{
ViewState["_AfterCountText"] = value;
}
}
[Bindable(true), Category("Appearance"), DefaultValue("Alignment.Center"), Localizable(true)]
public Alignment CountAlignment
{
get
{
Alignment s = Alignment.Center;
if(ViewState["_CountAlignment"]!=null)
s = (Alignment)ViewState["_CountAlignment"];
return s;
}
set
{
ViewState["_CountAlignment"] = value;
}
}
[Bindable(true), Category("Appearance"), DefaultValue("Position.Bottom"), Localizable(true)]
public Position CountPosition
{
get
{
Position s = Position.Bottom;
if (ViewState["_CountPosition"] != null)
s = (Position)ViewState["_CountPosition"];
return s;
}
set
{
ViewState["_CountPosition"] = value;
}
}
#endregion
#region Visibility"
[Bindable(true), Category("Visibility"), DefaultValue("true"), Localizable(true)]
public Boolean ShowCount
{
get
{
Boolean s = false;
if (ViewState["_ShowCount"] == null)
{
s = true;
}
return s;
}
set
{
ViewState["_ShowCount"] = value;
}
}
[Bindable(true), Category("Visibility"), DefaultValue("true"), Localizable(true)]
public Boolean ShowFirst
{
get
{
Boolean s = false;
if (ViewState["_ShowFirst"] == null)
{
s = true;
}
return s;
}
set
{
ViewState["_ShowFirst"] = value;
}
}
[Bindable(true), Category("Visibility"), DefaultValue("true"), Localizable(true)]
public Boolean ShowLast
{
get
{
Boolean s = false;
if (ViewState["_ShowLast"] == null)
{
s = true;
}
return s;
}
set
{
ViewState["_ShowLast"] = value;
}
}
[Bindable(true), Category("Visibility"), DefaultValue("true"), Localizable(true)]
public Boolean ShowPrevious
{
get
{
Boolean s = false;
if (ViewState["_ShowPrevious"] == null)
{
s = true;
}
return s;
}
set
{
ViewState["_ShowPrevious"] = value;
}
}
[Bindable(true), Category("Visibility"), DefaultValue("true"), Localizable(true)]
public Boolean ShowNext
{
get
{
Boolean s = false;
if (ViewState["_ShowNext"] == null)
{
s = true;
}
return s; }
set
{
ViewState["_ShowNext"] = value;
}
}
#endregion
#region Paging"
private Int32 _CurrentStartPage;
public Int32 CurrentStartPage
{
get
{
if ((CurrentPageIndex % NavigationLinkesCount == 0))
{
_CurrentStartPage = (CurrentPageIndex - NavigationLinkesCount) + 1;
}
else
{
_CurrentStartPage = (CurrentPageIndex - (CurrentPageIndex % NavigationLinkesCount)) + 1;
}
return _CurrentStartPage;
}
}
private Int32 _CurrentPageIndex;
public Int32 CurrentPageIndex
{
get
{
if ((ViewState["_CurrentPageIndex"] == null))
{
_CurrentPageIndex = 1;
}
else
{
_CurrentPageIndex = Convert.ToInt32(ViewState["_CurrentPageIndex"]);
}
return _CurrentPageIndex;
}
set
{
_CurrentPageIndex = value;
ViewState["_CurrentPageIndex"] = _CurrentPageIndex;
}
}
private Int32 _PagesCount;
public Int32 PagesCount
{
get
{
if (ViewState["_PagesCount"] == null)
{
_PagesCount = 1;
}
else
{
_PagesCount = Convert.ToInt32(ViewState["_PagesCount"]);
}
return _PagesCount;
}
set
{
_PagesCount = value;
ViewState["_PagesCount"] = _PagesCount;
}
}
/// <summary>
/// count of navigation linkes that will appears
/// </summary>
private Int32 _NavigationLinkesCount;
public Int32 NavigationLinkesCount
{
get
{
if (ViewState["_NavigationLinkesCount"] == null)
{
_NavigationLinkesCount = 1;
}
else
{
_NavigationLinkesCount = Convert.ToInt32(ViewState["_NavigationLinkesCount"]);
}
return _NavigationLinkesCount;
}
set
{
_NavigationLinkesCount = value;
ViewState["_NavigationLinkesCount"] = _NavigationLinkesCount;
}}
private Int32 _NoRecordsPerPage;
public Int32 NoRecordsPerPage
{
get
{
if (ViewState["_NoRecordsPerPage"] == null)
{
_NoRecordsPerPage = 1;
}
else
{
_NoRecordsPerPage = Convert.ToInt32(ViewState["_NoRecordsPerPage"]);
}
return _NoRecordsPerPage;
}
set
{
_NoRecordsPerPage = value;
ViewState["_NoRecordsPerPage"] = _NoRecordsPerPage;
}
}
#endregion
#endregion
#region Methods
private void GeneratePaging()
{
this.Controls.Clear();
#region Count Label Top Left
// add count label according to it's position
lblCount = new Label();
lblCount.ID = this.ClientID + "_" + "lblCount";
lblCount.Visible = ShowCount;
if (CountPosition == Position.Top)
{
// Controls.Add(new LiteralControl("<table width='100%'><tr><td align='" + CountAlignment.ToString() + "'" + ">"))
Controls.Add(new LiteralControl("<div style='text-align: " + CountAlignment.ToString() + "'>"));
this.Controls.Add(lblCount);
Controls.Add(new LiteralControl("</div>"));
}
else if (CountPosition == Position.Left)
{
this.Controls.Add(lblCount);
}
#endregion
Int32 EndPage = CurrentStartPage + NavigationLinkesCount - 1;
