Functional Interfaces In Java
Functional Interfaces
Functional interfaces have a single functionality to show. Such as, a Comparable interface has a single method ‘compareTo’ only and it is used for comparison purpose.
Java 8 has defined many functional interfaces to be used widely in lambda expressions.
Functional interfaces that are define in java.util.Function package
Interfaces
BiConsumer<T,U>
This interface represents an operation which accepts two input arguments.
BiFunction<T,U,R>
This interface represents a function which accepts two arguments and returns a result.
BinaryOperator<T>
This interface represents an operation upon two operands of the same type and returns a result of the same type as the operands.
BiPredicate<T,U>
This interface represents a predicate of two arguments.
BooleanSupplier
This interface represents a supplier of Boolean-valued results.
Consumer<T>
This interface represents an operation which accepts a single input argument.
DoubleBinaryOperator
This interface represents an operation upon two double-valued operands and returns a double-valued result.
DoubleConsumer
This interface represents an operation which accepts a single double-valued argument.
DoubleFunction<R>
This interface represents a function which accepts a double-valued argument and returns a result.
DoublePredicate
This interface represents a predicate of one double-valued argument.
DoubleSupplier
This interface represents a supplier of double-valued results.
DoubleToIntFunction
This interface represents a function which accepts a double-valued argument and returns an int-valued result.
DoubleToLongFunction
This interface represents a function which accepts a double-valued argument and returns a long-valued result.
DoubleUnaryOperator
This interface represents an operation on a single double-valued operand which returns a double-valued result.
Function<T,R>
This interface represents a function which accepts one argument and returns a result.
IntBinaryOperator
This interface represents an operation upon two int-valued operands and returns an int-valued result.
IntConsumer
This interface represents an operation which accepts a single int-valued argument.
IntFunction<R>
This interface represents a function which accepts an int-valued argument and returns a result.
IntPredicate
This interface represents a predicate of one int-valued argument.
IntSupplier
This interface represents a supplier of int-valued results.
IntToDoubleFunction
This interface represents a function which accepts an int-valued argument and returns a double-valued result.
IntToLongFunction
This interface represents a function which accepts an int-valued argument and returns a long-valued result.
IntUnaryOperator
This interface represents an operation on a single int-valued operand that returns an int-valued result.
LongBinaryOperator
This interface represents an operation upon two long-valued operands and returns a long-valued result.
LongConsumer
This interface represents an operation which accepts a single long-valued argument.
LongFunction<R>
This interface represents a function which accepts a long-valued argument and returns a result.
LongPredicate
This interface represents a predicate of one long-valued argument.
LongSupplier
This interface represents a supplier of long-valued results.
LongToDoubleFunction
This interface represents a function which accepts a long-valued argument and returns a double-valued result.
LongToIntFunction
This interface represents a function which accepts a long-valued argument and returns an int-valued result.
LongUnaryOperator
This interface represents an operation on a single long-valued operand that returns a long-valued result.
ObjDoubleConsumer<T>
This interface represents an operation which accepts an object-valued and a double-valued argument.
ObjIntConsumer<T>
This interface represents an operation which accepts an object-valued and an int-valued argument.
ObjLongConsumer<T>
This interface represents an operation which accepts an object-valued and a long-valued argument.
Predicate<T>
This interface represents a predicate of one argument.
Supplier<T>
This interface represents a supplier of results.
ToDoubleBiFunction<T,U>
This interface represents a function which accepts two arguments and returns a double-valued result.
ToDoubleFunction<T>
This interface represents a function which returns a double-valued result.
ToIntBiFunction<T,U>
This interface represents a function which accepts two arguments and returns an int-valued result.
ToIntFunction<T>
This interface represents a function which returns an int-valued result.
ToLongBiFunction<T,U>
This interface represents a function which accepts two arguments and returns a long-valued result.
ToLongFunction<T>
This interface represents a function which returns a long-valued result.
UnaryOperator<T>
This interface represents an operation on a single operand which returns a result of the same type as its operand.
Let’s see an example of Functional Interface.
Code
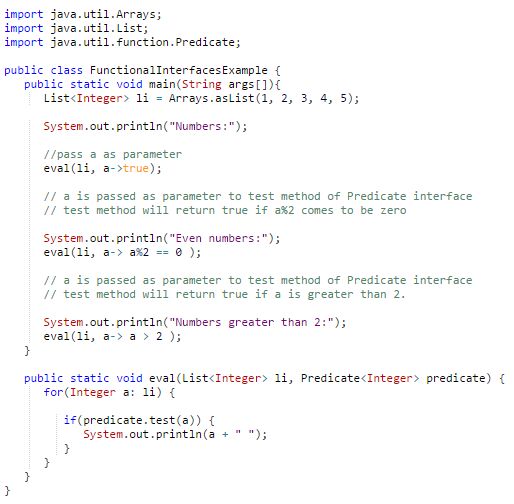
- import java.util.Arrays;
- import java.util.List;
- import java.util.function.Predicate;
- public class FunctionalInterfacesExample {
- public static void main(String args[]){
- List<Integer> li = Arrays.asList(1, 2, 3, 4, 5);
- System.out.println("Numbers:");
- //pass a as parameter
- eval(li, a->true);
- // a is passed as parameter to test method of Predicate interface
- // test method will return true if a%2 comes to be zero
- System.out.println("Even numbers:");
- eval(li, a-> a%2 == 0 );
- // a is passed as parameter to test method of Predicate interface
- // test method will return true if a is greater than 2.
- System.out.println("Numbers greater than 2:");
- eval(li, a-> a > 2 );
- }
- public static void eval(List<Integer> li, Predicate<Integer> predicate) {
- for(Integer a: li) {
- if(predicate.test(a)) {
- System.out.println(a + " ");
- }
- }
- }
- }
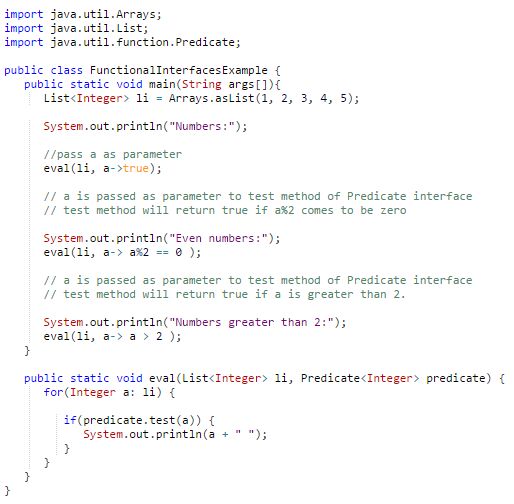
Output
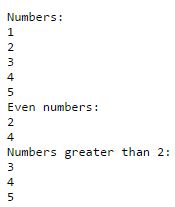
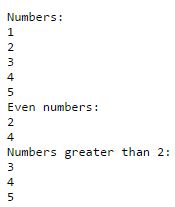
In the above example, we pass Predicate interface that takes a single input and returns Boolean. Predicate <T> interface is a functional interface and a method test(Object) to return a Boolean value. This interface shows that an object is tested to be true or false.
Summary
Thus, we learned that Functional interfaces have a single functionality to show and Java 8 has defined many functional interfaces to be used widely in lambda expressions and also learns how to use it in Java.