How memory is managed in .Net?
I studied many articles about memory management, but they are all confusing about when memory is allocated in the stack and when memory is allocated in the heap. But I always got confused. But finally, I got exactly what I wanted.
Hopefully this article will help some of my friends who are still confused about the Stack and Heap.
In a simple way I can say that ....
As we know the Common Language Runtime (CLR) automatically handles Memory Management with the help of its components. Component one is the Garbage Collector which plays an important role in Memory Management. It contains two methods (Dispose and Finalize). Objects are automatically freed when they are no longer needed by the application through Garbage Collector.
When the Garbage Collector will be called to clean up unnecessary objects from memory. Non deterministic finalization is performed when the CLR foresees a memory deficiency.
How memory is managed for Value Type:
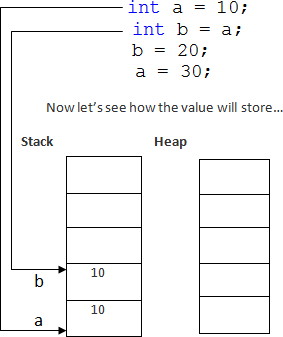
In above figure you can see what happens in first two statements, simple value of variable a and b is storing in stack, now let's see what happen in 3rd and 4th statement.
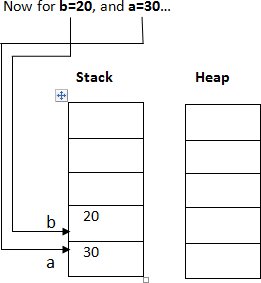
As we can see that simply changes the position where the variable is actually stored. So in these statements we can see that there is no use of Heap.
Now we will just see for Reference Type:
class Program
{
public int value;
static void Main(string[] args)
{
Program prog = new Program();
prog.value = 100;
Program prog1 = prog;
prog1.value = 200;
}
}
Now let's see how value will store in stack and heap in reference type.
Now we can see here for first statement...
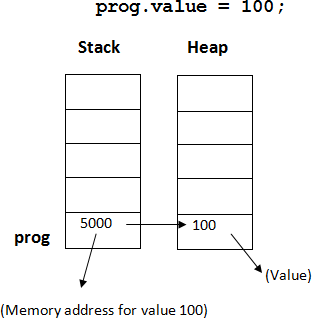
Now we will see for next two statements:
Program prog1 = prog;
prog1.value = 200;
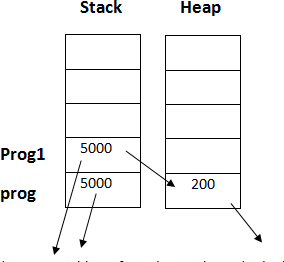
(Memory address for value 100) (Value)
Now we can see that the address is transferred from prog to prog1, and address is same so the value of that particular address is going to change as change is having on address so the value will be overriden in the Heap.
Hope you have gotten idea that how exactly reference type stores the value....
Now finally I will talk about very important thing that is how exactly string stores:
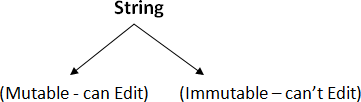
class Program
{
static void Main(string[] args)
{
string s = "Hello";
string ss = s;
s = s + " " + "and Welcome";
Console.WriteLine(s);
Console.WriteLine(ss);
Console.ReadKey();
}
}
Now we will see how value will store for this program:
For first statement:
string s = "Hello";
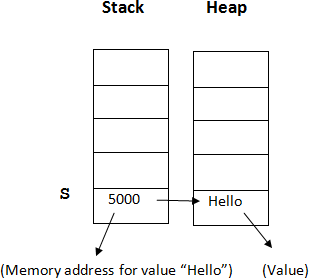
For second statement...
string ss = s;
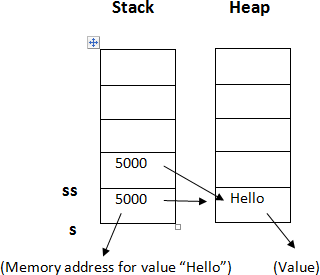
In this diagram we can see that the address is transferred from s to ss, so as well as the value will also be transferred to ss, so in heap value will be overriden.
Now come for the next statement....
s = s + " " + "and Welcome";
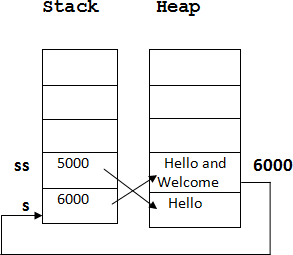
As in this image we can see that, as we just add "and welcome" in string "s", then the value is changed at the address but in the heap hello will be at it's own position so the value will not be overriden, and the new value of "s" will be stored at the new address which is here 6000. So now the address also will change for "s", now the new address for "s" is 6000.
Hope you understand that how exactly value stored in stack and heap...
Thanks...
By,
Yogendra Kumar