Runtime Class In Java
Runtime Class
Runtime class is mainly used to interact with Java runtime environment. It provides methods to execute a process, invoke GC, get total and free memory etc. There is only one object of java.lang.Runtime class, which is available for one Java Application.
Runtime.getRuntime() method is used to return the singleton instance of Runtime class.
Methods of Java Runtime class are given below.
public static Runtime getRuntime()
This method is used to return the instance of Runtime class.
public void exit(int status)
This method is used to terminate the current virtual machine.
public void addShutdownHook(Thread hook)
This method is used to register new hook thread.
public Process exec(String command)throws IOException
This method is used to execute the given command in a separate process.
public int availableProcessors()
This method is used to return the no. of available processors.
public long freeMemory()
This method is used to return the amount of free memory in JVM.
public long totalMemory()
This method is used to return the amount of total memory in JVM.
Let’s see some methods of Runtime class with the example, given below.
Runtime exec() method
Code
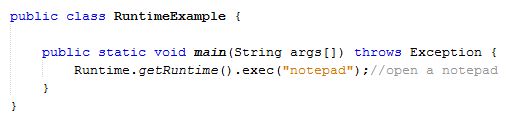
- public class RuntimeExample {
- public static void main(String args[]) throws Exception {
- Runtime.getRuntime().exec("notepad"); //open a notepad
- }
- }
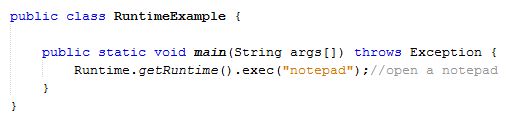
Output
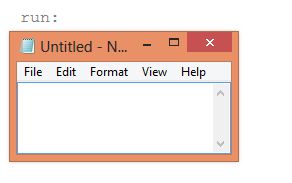
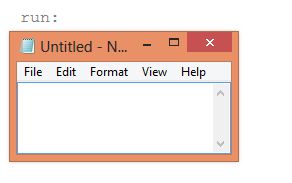
How to shutdown the system
We use shutdown -s command to shutdown the system. For windows OS, we need to give full path of shutdown command like c:\\Windows\\System32\\shutdown.
- Use -s switch to shutdown the system
- Use -r switch to restart the system
- Use -t switch to specify the time delay.
Code
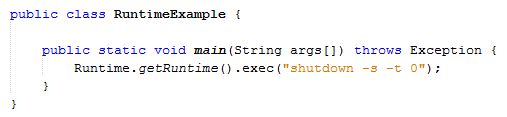
- public class RuntimeExample {
- public static void main(String args[]) throws Exception {
- Runtime.getRuntime().exec("shutdown -s -t 0");
- }
- }
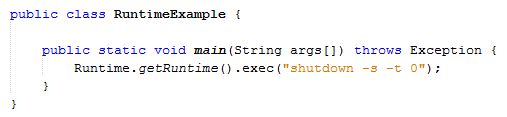
How to shutdown Windows system
Code

- public class RuntimeExample {
- public static void main(String args[]) throws Exception {
- Runtime.getRuntime().exec("c:\\Windows\\System32\\shutdown -s -t 0");
- }
- }

How to restart the system
Code
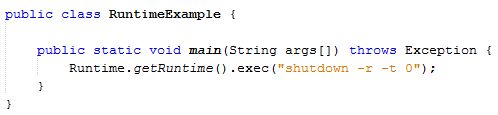
- public class RuntimeExample {
- public static void main(String args[]) throws Exception {
- Runtime.getRuntime().exec("shutdown -r -t 0");
- }
- }
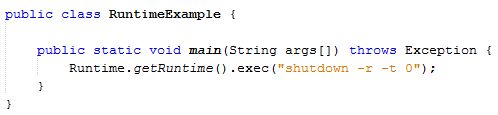
Runtime availableProcessors() method
Code
- public class RuntimeExample {
- public static void main(String args[]) throws Exception {
- System.out.println(Runtime.getRuntime().availableProcessors());
- }
- }
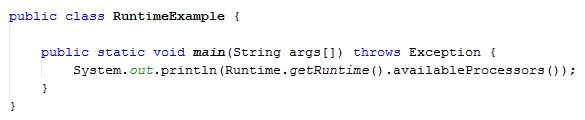
Runtime freeMemory() and totalMemory() method
Code
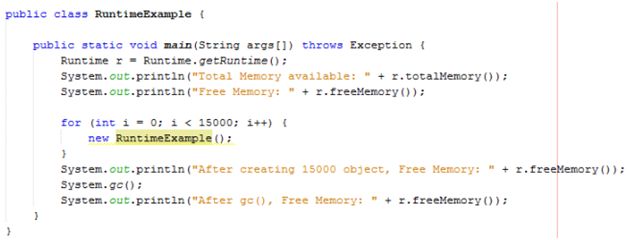
- public class RuntimeExample {
- public static void main(String args[]) throws Exception {
- Runtime r = Runtime.getRuntime();
- System.out.println("Total Memory available: " + r.totalMemory());
- System.out.println("Free Memory: " + r.freeMemory());
- for (int i = 0; i < 15000; i++) {
- new RuntimeExample();
- }
- System.out.println("After creating 15000 objects, Free Memory: " + r.freeMemory());
- System.gc();
- System.out.println("After gc(), Free Memory: " + r.freeMemory());
- }
- }
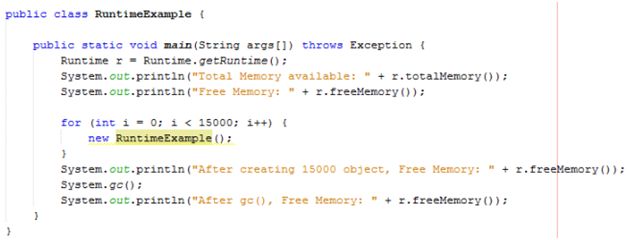
Output
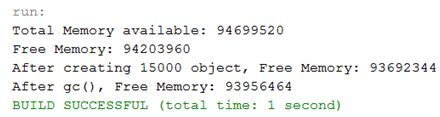
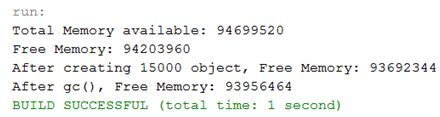
In the example, mentioned above, we have seen that after creating 15000 objects, free memory will be less than the previous free memory. After gc() call, we will get more free memory.
Summary
Thus, we learnt, Runtime class is mainly used to interact with Java runtime environment and also learnt its important methods in Java.