Switch Statement In Java
Switch statement
Java switch statement is also a control flow statement. It is used when we want to be tested for equality against a list of the values. Switch statement returns one statement from the multiple conditions.
Syntax
switch(expression){
case value1:
// code.
break; //it is not compulsory(Optional).
case value2;
// code.
break;
.
.
default:
// code..
}
Let’s understand with the flowchart, given below.
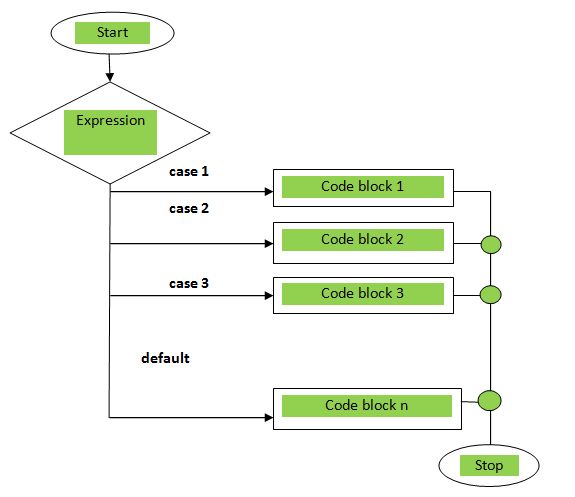
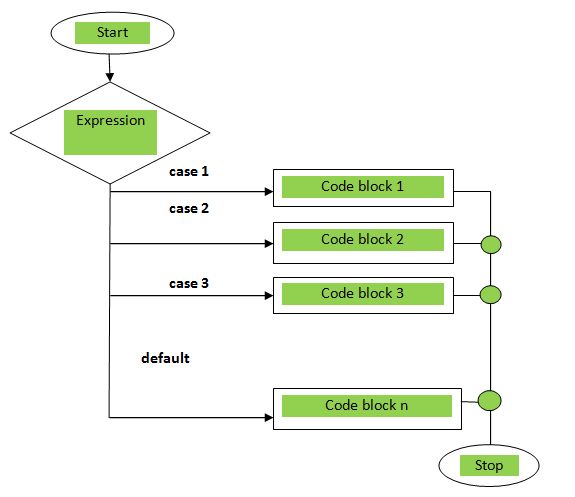
Let’s see an example, given below.
Code
- public class SwitchStatement {
- public static void main(String args[]) {
- char grade = 'C';
- switch (grade) {
- case 'A':
- System.out.println("Excellent");
- break;
- case 'B':
- System.out.println("Very Good");
- break;
- case 'C':
- System.out.println("Good");
- break;
- case 'D':
- System.out.println("Passed");
- case 'F':
- System.out.println("Better try again");
- break;
- default:
- System.out.println("Invalid ");
- }
- System.out.println("Your grade is " + grade);
- }
- }
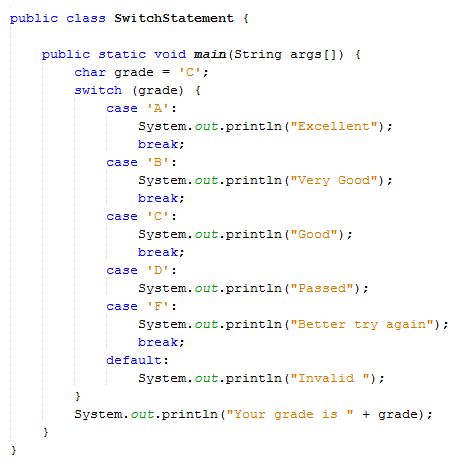
Output
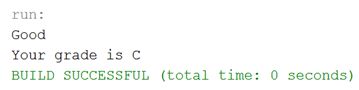
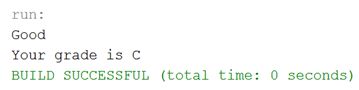
Switch Statement if fall-through
This switch statement if fall-through is used when we want to return all the statement after first matching case and if break statement is not used with the cases.
For example.
Code
- public class SwitchStatement2 {
- public static void main(String[] args) {
- char letter = 'B';
- switch (letter) {
- case 'A':
- System.out.println("A");
- case 'B':
- System.out.println("B");
- case 'C':
- System.out.println("C");
- default:
- System.out.println("Not in A, B or C");
- }
- }
- }
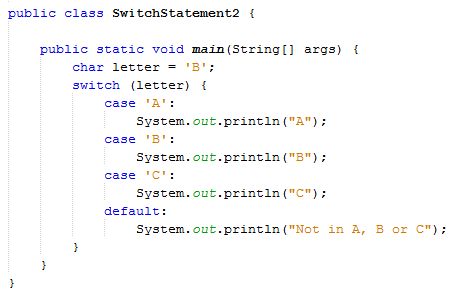
Output
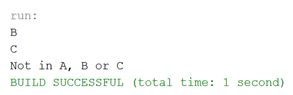
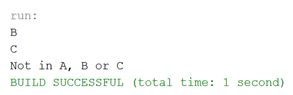
Summary
Thus, we learnt “switch statement” is used when we want to be tested for the equality against a list of the values and also learnt how to use it in Java.