LinkedHashSet Class In Java
LinkedHashSet Class
In Java, LinkedHashSet class holds only unique elements like HashSet. It extends HashSet class and implements Set interface. But it maintains insertion order.
Constructors of LinkedHashSet Class
HashSet( )
This constructor is used to construct a default HashSet.
HashSet(Collection c)
This constructor is used to initialize the hash set, using the elements of the collection c.
LinkedHashSet(int capacity)
This constructor is used to initialize the capacity of the LinkedHashSet to the given integer value capacity and the capacity grows automatically as elements are added to the HashSet.
LinkedHashSet(int capacity, float fillRatio)
This constructor is used to initialize both the capacity and the fill ratio (also called load capacity) of the hash set from its arguments.
Let’s see an example, given below.
Code
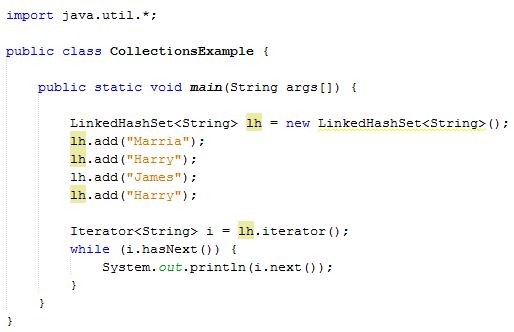
- import java.util.*;
- public class CollectionsExample {
- public static void main(String args[]) {
- LinkedHashSet < String > lh = new LinkedHashSet < String > ();
- lh.add("Marria");
- lh.add("Harry");
- lh.add("James");
- lh.add("Harry");
- Iterator < String > i = lh.iterator();
- while (i.hasNext()) {
- System.out.println(i.next());
- }
- }
- }
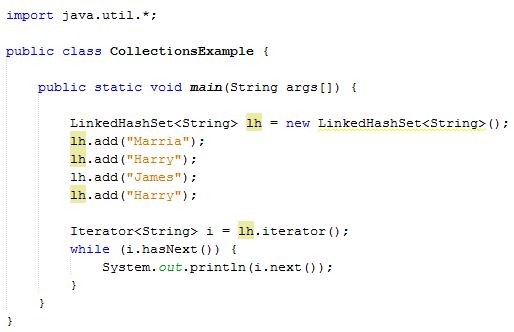
Output
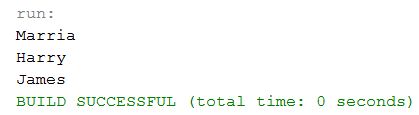
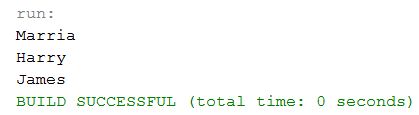
In the example, shown above, we can observe the output. LinkedHashSet have preserved the insertion order.
Summary
Thus, we learnt that Java LinkedHashSet class holds only unique elements like HashSet. It extends HashSet class and implements Set interface and also learnt how to create it in Java.