For Loop In Java
Loop Control Statements In Java
Loop control statements are used when a code may either be executed a fixed number of times or while some condition is true. Java gives you a choice of three types of loop statements: while, do-while and for.
- while loop - The while loop keeps repeating an action until a related condition returns false. This is mainly useful, where the developer does not know in advance, how many times the loop will be run.
- do while loop- The do while loop is same as while but the condition is checked after the loop body is executed. This ensures that the loop body is run at least once.
- for loop- For loop is normally used, usually where the loop will be traversed a fixed number of times.
The for Loop
For statement makes it easier to count the iterations of a loop and works well, where the number of iterations of the loop is referred before the loop is entered.
Syntax
for(initialization; test condition; increment or decrement)
{
Statements;
}
The main purpose is to repeat the statement when the condition remains true. It is similar to while loop. In addition, for loop specifies an initialization instruction and an increment or decrement of the control variable instruction. Hence, this loop is specially designed to perform a repetitive action with a counter.
Let’s understand with the flowchart, given below.
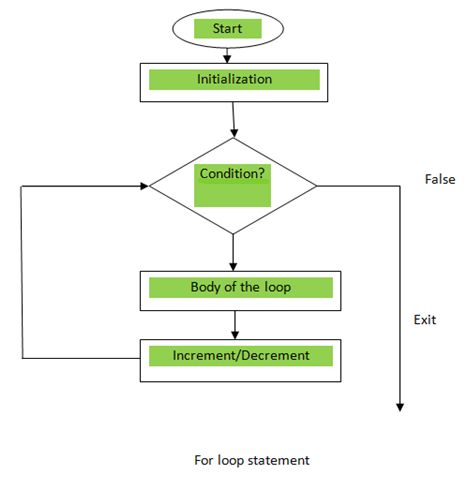
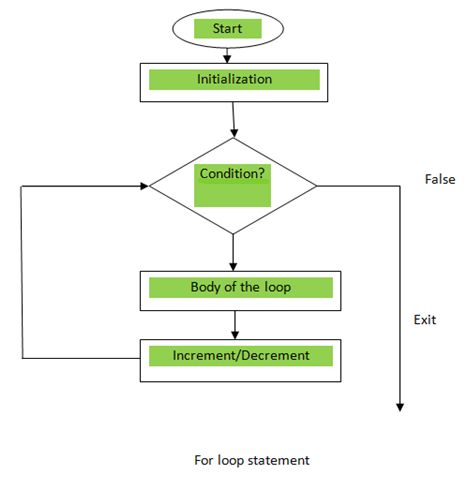
For loop is shown in the figure and works in the manner, given below.
- Initialization is executed. This is executed only once.
- Condition is checked. If it is true, the loop continues otherwise the loop exit and the statement is skipped.
- Statements are executed. It can be either a single instruction or a block of instructions enclosed within the curly brackets {}.
- Finally, the increment or decrement of the control variable field is executed and then the loop gets back to step2.
Let’s see an example, given below.
Code
- public class ForLoopExm {
- public static void main(String[] args) {
- for (int i = 1; i < 8; i++) {
- System.out.println("for loop : " + i);
- }
- }
- }
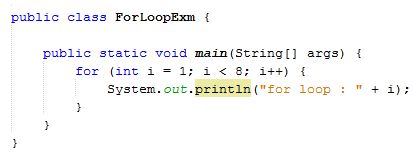
Output
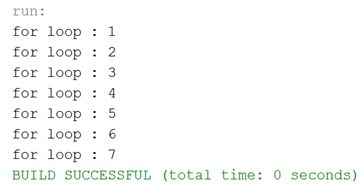
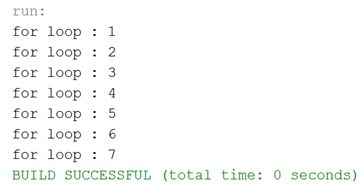
The three statements inside the braces of “for loop” generally meant for one activity each, however any of them can be left blank also. We can declare more than one control variables, which can be initialized but must be separated by the comma.
Various forms of loop statements are,
- for (;condition; increment/decrement)
Body;
A blank first statement means that no initialization. - for (initialization; condition;)
Body;
A blank last statement means no increment/decrement. - for (initialization; ; increment/decrement)
Body;
A second blank conditional statement means no test condition to control the exit from the loop. Hence, in the absence of the second statement, it is necessary to test the condition inside the loop otherwise it results in an infinite loop, where the control never exits. - for (; ; increment/decrement)
Body;
Initialization is necessary before the loop and test condition is checked inside the loop. - for (initialization ; ;)
Body;
Test condition and controls the variable increment/decrement, which is done inside the loop body. - for (; condition ;)
Body;
Initialization is necessary to be done before the loop and controls the variable increment/decrement, which is to be done inside the loop body. - for (; ; ;)
Body;
Initialization is necessary to be done before the loop, test condition and controls the variable increment/decrement, which is to be done inside the loop body.
Summary
Thus, we learnt that loop control statements are used when a code may either be executed a fixed number of times or while some condition is true and also learnt for loop with their various forms in Java.