Reentrant Monitor In Java
Reentrant Monitor
In Java, monitors are reentrant which means Java, thread can reuse the same monitor for different synchronized methods, but the method is called from the method.
Advantage of Reentrant Monitor
Reentrant monitor eliminates the possibility of the single thread deadlocking.
Let's see an example of the reentrant monitor, given below.
Code
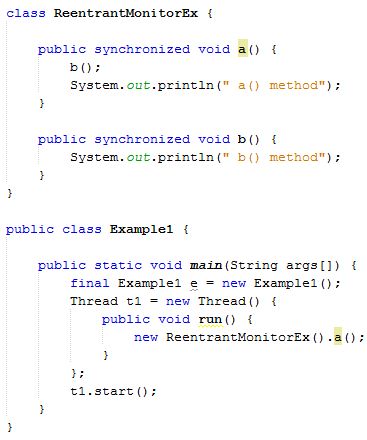
- class ReentrantMonitorEx {
- public synchronized void a() {
- b();
- System.out.println(" a() method");
- }
- public synchronized void b() {
- System.out.println(" b() method");
- }
- }
- public class Example1 {
- public static void main(String args[]) {
- final Example1 e = new Example1();
- Thread t1 = new Thread() {
- public void run() {
- new ReentrantMonitorEx().a();
- }
- };
- t1.start();
- }
- }
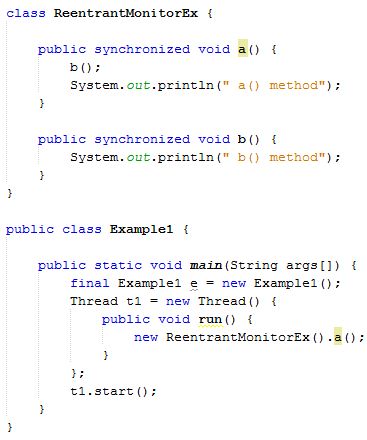
Output
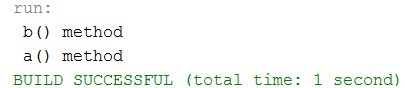
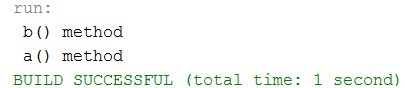
In the example, mentioned above, a and b are the synchronized methods. The a() method internally calls the b() method and we create thread, using annonymous class to call the a() method on a thread.
Summary
Thus, we learnt that Reentrant monitor eliminates the possibility of a single thread deadlocking and also learnt how to create it in Java.