Socket Programming In Java
Socket Programming
In Java, socket programming is used for communication between the Applications, running on the different JRE and it can be connection-oriented or connection-less.
Socket class and ServerSocket class are used for the connection-oriented socket programming and DatagramSocket class and DatagramPacket class are used for the connection-less socket programming.
In socket programming, the client have to know: IP Address of Server and Port number.
Socket class
A socket is simply an endpoint for connections between the machines. The Socket class can be used to create a socket.
Methods of Socket class
public InputStream getInputStream()
This method is used to return the InputStream attached with the socket.
public OutputStream getOutputStream()
This method is used to return the OutputStream attached with the socket.
public synchronized void close()
This method is used to close the socket
ServerSocket class
The ServerSocket class can be used to make a Server socket and this object is used to establish the communication with the clients.
Methods of ServerSocket class
public Socket accept()
This method is used to return the socket and establish a connection between the Server and client.
public synchronized void close()
This method is used to close the Server socket.
Let's see an example of socket programming,, given below.
Code
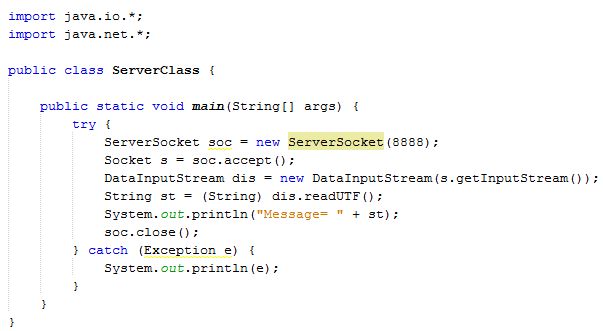
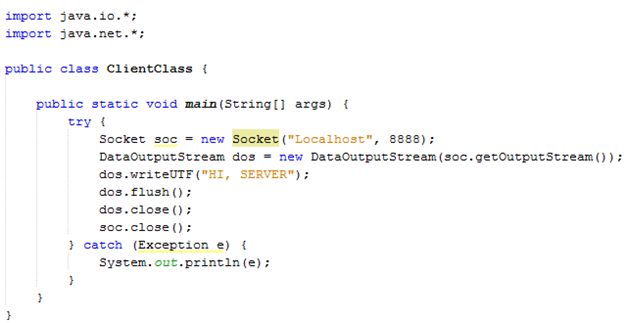
- import java.io.*;
- import java.net.*;
- public class ServerClass {
- public static void main(String[] args) {
- try {
- ServerSocket soc = new ServerSocket(8888);
- Socket s = soc.accept();
- DataInputStream dis = new DataInputStream(s.getInputStream());
- String st = (String) dis.readUTF();
- System.out.println("Message= " + st);
- soc.close();
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
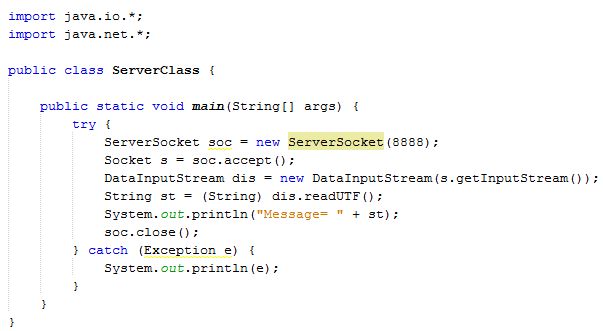
- import java.io.*;
- import java.net.*;
- public class ClientClass {
- public static void main(String[] args) {
- try {
- Socket soc = new Socket("Localhost", 8888);
- DataOutputStream dos = new DataOutputStream(soc.getOutputStream());
- dos.writeUTF("HI, SERVER");
- dos.flush();
- dos.close();
- soc.close();
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
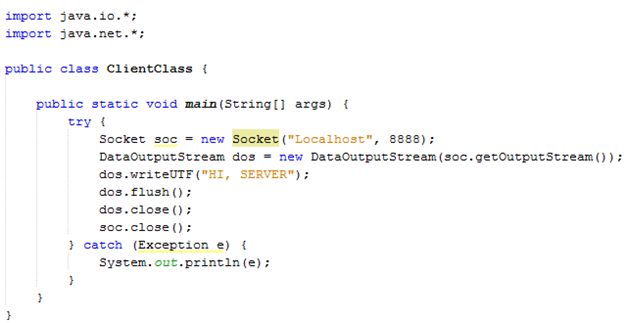
In the example of socket programming, given below, where the client sends a text and the Server receives it. After running the client Application message will be displayed on the Server console.
Let’s see another example of socket programming, given below.
