JButton Class In Java Swing
JButton Class
In Java Swing, JButton class creates a button, which has a platform-independent implementation and JButton class extends AbstractButton class.
Constructors
JButton()
This constructor creates a button with no text and icon.
JButton(String s)
This constructor creates a button with the particular text.
JButton(Icon i)
This constructor creates a button with the particular icon object.
Methods of AbstractButton class
public void setText(String s)
This method is used to set the specific text on the button.
public String getText()
This method is used to return the text of the button.
public void setEnabled(boolean b)
This method is used to enable or disable the button.
public void setIcon(Icon b)
This method is used to set the specific Icon on the button.
public Icon getIcon()
This method is used to get the icon of the button.
public void setMnemonic(int a)
This method is used to set the mnemonic on the button.
public void addActionListener(ActionListener a)
This method is used to add the action listener to the object.
Let’s see an example of JButton class, given below.
Code
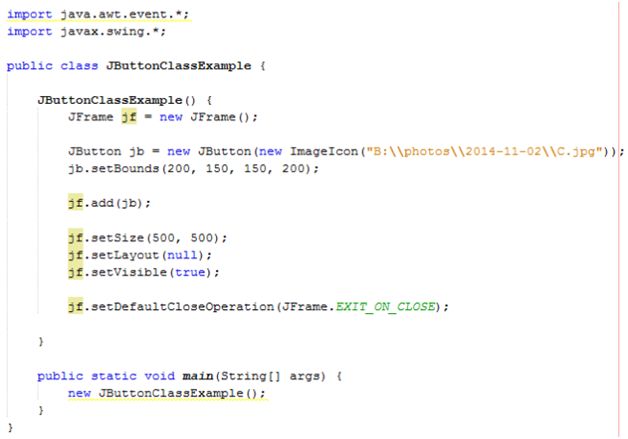
- import java.awt.event.*;
- import javax.swing.*;
- public class JButtonClassExample {
- JButtonClassExample() {
- JFrame jf = new JFrame();
- JButton jb = new JButton(new ImageIcon("B:\\photos\\2014-11-02\\C.jpg"));
- jb.setBounds(200, 150, 150, 200);
- jf.add(jb);
- jf.setSize(500, 500);
- jf.setLayout(null);
- jf.setVisible(true);
- jf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
- }
- public static void main(String[] args) {
- new JButtonClassExample();
- }
- }
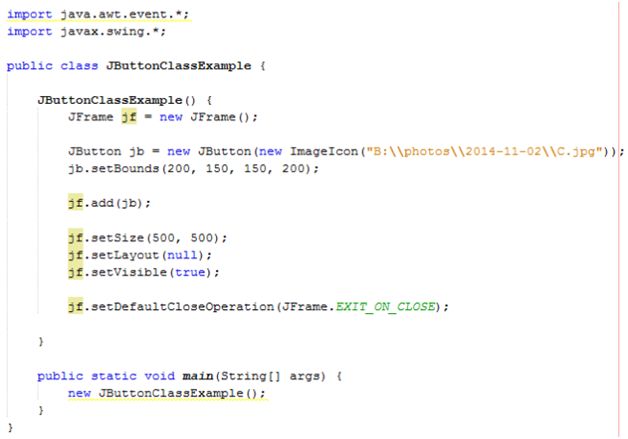
Output
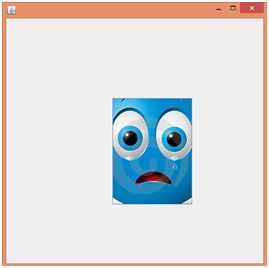
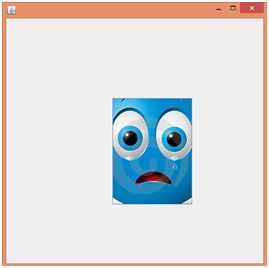
Summary
Thus, we learnt that JButton class creates a button, which has a platform-independent implementation. JButton class extends AbstractButton class and also learnt how we can use it in Java.