Before getting into the code, let's discuss why we need to do this programmatically rather than manually. This kind of functionality is usually required when we are performing an automated deployment process, where we need to create a shared folder for certain user accounts.
C# Code Using ManagementClass
public void ShareFolderPermission(string FolderPath, string ShareName, string Description)
{
try
{
// Calling Win32_Share class to create a shared folder
ManagementClass managementClass = new ManagementClass("Win32_Share");
// Get the parameter for the Create Method for the folder
ManagementBaseObject inParams = managementClass.GetMethodParameters("Create");
ManagementBaseObject outParams;
// Assigning the values to the parameters
inParams["Description"] = Description;
inParams["Name"] = ShareName;
inParams["Path"] = FolderPath;
inParams["Type"] = 0x0;
// Finally Invoke the Create Method to do the process
outParams = managementClass.InvokeMethod("Create", inParams, null);
// Validation done here to check sharing is done or not
if ((uint)(outParams.Properties["ReturnValue"].Value) != 0)
MessageBox.Show("Folder might be already in share or unable to share the directory");
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
Code Explanation
- ManagementClass
It is a WMI class which is generally used to access the WMI data through the ManagementPath that we pass through the constructor of the ManagementClass.
E.g.:- ManagementClass managementClass = new ManagementClass("Win32_Share");
- ManagementBaseObject
It contains the basic elements of the management object. It serves as a more specific management object classes.
E.g.:- ManagementBaseObject inParams = managementClass.GetMethodParameters("Create");
Execution
Call the above function like this
ShareFolderPermissions(@"D:\ Test", "Test", "TestApplication");
Once executed, go to the above specified path, right click the folder and view the properties of it. Go to Sharing tab you can see the result like this.
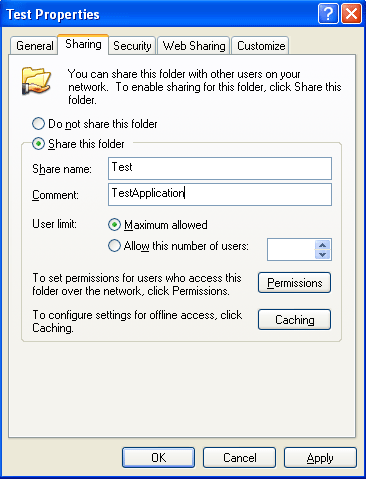
That's the explanation about the code used here. Rest of the code is understandable.