if ((EndPage > PagesCount))
{
EndPage = PagesCount;
}
LinkButton lbtn;
for (int i = CurrentStartPage; i <= EndPage; i++)
{
#region First Button And Prev
//First Button And Prev
if (CurrentPageIndex > 1 && (i == CurrentStartPage))
{ // added once in start
//First
lbtn = new LinkButton();
lbtn.Text = FirstButtonText;
lbtn.ID = this.ClientID + "_" + "btnFirst";
lbtn.CausesValidation = false;
lbtn.Visible = ShowFirst;
lbtn.Click += LinkedButtonClick;
Controls.Add(lbtn);
if (ShowFirst)
{
Controls.Add(new LiteralControl(LinkSeparatorText));
//Previous
lbtn = new LinkButton();
lbtn.Text = PreviousButtonText;
lbtn.ID = this.ClientID + "_" + "btnPrevPage" + (CurrentPageIndex - 1);
lbtn.CausesValidation = false;
lbtn.Visible = ShowPrevious;
lbtn.Click += LinkedButtonClick;
Controls.Add(lbtn);
Controls.Add(new LiteralControl(LinkSeparatorText));
}}
#endregion
#region Pages No
//Page Index
lbtn = new LinkButton();
lbtn.Text = i.ToString();
lbtn.ID = this.ClientID + "_" + "btn" + i.ToString();
lbtn.CausesValidation = false;
lbtn.Click += LinkedButtonClick;
Controls.Add(lbtn);
if (PagesCount > 1)
{
Controls.Add(new LiteralControl(LinkSeparatorText));
}
lbtn.Enabled = true;
#endregion
#region Next Button And Last
//Next Button And Last
//added once if( CurrentPageIndex less than
if (CurrentPageIndex < PagesCount && (i == EndPage))
{ //add once in End
//Next
lbtn = new LinkButton();
lbtn.Text = NextButtonText;
lbtn.ID = this.ClientID + "_" + "btnNextPage" + (CurrentPageIndex + 1);
lbtn.CausesValidation = false;
lbtn.Visible = ShowNext;
lbtn.Click += LinkedButtonClick;
Controls.Add(lbtn);
if (ShowNext)
{
Controls.Add(new LiteralControl(LinkSeparatorText));
//Last
lbtn = new LinkButton();
lbtn.Text = LastButtonText;
lbtn.ID = this.ClientID + "_" + "btnLast" + (i + 1);
lbtn.CausesValidation = false;
lbtn.Visible = ShowLast;
lbtn.Click += LinkedButtonClick;
Controls.Add(lbtn);
}
}
#endregion
}
#region Count Label Bottom Right
//add count label according to it's position
if (CountPosition == Position.Bottom)
{
Controls.Add(new LiteralControl("<br>"));
Controls.Add(new LiteralControl("<div style='text-align: " + CountAlignment.ToString() + "'>"));
this.Controls.Add(lblCount);
Controls.Add(new LiteralControl("</div>"));}
else if (CountPosition == Position.Right)
{
this.Controls.Add(lblCount);
}
#endregion
}
private void SelectPage()
{
Int32 PageIndex = CurrentPageIndex;
Control ctl = FindControl(this.ClientID + "_" + "btn" + PageIndex);
if (ctl != null)
{
LinkButton btnToBeClicked = (LinkButton)ctl;
if (btnToBeClicked != null)
{
btnToBeClicked.Enabled = false;
lblCount.Text = BeforeCountText + PageIndex + SeparatedCountText + PagesCount + AfterCountText;
}
}
}
public void PaintWithoutClickSelected()
{
GeneratePaging();
SelectPage();
}
public void PaintWithClickSelected()
{
PaintWithoutClickSelected();
FirePageClickEvent();
}
#endregion
#region Event
/// <summary>
/// page index click event fire when click on navegations buttons
/// </summary>
public event EventHandler PageIndexClick;
protected override void OnLoad(System.EventArgs e)
{
//Generate Linked Buttons
PaintWithoutClickSelected();
base.OnLoad(e);
}
protected override void CreateChildControls()
{
base.CreateChildControls();
}
protected override void OnPreRender(EventArgs e)
{
//Generate Linked Buttons
PaintWithoutClickSelected();
base.OnPreRender(e);
}
protected override void Render(System.Web.UI.HtmlTextWriter writer)
{
foreach (Control ctl in this.Controls)
{
if (ctl != null)
{
ctl.RenderControl(writer);
}
}
base.Render(writer);
}
protected override void RenderContents(HtmlTextWriter output)
{
output.Write(Text);
}
protected void LinkedButtonClick(Object sender, EventArgs e)
{
LinkButton lbtn = (LinkButton)sender;
if ((lbtn.ID.IndexOf(this.ClientID + "_" + "btnFirst") > -1))
{
CurrentPageIndex = 1;
}
else if ((lbtn.ID.IndexOf(this.ClientID + "_" + "btnPrevPage") > -1))
{
if ((CurrentPageIndex > 1))
{
CurrentPageIndex = CurrentPageIndex - 1;
}
}
else if ((lbtn.ID.IndexOf(this.ClientID + "_" + "btnNextPage") > -1))
{
if ((CurrentPageIndex < PagesCount))
{
CurrentPageIndex = CurrentPageIndex + 1;
}
}
else if ((lbtn.ID.IndexOf(this.ClientID + "_" + "btnLast") > -1))
{
CurrentPageIndex = PagesCount;
}
else
{
CurrentPageIndex = Convert.ToInt32(lbtn.Text);
}
PaintWithClickSelected();
}
private void FirePageClickEvent()
{
if (PageIndexClick != null)
{
PageIndexClick(null, null);
}
}
#endregion
}