How To Read Data From Keyboard In Java
Read data from keyboard
There are several ways to read data from the keyboard in Java.
Such as,
- InputStreamReader
- Console
- Scanner
- DataInputStream
InputStreamReader class
InputStreamReader class can used to read the data from the keyboard in Java.
It performs mainly two tasks, which are,
- Connects to the input stream of the keyboard.
- Converts the byte-oriented stream into the character-oriented stream.
BufferedReader class
BufferedReader class can be used to read the data one line by line through readLine() method.
Let’s see an example, given below.
Read the data from the keyboard by InputStreamReader and BufferdReader class.
Code
- import java.io.*;
- public class FileHandlingExample {
- public static void main(String args[]) throws Exception {
- InputStreamReader isr = new InputStreamReader(System.in);
- BufferedReader br = new BufferedReader(isr);
- System.out.println("Please enter your name: ");
- String name = br.readLine();
- System.out.println("Hello, " + name);
- }
- }
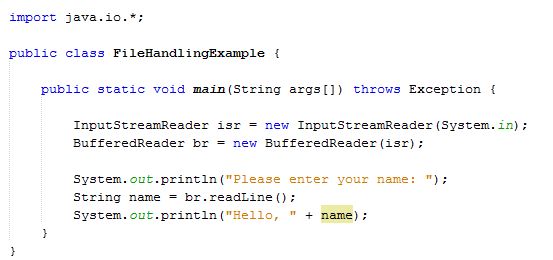
Output
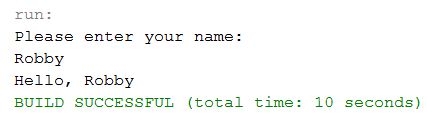
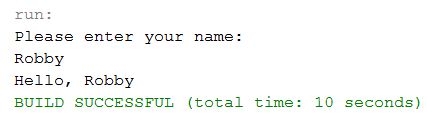
In the example, mentioned above, we connect the BufferedReader stream with the InputStreamReader stream to read the line by line data from the keyboard.
Let’s see another example, given below.
Read data from the keyboard by InputStreamReader and BufferdReader class, awaiting the user writes End.
Code
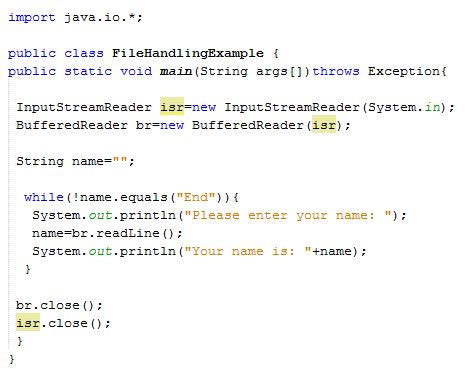
- import java.io.*;
- public class FileHandlingExample {
- public static void main(String args[]) throws Exception {
- InputStreamReader isr = new InputStreamReader(System.in);
- BufferedReader br = new BufferedReader(isr);
- String name = "";
- while (!name.equals("End")) {
- System.out.println("Please enter your name: ");
- name = br.readLine();
- System.out.println("Your name is: " + name);
- }
- br.close();
- isr.close();
- }
- }
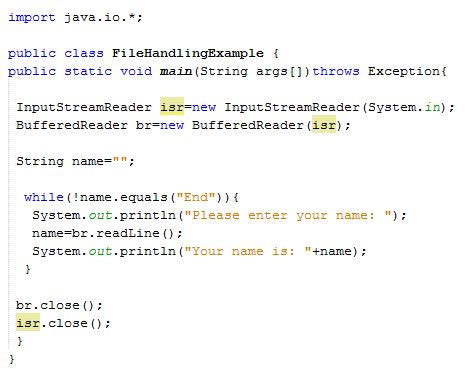
Output
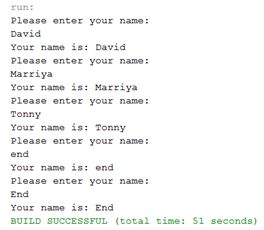
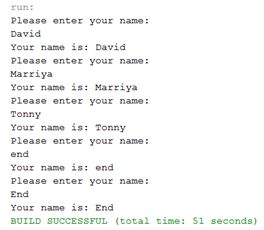
In the example, shown above, we read and print the data until the user prints End.
Summary
Thus, we learnt that InputStreamReader class can be used to read the data from the keyboard and BufferedReader class can be used to read the data line by line through readLine() method in Java and also learn how to create it.