Inter-Thread Communication In Java
Inter-Thread Communication
In Java, Inter-thread communication is allowing synchronized threads to communicate with each other. It is also called as a co-operation. Inter-thread communication is a mechanism, where a thread is paused , running in its critical section and another thread is permitted to enter in the same critical section to be executed.
Inter-thread communication is implemented by following methods of Object class, given below.
- wait() method
wait() method causes current thread to free the lock and wait until either another thread calls the notify() method or the notifyAll() method for the object or a particular amount of time has elapsed.
The current thread must own the object's monitor. Thus, it must be called from the synchronized method only else it will throw an exception.
Method
public final void wait()throws InterruptedException
This method is used to wait until the object is notified.
public final void wait(long timeout)throws InterruptedException
This method is used to wait for the specified amount of time.
- notify() method
notify() method wakes up a single thread that is waiting on the object's monitor. If any threads are waiting on the object, one of them is chosen to be awakened. The choice is random and occurs at the discretion of the implementation.
Syntax
public final void notify()
- notifyAll() method
notifyAll() method wakes up all the threads, which are waiting on this object's monitor.
Syntax
public final void notifyAll()
Process of inter-thread communication.
- Threads enter to obtain lock.
- Lock is obtained by the thread.
- The thread goes to waiting state, if we call wait() method on the object else it releases the lock and exits.
- If we call notify() or notifyAll() method, thread moves to the notified state or runnable state.
- Now, the thread is available to obtain the lock.
- After completion of the task, thread frees the lock and exits the monitor state of the object.
wait() method , notify() method and notifyAll() method are defined in Object class and not in Thread class because they are related to the lock and object has a lock.
Difference between wait and sleep methods
Let's see the difference between wait and sleep methods.
wait() Method | sleep() Method |
wait() method releases the lock. | sleep() method doesn't release the lock. |
wait() method is the method of Object class. | sleep() method is the method of Thread class. |
wait() method is the non-static method. | sleep() method is the static method. |
This method should be notified by notify(), notifyAll() methods. | After the particular amount of time, sleep is completed. |
Let's see an example of inter thread communication.
Code
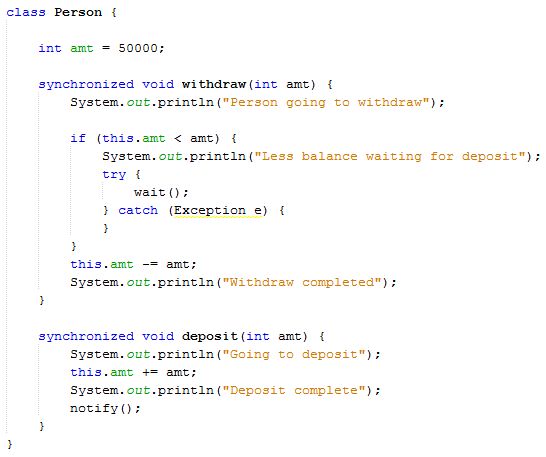
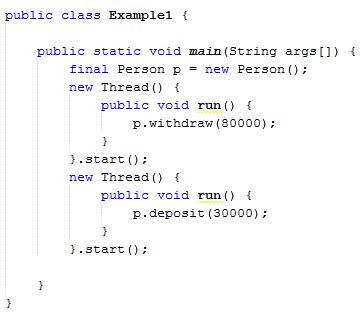
- class Person {
- int amt = 50000;
- synchronized void withdraw(int amt) {
- System.out.println("Person going to withdraw");
- if (this.amt < amt) {
- System.out.println("Less balance waiting for deposit");
- try {
- wait();
- } catch (Exception e) {}
- }
- this.amt -= amt;
- System.out.println("Withdraw completed");
- }
- synchronized void deposit(int amt) {
- System.out.println("Going to deposit");
- this.amt += amt;
- System.out.println("Deposit complete");
- notify();
- }
- }
- public class Example1 {
- public static void main(String args[]) {
- final Person p = new Person();
- new Thread() {
- public void run() {
- p.withdraw(80000);
- }
- }.start();
- new Thread() {
- public void run() {
- p.deposit(30000);
- }
- }.start();
- }
- }
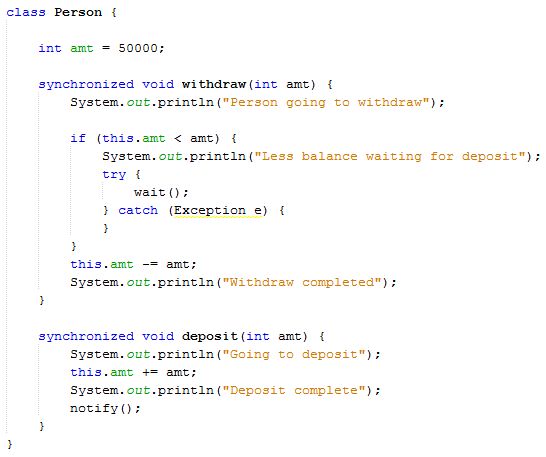
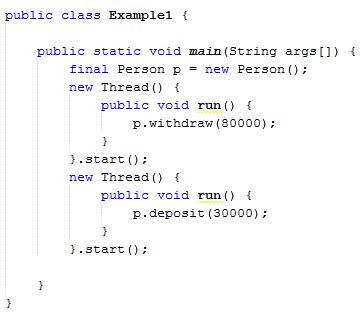
Output
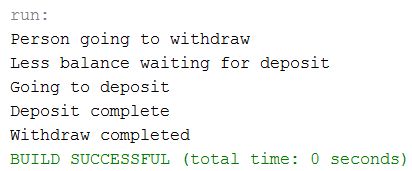
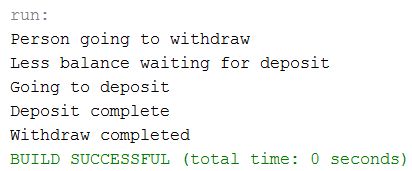
Summary
Thus, we learnt Java Inter-thread communication is allowing synchronized threads to communicate with each other. It is also called as a co-operation and also learnt process of inter-thread communication in Java.