Introduction Of AWT In Java
AWT
AWT stands for Abstract Windowing Toolkit. AWT is an API to develop GUI or Window-based Application in Java and its components are platform-dependent specifically components are displayed according to the view of an operating system. AWT is heavyweight that is to say its components use the resources of the system.
The java.awt package provides many classes for AWT API such as Label, TextArea, RadioButton, CheckBox, List etc.
AWT Hierarchy
The hierarchy of AWT classes is given below.
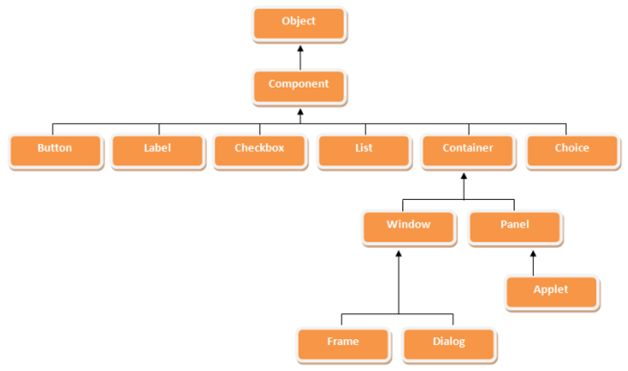
Container
The container is a component in AWT, which can hold many another components such as buttons, textfields, labels etc. The classes that extend container class are called as the container such as frame, dialog and panel.
Window
The Window is the container, which has no borders and menu bars. We should use frame, dialog or another Window to create a Window.
Panel
The panel is the container, which doesn't hold the title bar, menu bars and it can have other components such as button, textfield etc.
Frame
The frame is the container, which holds the title bar, menu bars and it can have other components such as button, textfield etc.
Methods of Component class
public void add(Component c)
This method is used to insert a component on the component.
public void setSize(int width,int height)
This method is used to set the size (width and height) of the component.
public void setLayout(LayoutManager m)
This method is used to define the layout manager for the component.
public void setVisible(boolean status)
This method is used to change the visibility of the component and by default, it is false.
To create AWT example, first we need a frame.
There are 2 ways to create a frame in AWT.
- Extending Frame class (inheritance)
- Creating the object of Frame class (association)
Let’s see an example of AWT through an inheritance.
Code
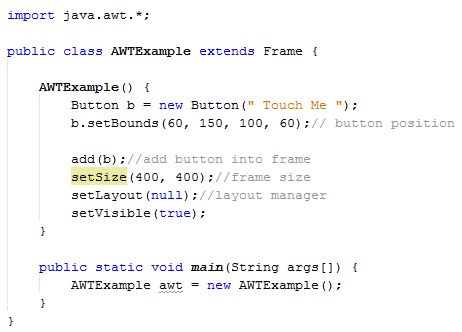
- import java.awt.*;
- public class AWTExample extends Frame {
- AWTExample() {
- Button b = new Button(" Touch Me ");
- b.setBounds(60, 150, 100, 60); // button position
- add(b); //add button into frame
- setSize(400, 400); //frame size
- setLayout(null); //layout manager
- setVisible(true);
- }
- public static void main(String args[]) {
- AWTExample awt = new AWTExample();
- }
- }
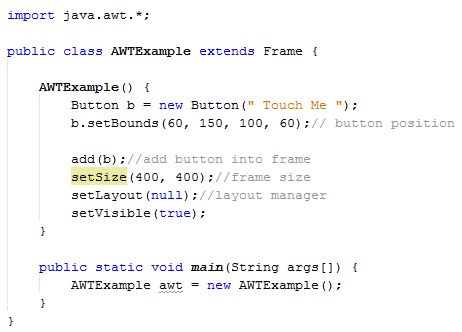
Output
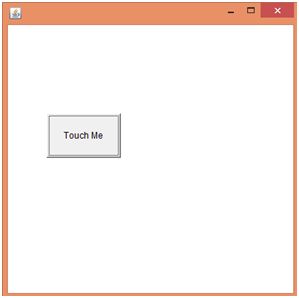
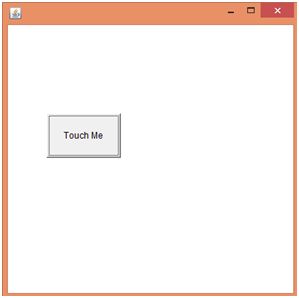
In the example, shown above, te setBounds(int xaxis, int yaxis, int width, int height) method is used to set the position of AWT button.
Let’s see an example of AWT through association.
Code
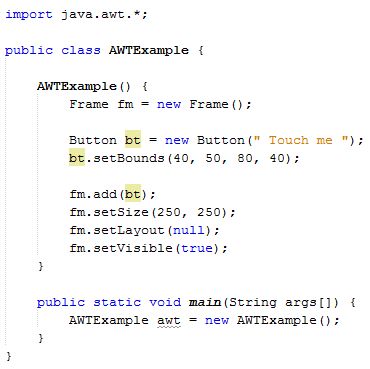
- import java.awt.*;
- public class AWTExample {
- AWTExample() {
- Frame fm = new Frame();
- Button bt = new Button(" Touch me ");
- bt.setBounds(40, 50, 80, 40);
- fm.add(bt);
- fm.setSize(250, 250);
- fm.setLayout(null);
- fm.setVisible(true);
- }
- public static void main(String args[]) {
- AWTExample awt = new AWTExample();
- }
- }
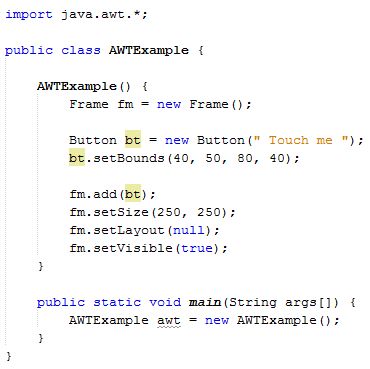
Output
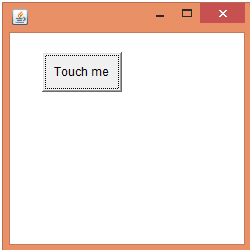
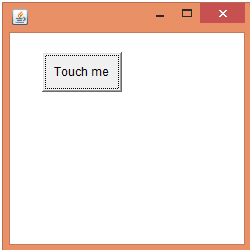
Summary
Thus, we learnt AWT stands for Abstract Windowing Toolkit. AWT is an API to develop GUI or Window-based Application in Java and also learnt how we can use it in Java.