Scanner Class In Java
Scanner Class
The java.util.Scanner class is also used to read the input from the keyboard.
By default, Scanner class breaks the input into the tokens, using a delimiter that is whitespace.
Scanner class provides the various methods to read and parse various primitive values. It is generally used to parse the text for the string and primitive types, using regular expression. This class extends Object class and implements Iterator interface and Closeable interface.
A scanning operation may block waiting for the input and Scanner class is not safe for the multithreaded use without an external synchronization.
Methods of Scanner class
public String next()
This method is used to return the next token from the scanner.
public String nextLine()
This method is used to return the scanner position to the next line and returns the value as a string.
public byte nextByte()
It scans the next token as a byte.
public short nextShort()
It scans the next token as a short value.
public int nextInt()
It scans the next token as an int value.
public long nextLong()
It scans the next token as a long value.
public float nextFloat()
It scans the next token as a float value.
public double nextDouble()
It scans the next token as a double value.
Let’s see an example, given below.
Code
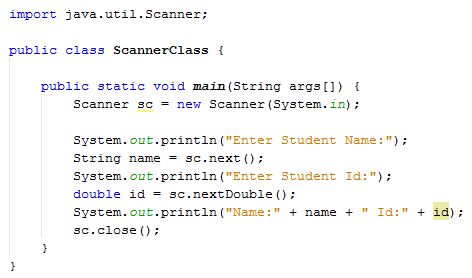
Output
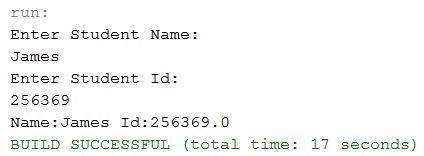
- import java.util.Scanner;
- public class ScannerClass {
- public static void main(String args[]) {
- Scanner sc = new Scanner(System.in);
- System.out.println("Enter Student Name:");
- String name = sc.next();
- System.out.println("Enter Student Id:");
- double id = sc.nextDouble();
- System.out.println("Name:" + name + " Id:" + id);
- sc.close();
- }
- }
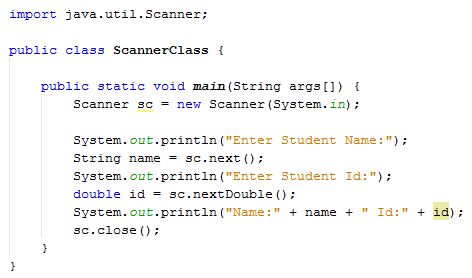
Output
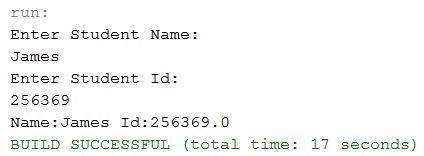
In the example, shown above, we create a Scanner class that reads the int, string and double value as an input.
Let’s see another example, given below, Scanner class with delimiter.
Code
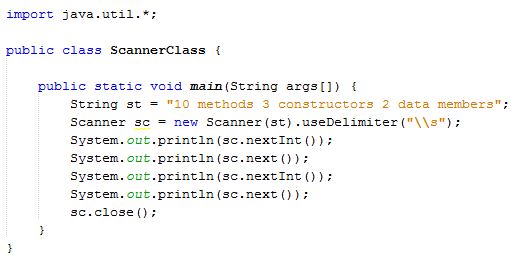
- import java.util.*;
- public class ScannerClass {
- public static void main(String args[]) {
- String st = "10 methods 3 constructors 2 data members";
- Scanner sc = new Scanner(st).useDelimiter("\\s");
- System.out.println(sc.nextInt());
- System.out.println(sc.next());
- System.out.println(sc.nextInt());
- System.out.println(sc.next());
- sc.close();
- }
- }
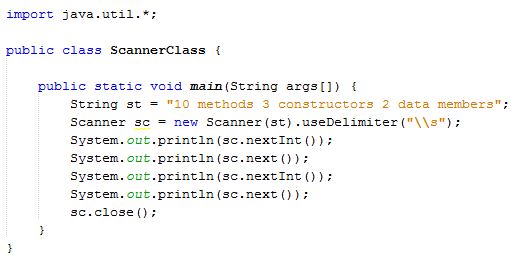
Output
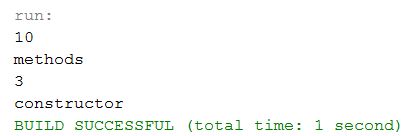
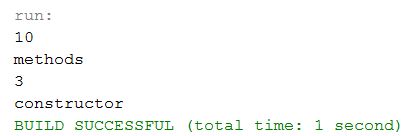
In the example, we can observe that scanner class breaks the input into tokens using a delimiter that is whitespace.
Summary
Thus, we learnt that java.util.Scanner class is also used to read the input from the keyboard and also learnt its important methods in Java.