How To Create A Thread In Java
Create a Thread
There are two ways to create a thread in Java multithreading, which are,
- By extending Thread class.
- By implementing Runnable interface.
Thread class in Java multithreading
Thread class provides many constructors and methods to create and perform many operations on a thread. Thread class extends an Object class and implements Runnable interface in Java multithreading.
Constructors of thread class
- Thread()
- Thread(String name)
- Thread(Runnable r)
- Thread(Runnable r, String name)
Methods of thread class
- public void run()
This method is used to perform a action for a thread. - public void start()
This method is used to start the execution of the thread. JVM calls the run () method on the thread. - public void sleep(long miliseconds)
This method causes the currently executing thread to sleep for the specified number of milliseconds. - public void join()
This method is used to wait for a thread to die. - public void join(long miliseconds)
This method is used to waits for a thread to die for the specified miliseconds. - public int getPriority()
This method is used to return the priority of the thread. - public int setPriority(int priority)
This method is used to change the priority of the thread. - public String getName()
This method is used to return the name of the thread. - public void setName(String name)
This method is used to change the name of the thread. - public Thread currentThread()
This method is used to return the reference of the currently executing thread. - public int getId()
This method is used to return the ID of the thread. - public Thread.State getState()
This method is used to return the state of the thread. - public boolean isAlive()
This method is used to test, if the thread is alive. - public void yield()
This method causes the currently executing thread object to temporarily pause and allow other threads to execute. - public void suspend()
This method is used to suspend the thread(depricated). - public void resume()
This method is used to resume the suspended thread(depricated). - public void stop()
This method is used to stop the thread(depricated). - public boolean isDaemon()
This method is used to test, if the thread is a daemon thread. - public void setDaemon(boolean b)
This method is used to mark the thread as daemon or user thread. - public void interrupt()
This method is used to interrupt the thread. - public boolean isInterrupted()
This method is used to test, if the thread has been interrupted. - public static boolean interrupted()
This method is used to test, if the current thread has been interrupted.
Runnable interface
The Runnable interface must be implemented by any class, whose instances are intended to be executed by a thread. Runnable interface have only one run() method.
public void run()
This method is used to perform an action for a thread.
How to Start a thread
This start() method is used to start a newly created thread.
It performs many tasks, which are given below.
- A new thread starts.
- The thread shifts from the new state to the Runnable state.
- If the thread gets a chance to execute, its target run() method will run.
Let’s see an example, given below by extending Thread class.
Code
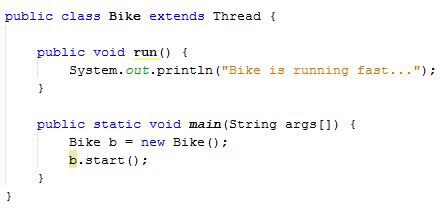
- public class Bike extends Thread {
- public void run() {
- System.out.println("Bike is running fast...");
- }
- public static void main(String args[]) {
- Bike b = new Bike();
- b.start();
- }
- }
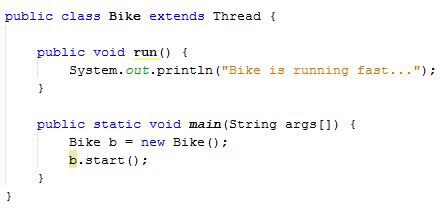
Output


In the example, given above, thread class constructor allocates a new thread object. When we create an object of Bike class, class constructor is called (provided by the compiler) from where thread class constructor is called (by super () as first statement). Thus, Bike class object is a thread object now.
Let’s see an example, given below, by implementing the Runnable interface.
Code
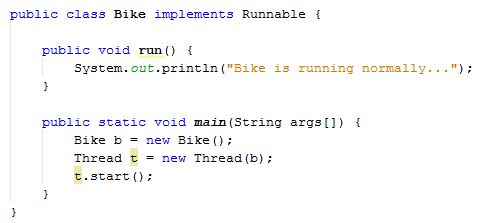
- public class Bike implements Runnable {
- public void run() {
- System.out.println("Bike is running normally...");
- }
- public static void main(String args[]) {
- Bike b = new Bike();
- Thread t = new Thread(b);
- t.start();
- }
- }
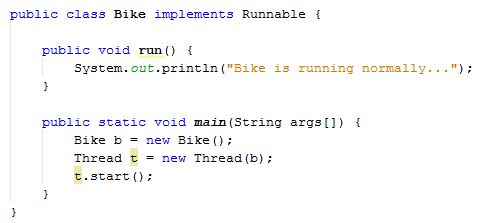
Output


In the example, given above, if we are not extending the thread class, the class object would not be treated as a thread object. Thus, we need to explicitly create Thread class object. We are passing the object of class, which implements Runnable. Thus, the class run () method may execute.
Summary
Thus, we learnt, there are two ways to create a thread in Java multithreading and also learnt some important constructors and methods of thread class.