Compress And Uncompress File In Java
Compress and Uncompress file
In Java, DeflaterOutputStream and InflaterInputStream classes are used to compress and uncompress the data in the deflate compression format.
DeflaterOutputStream class
The DeflaterOutputStream class is used to compress the data in the deflate compression format in Java. It has a capability to the other compression filters like GZIPOutputStream.
Let’s see an example, given below, with DeflaterOutputStream class.
Code
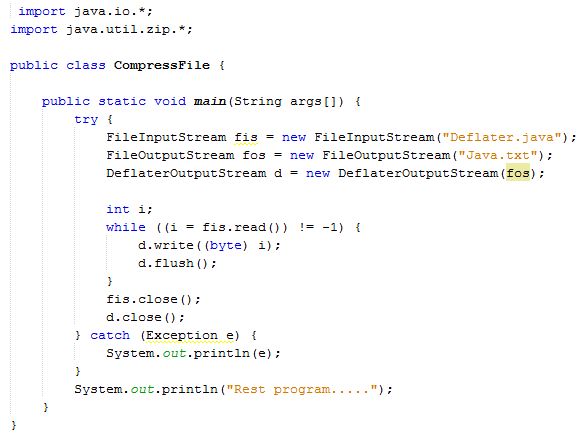
- import java.io.*;
- import java.util.zip.*;
- public class CompressFile {
- public static void main(String args[]) {
- try {
- FileInputStream fis = new FileInputStream("Deflater.java");
- FileOutputStream fos = new FileOutputStream("Java.txt");
- DeflaterOutputStream d = new DeflaterOutputStream(fos);
- int i;
- while ((i = fis.read()) != -1) {
- d.write((byte) i);
- d.flush();
- }
- fis.close();
- d.close();
- } catch (Exception e) {
- System.out.println(e);
- }
- System.out.println("Rest program.....");
- }
- }
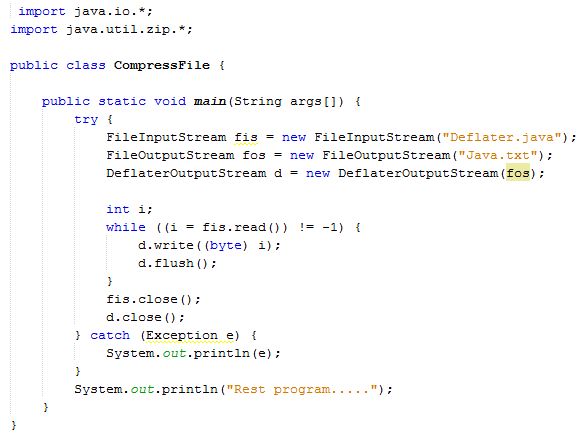
In the example, mentioned above, we read the data of a file and compress it into another file with the use of DeflaterOutputStream class. We can compress any file. At this time, we compress the Deflater.java file.
InflaterInputStream class
The InflaterInputStream class is used to uncompress or decompress the file in the deflate compression format in Java. It has a capability to the other decompression filters like GZIPInputStream class.
Let’s see an example, given below, with InflaterInputStream class.
Code
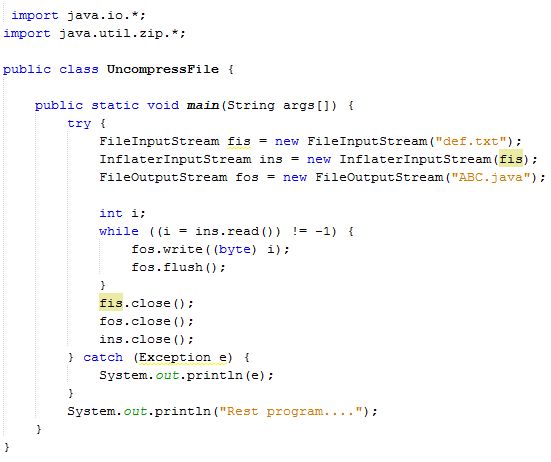
- import java.io.*;
- import java.util.zip.*;
- public class UncompressFile {
- public static void main(String args[]) {
- try {
- FileInputStream fis = new FileInputStream("def.txt");
- InflaterInputStream ins = new InflaterInputStream(fis);
- FileOutputStream fos = new FileOutputStream("ABC.java");
- int i;
- while ((i = ins.read()) != -1) {
- fos.write((byte) i);
- fos.flush();
- }
- fis.close();
- fos.close();
- ins.close();
- } catch (Exception e) {
- System.out.println(e);
- }
- System.out.println("Rest program....");
- }
- }
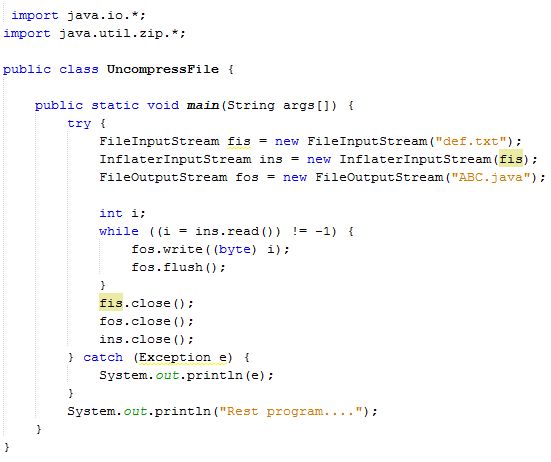
In the example, mentioned above, we uncompress the compressed file Java.txt into Simple.java.
Summary
Thus, we learnt that DeflaterOutputStream and InflaterInputStream classes are used to compress and uncompress the data in the deflate compression format and also learnt how to create it in Java.