To know what a MongoDB is and how to install it and how to install the C# MongoDB driver please see the article "Mongo database in .Net with C#".
Now we are creating the sample application.
Step 1:
Create a console application named "MongodbConsole" Application.
Now write the following code under the namespace area:
using MongoDB.Driver;
using MongoDB.Driver.Bson;
MongoDB.Driver is the C# driver for using the MongoDB database in a C# .Net application.
Step 2:
Now go to the "bin" folder under the "mongodb-win32-i386-1.8.1" folder. After that click the "mongod" exe just like figure 1. It is the server for the MongoDB. You must open the MongoDB database server while you are creating an application using MongoDB. We can open the MongoDB database server by using the windows service also.
Fig 1:

Now we have to first connect to the database. So under the void main we will write the code:
var mongo = new Mongo();
mongo.Connect();
var db = mongo.getDB("testmongo");
Here we are creating the object variable of "Mongo" database. Then we are doing to open the connection of the database by writing "mongo.Connect();". It is the same as "con.open()" for ADO.Net programming.
"mongo.getDB("testmongo");" means it will create the database name "testmongo" under the
"c:\Data\db" folder.
Now write the following code:
var categorylist = db.GetCollection("studcategories");
var category = new Document();
category ["Name"] = "shirsendu nandi";
category ["Id"] = "20";
category["Address"] = "bangalore";
categorylist.Insert(category);
Console.WriteLine("Total number of data is:-" + categorylist.Count());
Now "db.GetCollection ("studcategories");" means it will create a collection name "studcategories" under the "testmongo" database.
After that we are creating the variable of document (for e.g.: table in sqlserver).
Now we are assigning the the variable with name, id, age.
For e.g.: category ["Name"] = "shirsendu Nandi"; here the "Name" is the key value of the document and the "category ["Name"]" is the field. You can consider that to be like a "Dictionary" or "Hashtable" in .Net collection classes.
Now we are are saving the category value in the mongo database by writing this code:
"categorylist.Insert (category);"
We also get the total count of the records using "categorylist.Count ());"
Now press F5. Your record has been stored in the mongo db. To see that go to the "c:\Data\db" folder. You will see "testmongo.ns" has been created just like figure 2 (marked with red). By default the size of the database will be 16MB. It will increase automatically according to the data. But for 32 bit Windows the maximum memory capacity will be 2 GB.
Fig 2:

Step 3:
Now we have to retrieve the data from the "studcategories" and display it.
For that we have to write the code shown below:
var categories = db.GetCollection("studcategories").FindAll().Documents;
foreach(var lstcategory in categories)
{
Console.WriteLine(lstcategory["Name"]);
Console.WriteLine(lstcategory["Id"]);
Console.WriteLine(lstcategory["Adress"]);
}
Here first we find all the records from the documents of "studcategories" by using "FindAll().Documents;".
After that we loop through each "categories" and display the records.
So the complete code is:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using MongoDB.Driver;
using MongoDB.Driver.Bson;
namespace mongodbconsoleapplication
{
class Program
{
static void Main(string[] args)
{
var mongo = new Mongo();
mongo.Connect();
var db = mongo.getDB("testmongo");
var categorylist = db.GetCollection("studcategories");
var category = new Document();
category["Name"] = "shirsendu nandi";
category["Id"] = "20";
category["Adress"] = "bangalore";
categorylist.Insert(category);
Console.WriteLine("Total number of data is:-" + categorylist.Count());
var categories = db.GetCollection("studcategories").FindAll().Documents;
foreach(var lstcategory in categories)
{
Console.WriteLine(lstcategory["Name"]);
Console.WriteLine(lstcategory["Id"]);
Console.WriteLine(lstcategory["Adress"]);
}
Console.ReadLine();
}
}
}
Now run the application; it will look like the figure shown below:
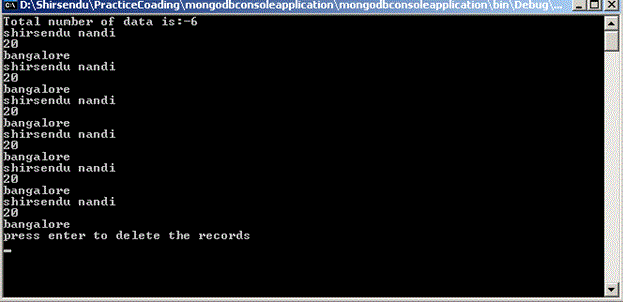
Conclusion:
So in this article I have described how to save and fetch data from a mongo db using C# .net.