Break Statement In Java
Break Statement
Sometimes, it is necessary to jump out of a loop irrespective of the conditional test value. Break statement is used inside any loop to allow the control jump to the instant statement, following the loop.
Syntax
break;
When we use nested loop, break jumps the control from the loop, where it has been used. Break statement can be used inside any loop i.e. while, do while, for and switch statement.
Let’s understand with the flowchart, given below.
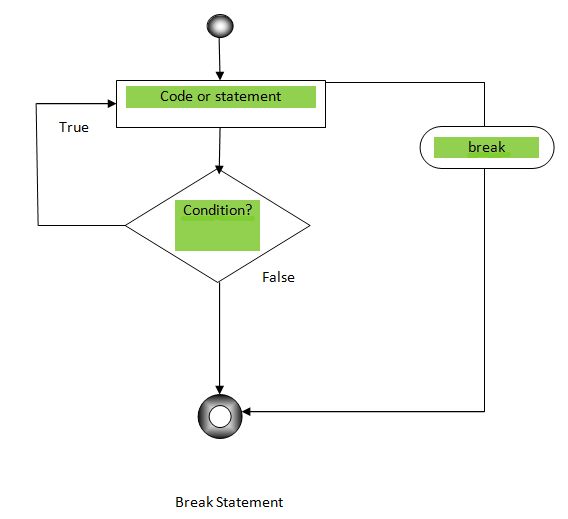
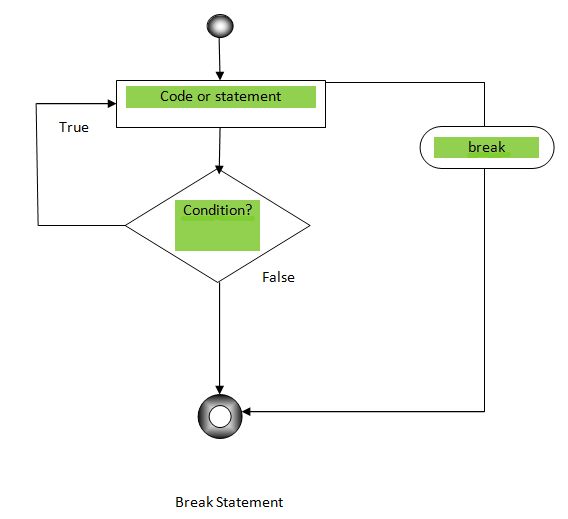
Break statement is different from exit. Break jumps the control out of the loop and exit stops the execution of the entire program.
Let’s see an example, given below.
Code
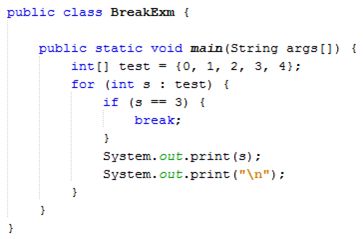
- public class BreakExm {
- public static void main(String args[]) {
- int[] test = {0, 1, 2, 3, 4};
- for (int s : test) {
- if (s == 3) {
- break;
- }
- System.out.print(s);
- System.out.print("\n");
- }
- }
- }
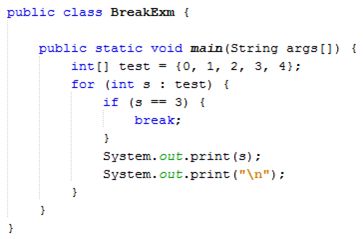
Output
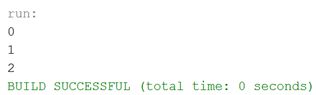
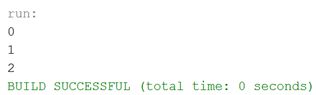
Break statement with nested loop.
It breaks nested loop only if we use break statement inside the nested loop.
The example of nested loop is given by,
Code
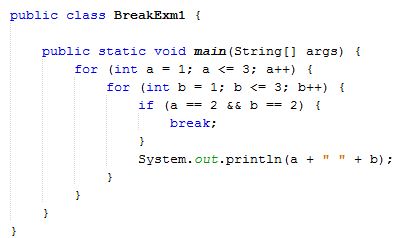
- public class BreakExm1 {
- public static void main(String[] args) {
- for (int a = 1; a <= 3; a++) {
- for (int b = 1; b <= 3; b++) {
- if (a == 2 && b == 2) {
- break;
- }
- System.out.println(a + " " + b);
- }
- }
- }
- }
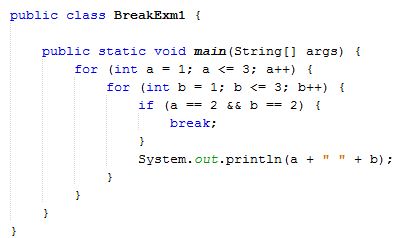
Output
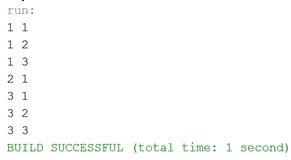
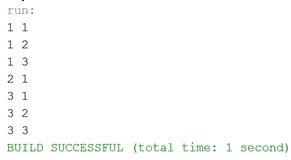
Summary
Thus, we learnt that Break statement is used inside any loop to allow the control jump to the instant statement, following the loop and also learnt how to use it in Java.