Code
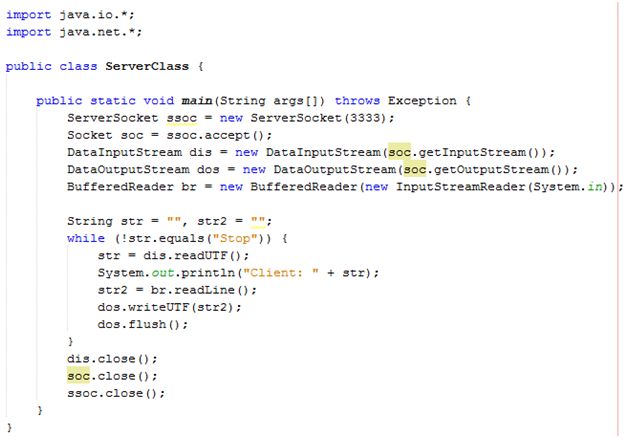
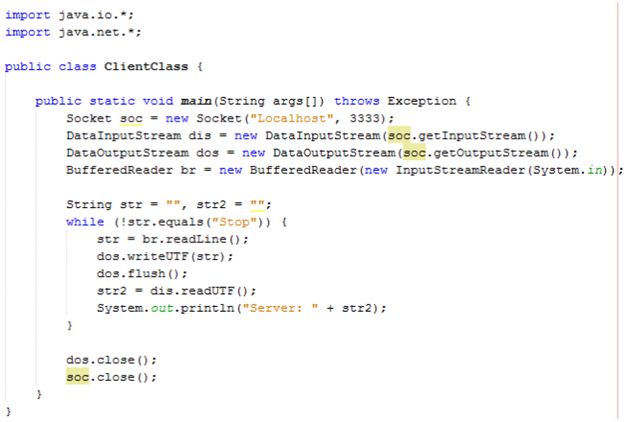
- import java.io.*;
- import java.net.*;
- public class ServerClass {
- public static void main(String args[]) throws Exception {
- ServerSocket ssoc = new ServerSocket(3333);
- Socket soc = ssoc.accept();
- DataInputStream dis = new DataInputStream(soc.getInputStream());
- DataOutputStream dos = new DataOutputStream(soc.getOutputStream());
- BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
- String str = "", str2 = "";
- while (!str.equals("Stop")) {
- str = dis.readUTF();
- System.out.println("Client: " + str);
- str2 = br.readLine();
- dos.writeUTF(str2);
- dos.flush();
- }
- dis.close();
- soc.close();
- ssoc.close();
- }
- }
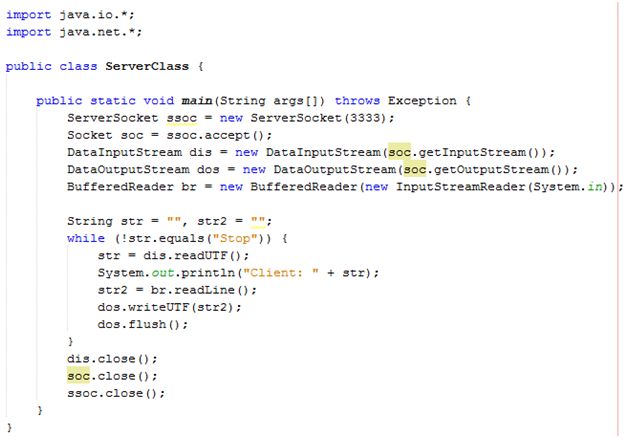
- import java.io.*;
- import java.net.*;
- public class ClientClass {
- public static void main(String args[]) throws Exception {
- Socket soc = new Socket("Localhost", 3333);
- DataInputStream dis = new DataInputStream(soc.getInputStream());
- DataOutputStream dos = new DataOutputStream(soc.getOutputStream());
- BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
- String str = "", str2 = "";
- while (!str.equals("Stop")) {
- str = br.readLine();
- dos.writeUTF(str);
- dos.flush();
- str2 = dis.readUTF();
- System.out.println("Server: " + str2);
- }
- dos.close();
- soc.close();
- }
- }
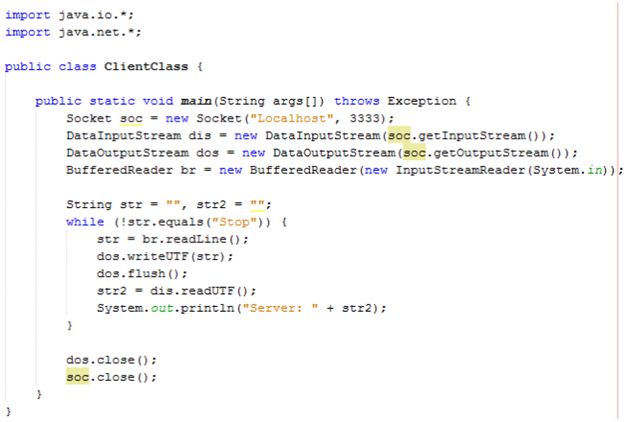
In the example, mentioned above, the client will write first to the Server then the Server will receive and print the text. The Server will also write to the client, client will receive, print the text and step goes on.
Summary
Thus, we learnt Java socket programming is used for the communication between the Applications, running on different JRE and it can be connection-oriented or connection-less and also learnt how we can use it in Java.